Let’s talk about OOP is not. When you look at different plugins in WordPress, you’ll see a common pattern of class blueprint with all static properties and methods. That is not OOP. Why? You can’t create an object from statics. Statics don’t know anything about objects, as they belong to the class. This episode will show you why this matters. The design approach of wrapping static functions and properties within a class is called Class Wrapper. It’s a design pattern that utilizes the class’ ability to hide complexity. When used for that purpose, it can add helper functionality to your […]
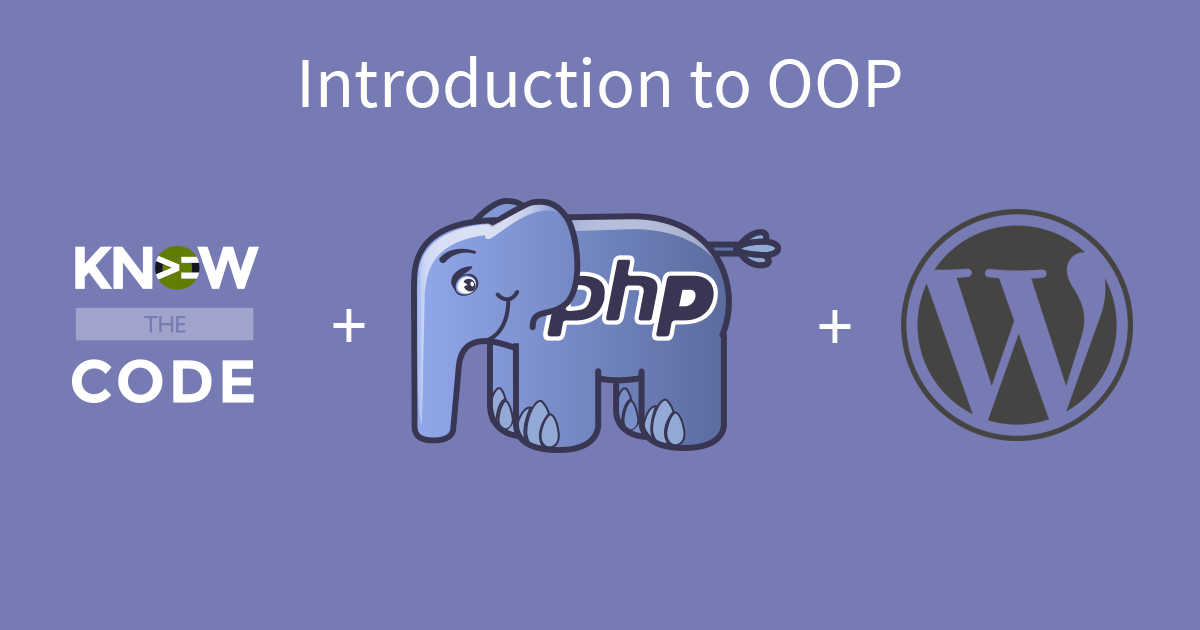