The repeater is a popular interface within a WordPress meta box. It allows your client to dynamically create new custom fields for a specific piece of content. In this hands-on lab, you’ll dive deep into JavaScript as you build your own repeater from scratch.
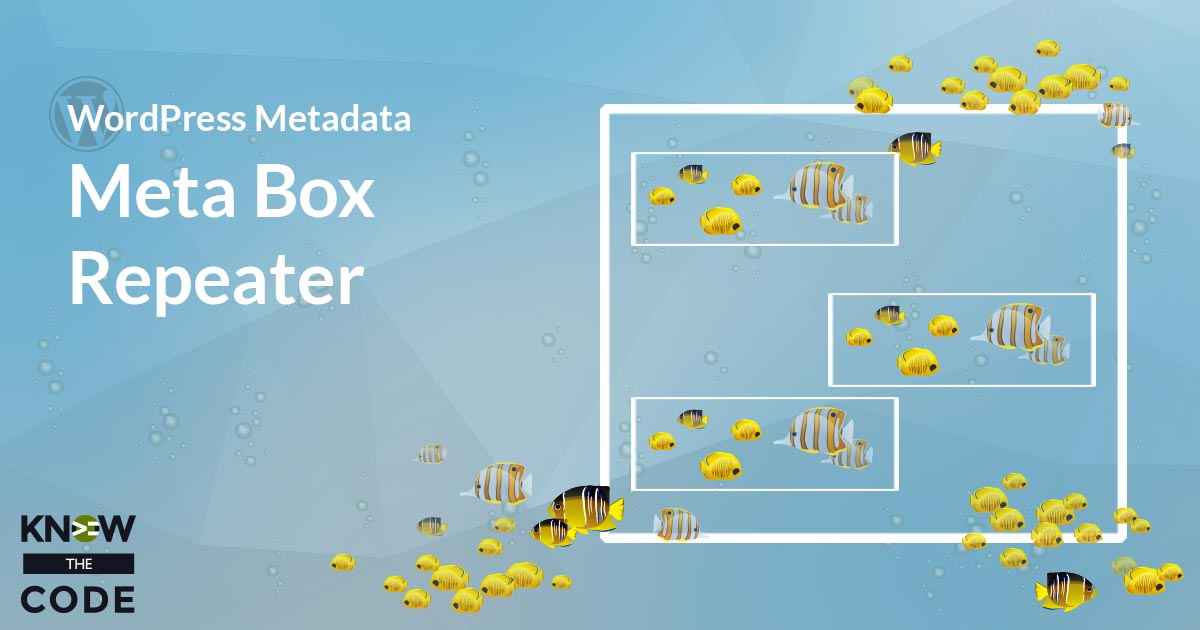