Object-oriented programming (OOP) seeks to emulate real objects through code. In life, objects have both characteristics, those attributes that define it, and behavior. OOP is a completely different approach to programming than procedural. As such, its actual implementation is often misapplied and misunderstood. In this hands-on lab, you will get an introduction to OOP. You’ll explore how to implement it for your WordPress plugins and theme.
In typical fashion here on Know the Code, this lab will be presented without all the confusing and scary technical jargon. Instead, you’ll be introduced to the concepts and how to apply them in your code. Then I’ll share those fancy terms, such as inheritance, encapsulation, and polymorphism. See, I told you they were scary words. But don’t worry, as I’ll break it down for you.
What Will You Learn?
In this hands-on lab, you and I are going to ease into programming. You’ll learn about:
- What is OOP?
- What is an object?
- What’s the difference between procedural and OOP?
- How OOP is used in WordPress
- The difference between Class Wrappers (i.e. a class that is only static properties and methods) and OOP
- The difference between a procedural function and a class’ method
- What the heck is
$this
? - OOP syntax
- How to create objects
- Declaring object attributes using properties
- Hiding away the details using visibility keywords (
public
,protected
, orprivate
) - Accessing the workers and attributes of an object using
->
or::
- Strategies for creating class structures
- Practical examples to help you transition into OOP
Prerequisites
In order to do this lab, you will need to have your local development machine ready to go. Check out this article in the Help Center for what is needed.
This lab assumes that you already know the basics of PHP. If you need to brush up on your PHP fundamentals, then head over to the PHP Library before doing this lab. I want to make sure you get the most out of it and are not struggling with the language basics.
You get WET when you swim. Stay DRY when you code.
Episodes
Total Lab Runtime: 03:04:55
- 1 Lab Introductionfree 08:27
- 2 What is Object-Oriented Programming (OOP)?free 14:14
- 3 What is an object?free 13:30
- 4 Meet the Class Blueprintpro 04:53
- 5 Creating an Objectpro 07:57
- 6 Defining Characteristicspro 10:54
- 7 Putting the Object to Workpro 13:56
- 8 Hiding Away the Complexitypro 22:40
- 9 Working within the Object with $thispro 15:41
- 10 Internal Control of Object Creationpro 15:07
- 11 Class Constantspro 08:58
- 12 Class Staticpro 17:49
- 13 What OOP is Notpro 19:11
- 14 Practical Examplespro 04:52
- 15 Wrap it Uppro 06:46
What to Do Next?
Other PHP Resources
A collection of other awesome educational resources.
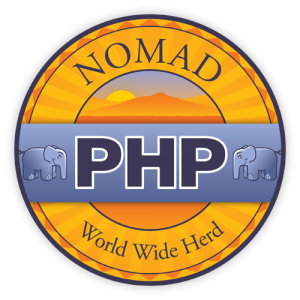
Object-Oriented Bootcamp in PHP – Laracasts
Jeffrey Way at Laracasts has an awesome Object-Oriented Bootcamp. If you get a chance, go check out that bootcamp.
Object-Oriented PHP – Codecademy
Codecademy has an interactive course that lets you write code as you learn. Check it out Learn more
The Ultimate Guide to Object-Oriented PHP for WordPress Developers
Josh Pollock wrote an awesome e-book that is available to you from WP Engine. It includes PHP fundametnals, PHP 7, WP_Query, Magic methods, and more. Check it out Learn more