Even though I use and like Genesis doesn’t mean there aren’t areas where it doesn’t fully comply with clean, quality coding techniques. In the last episode, I showed you the code for processing the post image. Did you notice that the code within the function genesis_do_post_image() will not run (none of it) if the conditional expression is not true? Ah, this is a perfect example of when to return early. Return early pattern: when you are done processing and nothing else in the function/method is going to be processed, then so bail out and return early. Why? Why would you […]
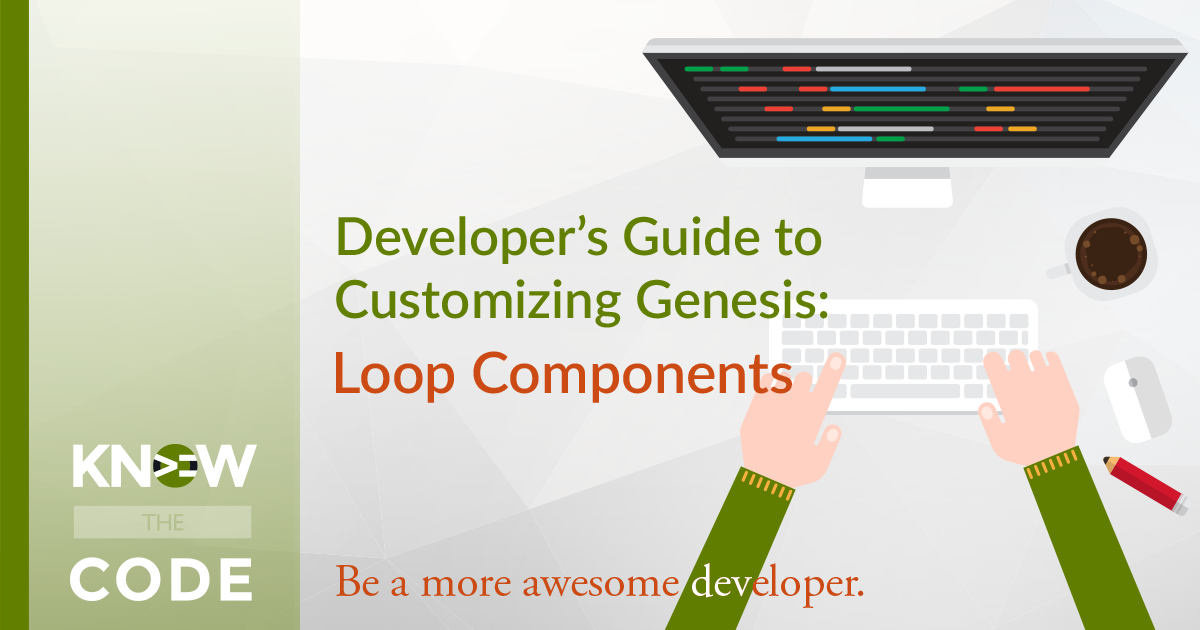