PHP gives another way to build dynamic strings besides embedding variables and concatenation. You can build a formatted string with placeholders and then use either sprintf or printf to build the string. Resources sprintf – in Docx sprintf – PHP Manual printf – PHP Manual Type Casting
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
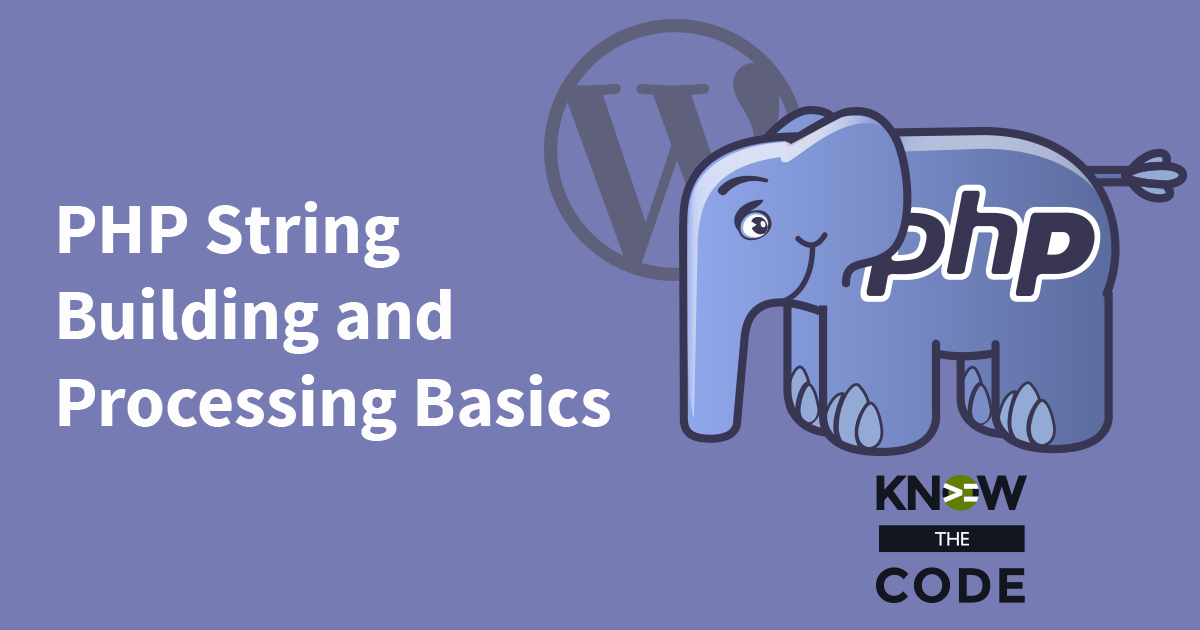
Formatting a String using Placeholders
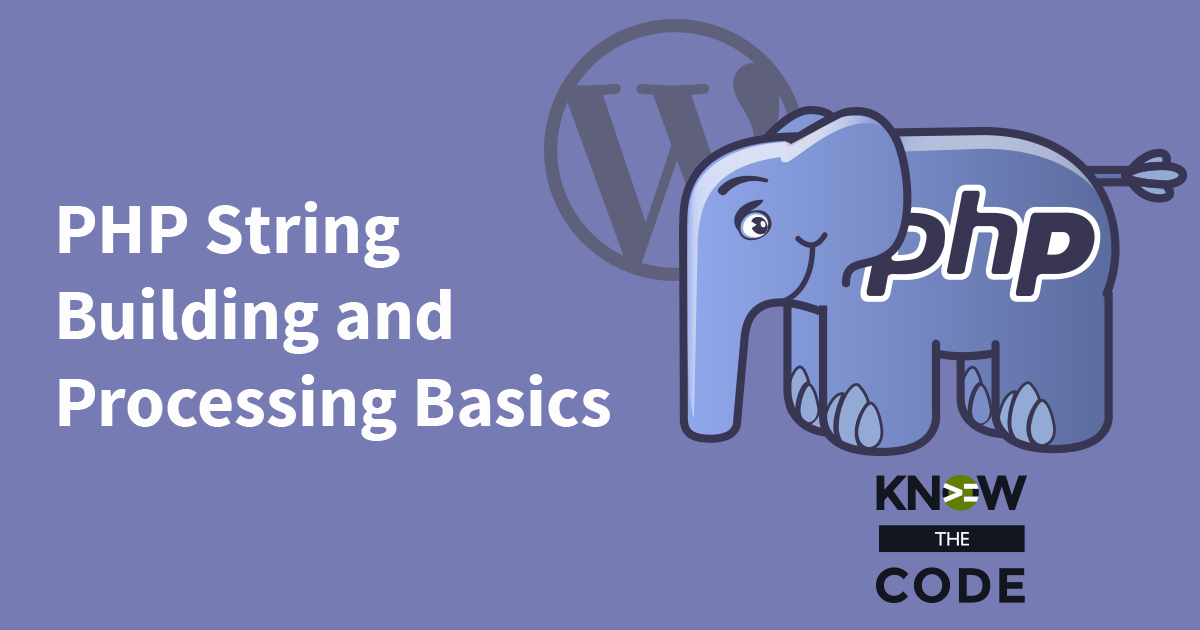
Concatenating and Assigning Shorthand
Often we need to build strings incrementally. A good example is with building an output HTML string pattern or even a message. You build part of it, do some processing, and then keep appending to the same string and assigning it back to the variable. PHP gives you a shortcode operator for this process that turns: $message = $message . ‘Welcome ‘ . $first_name; into: $message .= ‘Welcome ‘ . $first_name;. Both of these lines of code are processed the same way by the PHP interpreter. First, the strings are concatenated. Then the assignment is made to the variable $message.
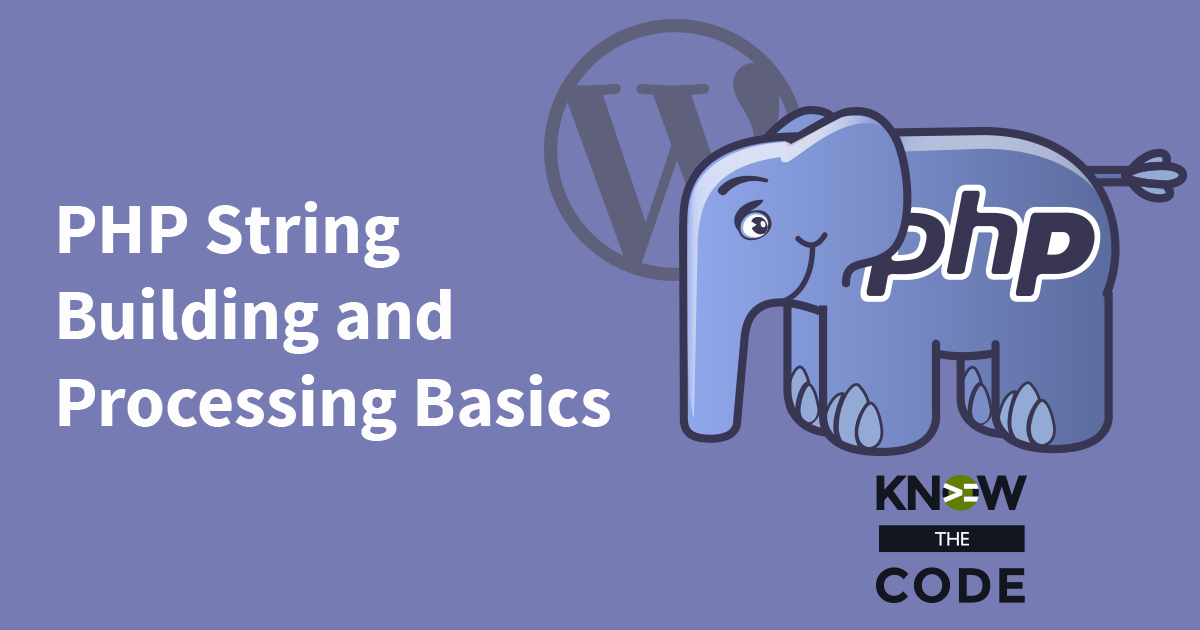
Concatenating Strings with a Dot
PHP gives multiple ways to build strings. You previously learned about embedding variables into the string. In this episode, you will learn about the concatenating operator, which is a dot .. PHP takes the left string and smooshes it together with the string on the right side of the dot. Think of it as smooshing the two strings together. This method gives you the ability to break the code out of the string literal. Then you get the ability to call functions too. When putting together multiple string parts in one line of code, I want you to stop and […]
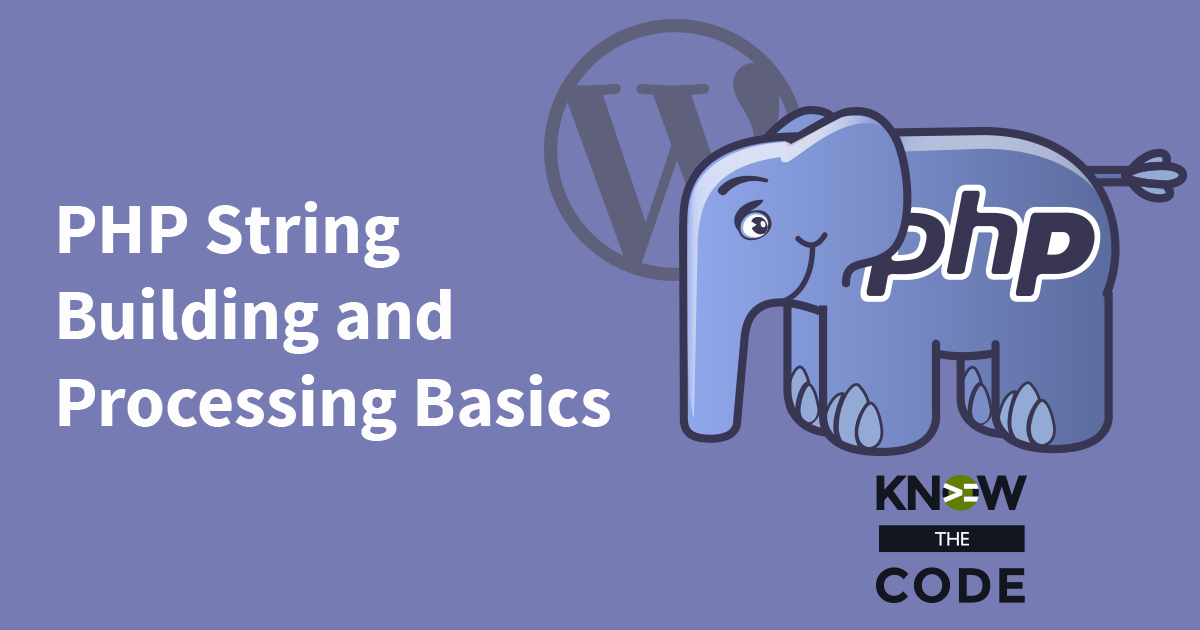
Embedding Variables in a String
PHP provides the means for us to build dynamic strings, i.e. strings that have varying strings within them. One method is to embed a variable directly into the string. When PHP comes to a single quote, it handles the string within the quotes as a string literal. There is no processing that happens. Rather, it treats it as a hard-coded string. Therefore, if you wrote echo ‘My name is {$first_name}.’, the entire string would be rendered out to the browser just as you wrote it. The variable would not be processed.
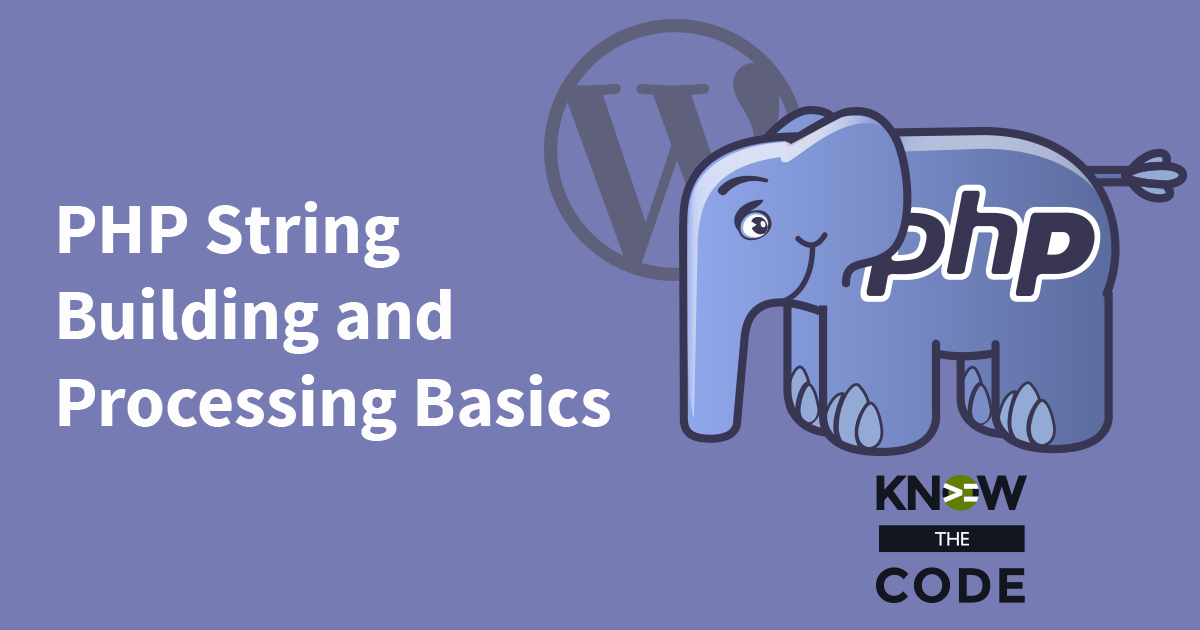
Lab Introduction
In this lab, you and I are going to work with strings. You’ll learn about the why, when, what, and how of string building and processing with PHP. You’ll learn about embedding variables to make a string dynamic, concatenating strings, shorthand versions, formatting strings, counting the length, checking if a string has a substring in it, and more. To get started, let’s spin up a new test website. I’ll walk you through the process using: DesktopServer PhpStorm UpDevTools Kint PHP Debugger plugin Getting a Kint Error? If you are getting a d() or ddd() function not found fatal error with […]
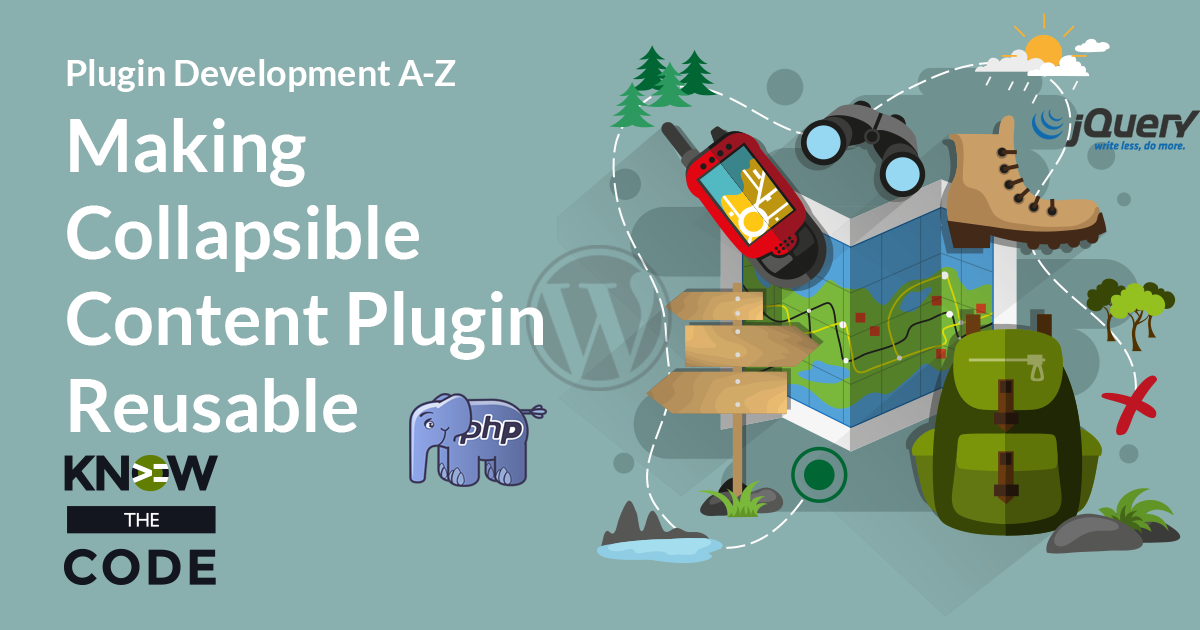
Move Config to FAQ Module
Let’s stop and talk about our configuration architecture. Does it make sense to have the configuration files in the plugin’s config folder? Or should those be with the FAQ Module? Hmmm, let’s talk our way through both strategies.
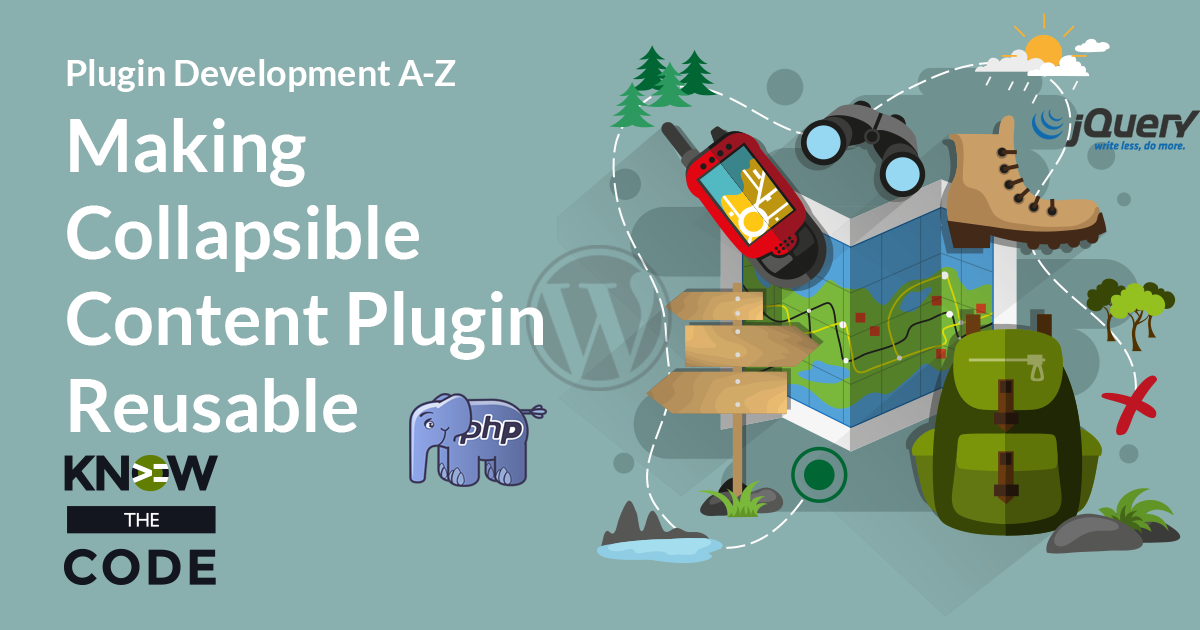
Refactoring the Label Generator
Whew, now it’s time to refactor the label generator function. Our labels are split apart into the three categories. What do we need to do? First, we need to know if we are generating labels for a taxonomy or post type. Then we can merge those together with the shared labels.
mb_strtolower
The What It’s available in PHP 4, 5, and 7. Syntax string mb_strtolower( string $string_to_convert, string $character_encoding = mb_internal_encoding() ); PHP Manual Description This construct converts a string’s alphabetic characters to lowercase. It uses the current locale to determine the converter. Unlike the strtolower(), you can specify the character encoding, such as “UTF-8.” Why? This function ensures characters such as umlaut-A (Ä) are properly converted. Parameters string_to_convert This is the string to be converted into lowercase characters. character_encoding (optional) You can specify the character encoding to ensure that expanded character sets, such as umlaut-A (Ä) are properly converted. Return Values […]
strtolower
The What It’s available in PHP 4, 5, and 7. Syntax string strtolower( string $string_to_convert ); PHP Manual Description This construct converts a string’s alphabetic characters to lowercase. It uses the current locale to determine the converter. This function uses the Unicode character set for the character encoding. Note: If you need to convert expanded character sets, such as umlaut-A (Ä), then use mb_strtolower() instead, as you can specify the character encoding, such as “UTF-8.” Parameters string_to_convert This is the string to be converted into lowercase characters. Return Values This function returns the converted string. More coming soon…keep checking back. […]