What’s the limit for inline comments, and how can you minimize them? Let’s look at a bad and a good example of inline comments, and how they’re chances to improve your code.
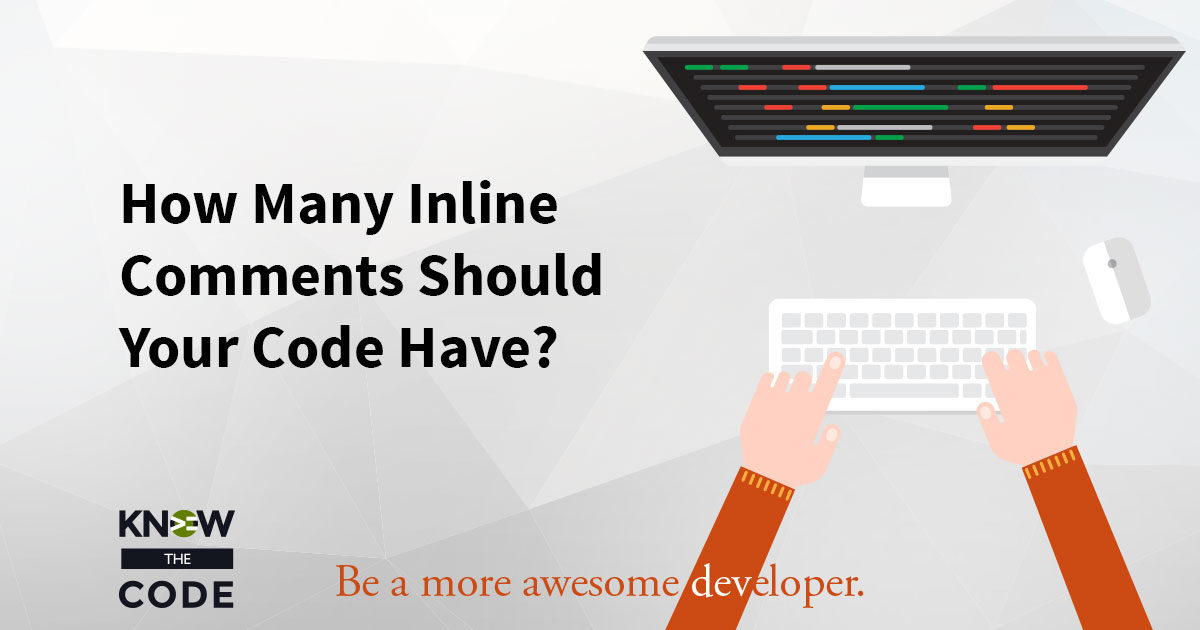
Inline comments aren’t a huge problem. They make code a little harder to read.
But more than 2-3 in a function usually point to a bigger problem.
They’re usually a sign that the code is hard to understand.
Poor Use Of Comments
For example, here’s a method that I wrote, with 4 lines of inline comments:
/**
* Gets the markup in Bootstrap format.
*
* @param array $args Widget arguments.
* @param array $instance The widget instance.
*/
public function widget( $args, $instance ) {
ob_start();
parent::widget( $args, $instance );
$output = ob_get_clean();
/**
* DOMDocument::loadHTML() raises an error in parsing some HTML5 elements, like <aside> and <section>.
*
* Themes can add HTML5 elements via $args['before_widget'] and $args['before_widget'].
* So this removes them from the markup that DOMDocument::loadHTML() parses.
* The 'before_widget' and 'after_widget' markup will still be part of the final widget markup in the echo statement.
*/
$markup_to_search = str_replace(
array( $args['before_widget'], $args['after_widget'] ),
'',
$output
);
$plugin = Plugin::get_instance();
$list_group = $plugin::$components::$widget_output::reformat_dom_document( $markup_to_search );
echo preg_replace( '/<ul[\s\S]*<\/ul/', $list_group, $output ); // WPCS: XSS ok.
}
It’s not impossible to read. But it points to a need to improve the code.
I could have abstracted lines 19-26 into a separate method. Or moved the comments in lines 12-18 into the PHP DocBlock.
Good Use Of Comments
This method doesn’t have any inline comment:
/**
* Save the new form settings.
*
* This saves the values of the new settings that this class adds.
* Including the settings to display horizontally, and at the bottom of the post.
* rgpost() is a Gravity Forms helper function.
*
* @param array $form The form that is shown.
* @return array $form With additional settings, or null if $form is not an array.
*/
public function save_settings( $form ) {
if ( ! is_array( $form ) ) {
return $form;
}
$form[ $self::bottom_of_post ] = rgpost( $self::bottom_of_post );
$form[ self::horizontal_display ] = rgpost( $self::horizontal_display );
return $form;
}
It could have had a comment above rgpost()
on line 15, but it’s in the the PHP DocBlock on line 6.
I think this makes it easier to read.
Inline Comments In Code
- They’re not a big problem in themselves
- But more than 2-3 in a function usually point to a need to improve the code
- To remove the need for them, you could abstract code into a function, use descriptive variable names, or add more comments to the DocBlock above the function
Did you learn something new? Share your thoughts.