In the last lab, you and I built a custom meta box for the Subtitle custom fields, adding the feature of subtitles for posts. What happens if you need to add more than one meta box to a project? Using the code from the last lab, you would have to copy and paste the meta box file, change what’s different and each of the function names, and then create a new view file. In doing so, your code is redundant with repeating code patterns. Plus, it takes you time to make the codes, meaning an error could occur. What if […]
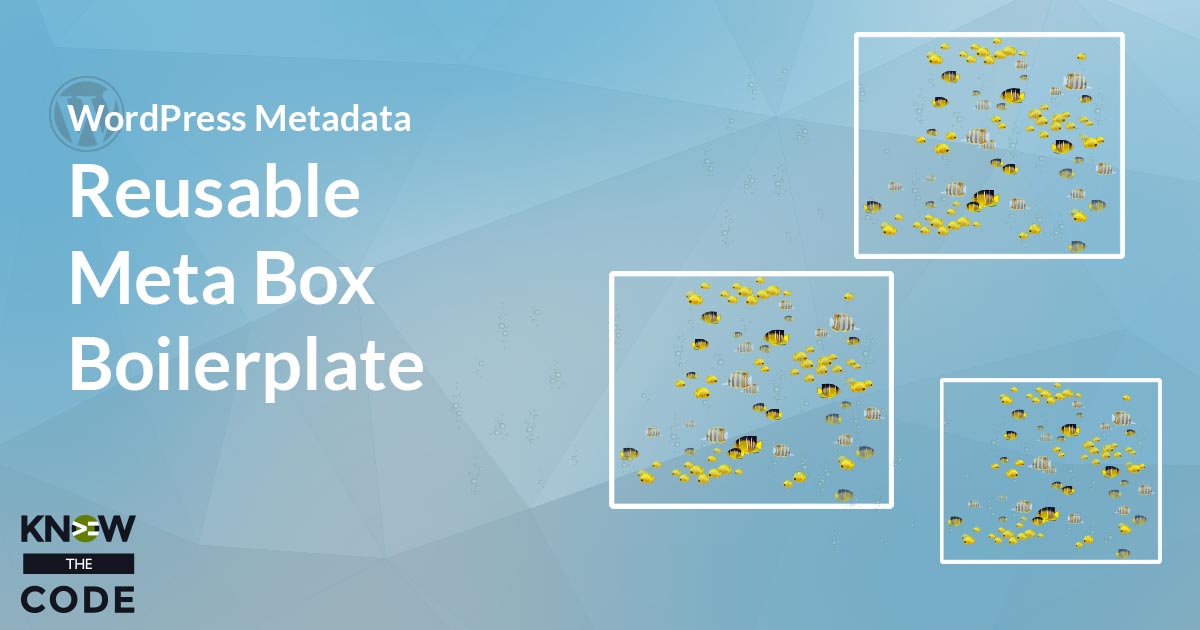