There will be times when you need to remove a callback from a 3rd party plugin or application. Your project may require a completely different implementation for part of the plugin. This edge case will happen in your career. Okay, then how do you remove an object’s method callback within a 3rd party plugin? Imagine that you have a project that is using WooCommerce and you need to build a completely different implementation of sending out an email when a new customer note occurs. How can you remove the built-in callback so that you can overwrite it with your code? […]
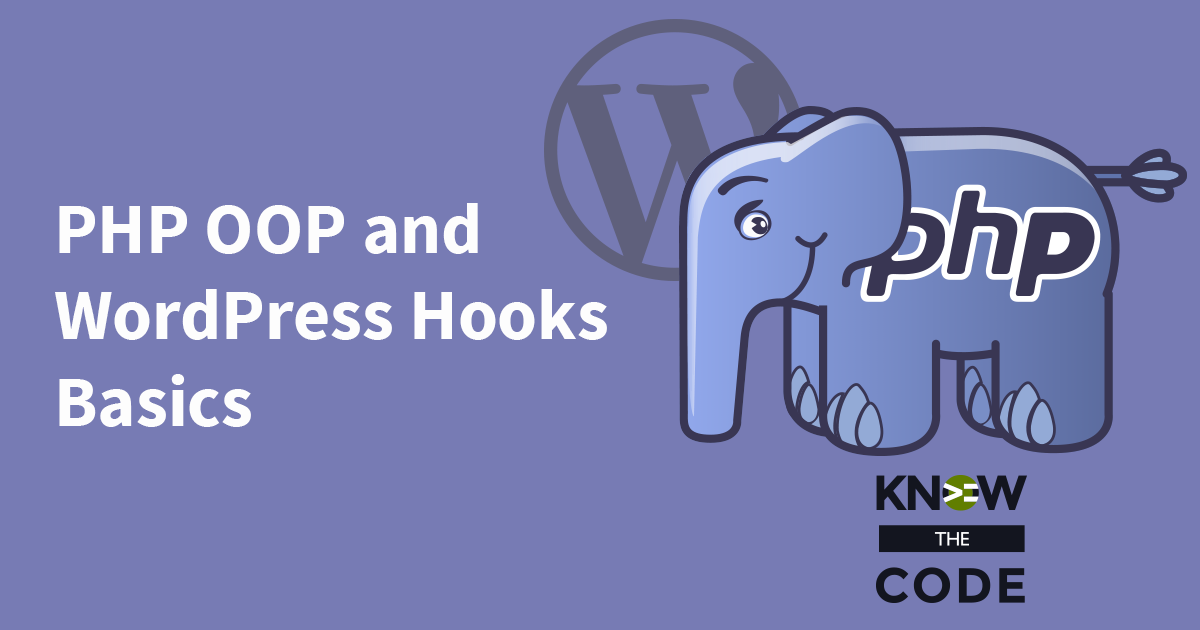