One of our jobs is to build quality code that is reusable again and again without changing the codebase. Ideally, we’d like to configure what’s different and have the codebase the same for all of our projects. In Part 3, you will convert the Collapsible Content plugin into the ModularConfiguration design pattern by moving all runtime configurable parameters into configuration files Then the codebase will be made reusable. The actual implementation then is determined by the configuration file that’s loaded. This approach will save you time.
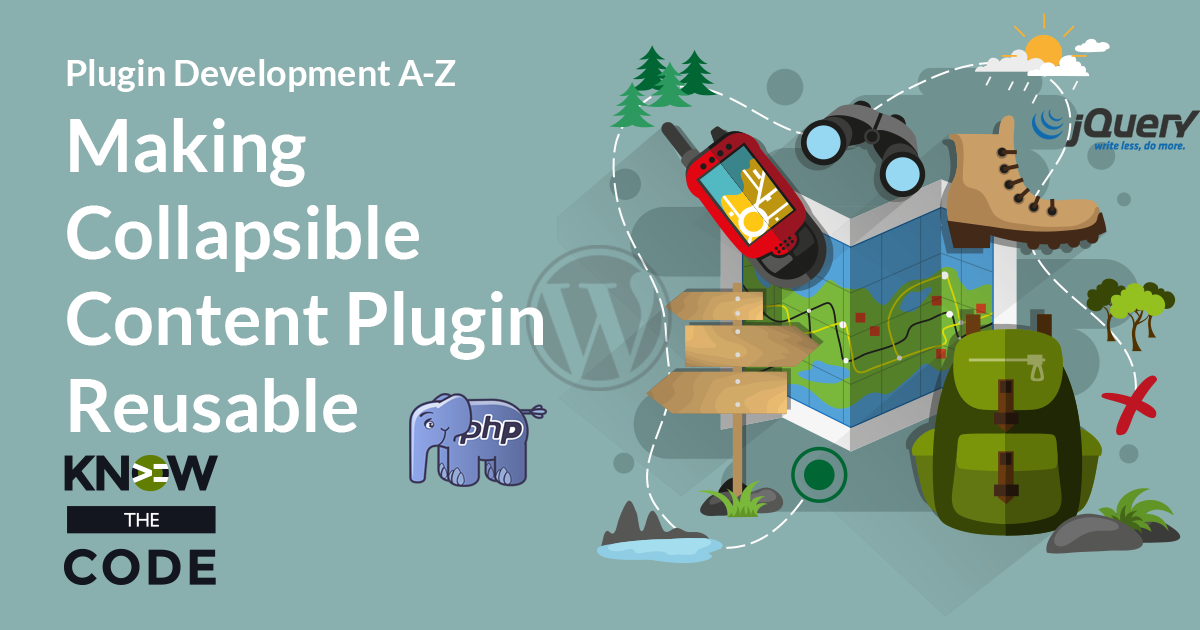