In past episodes, we talked about caching a collection of elements to avoid multiple returns to the DOM to fetch the elements. Let’s talk about it now in the context of the script you are writing. You’ll see an additional “why” of caching.
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
jQuery’s $
The variable $ does not have context and does not natively mean jQuery. It can also stand for Mootools and any value. You need to specifically initialize jQuery to the $ variable. Let’s talk about it in relationship to the IIFE. I’ll show you how to pass jQuery into the IIFE (yes, you can pass in objects) and then assign it to the variable for use within the IIFE’s scope.
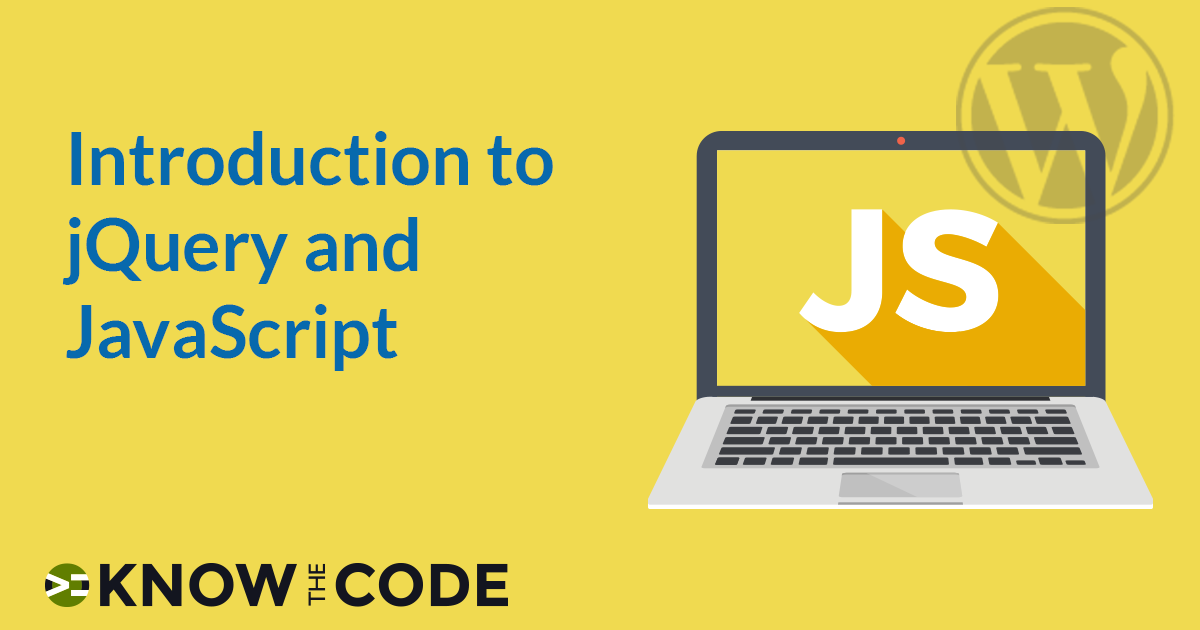
Basic Events
JavaScript provides plenty of event handlers. jQuery provides you with the sugar to work with key presses, mouse clicks, bindings, and more. Let’s take a look at clicks. You’ll learn about and write code for the click event using both .on() and .click().
Data Types
Let’s do a quick review of data types in JavaScript. We’ll talk about primitive types (string, number, boolean, undefined, and null) as well as objects and arrays. You will learn: JavaScript is a loosely typed (dynamic) language, meaning the data type is assigned by its value Changing data types Checking what the data type is with typeof Arrays and Objects For a more in-depth lesson on data types, make sure you sign up for JavaScript for WordPress and/or MDN JavaScript data types and data structures.
Variables
With all programming languages, you need a way to store a value in a variable, in order to work with that value. JavaScript provides variables for you. Let’s talk about how to declare and use variables. You will use the var variable statement to declare variables. You get to play around with changing (manipulating) the DOM to set different CSS styles to elements. You’ll learn about: The difference between “declaring” and “initializing” variables Browser’s console retains code in memory – until you refresh Why does the console show “undefined?” Optimizing by assigning a DOM lookup to a variable What JavaScript […]
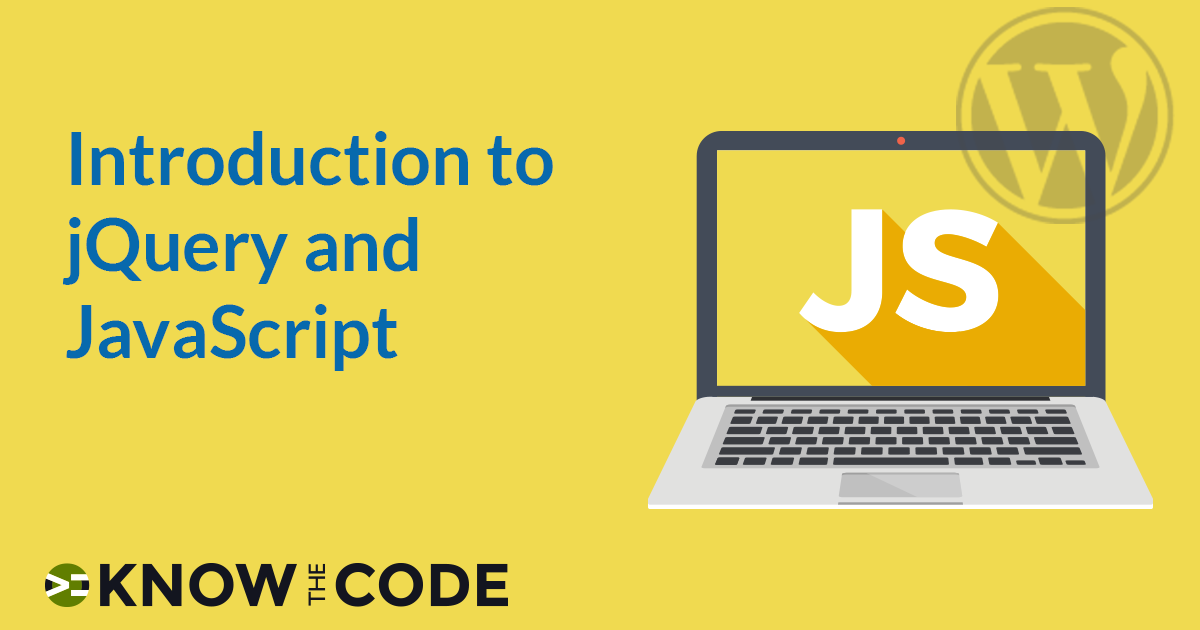
Basic Animation Effects
I bet you’d like to animate an element to increase the front-end interactive. In this episode, you will work with various jQuery effect commands including: hide() show() slideUp() slideDown() You’ll see how to change the duration, which changes the speed of the effect.
Traverse the DOM
In this episode, you will learn how to traverse up and down the DOM tree with jQuery. In a previous episode, we talked about how JavaScript traverses through the DOM tree, node-by-node to fetch elements and manipulate them. How can you move up and down from a given node? Let’s look at the jQuery traversing commands: next() prev() closest() children()
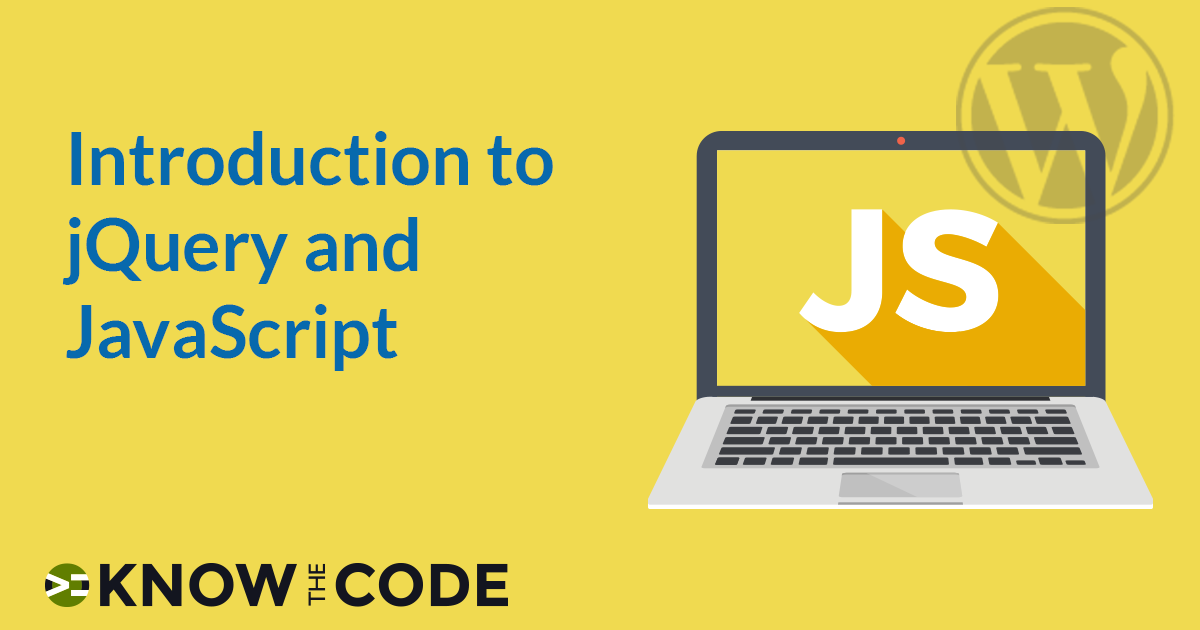
The Why of jQuery
Why would you want to use a library like jQuery? Why? It’s all about rapid web development. Libraries and frameworks allow you to write less code, which reduces your development time and costs. Let’s talk about the why of libraries like jQuery.
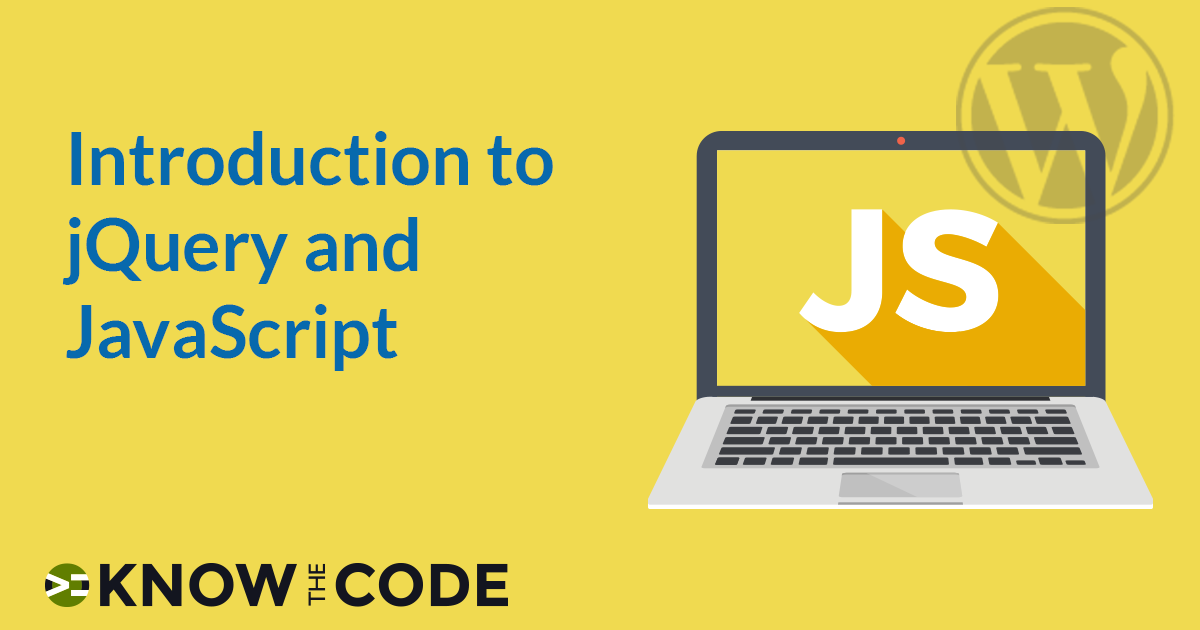
Select Elements from the DOM
In this episode, you will learn how to select elements (nodes) out of the DOM using both JavaScript and jQuery. You’ll learn what is returned, how to select by HTML tag, and how to select by an attribute, such as id or class. We’ll start with a discussion on the $ representation for jQuery.
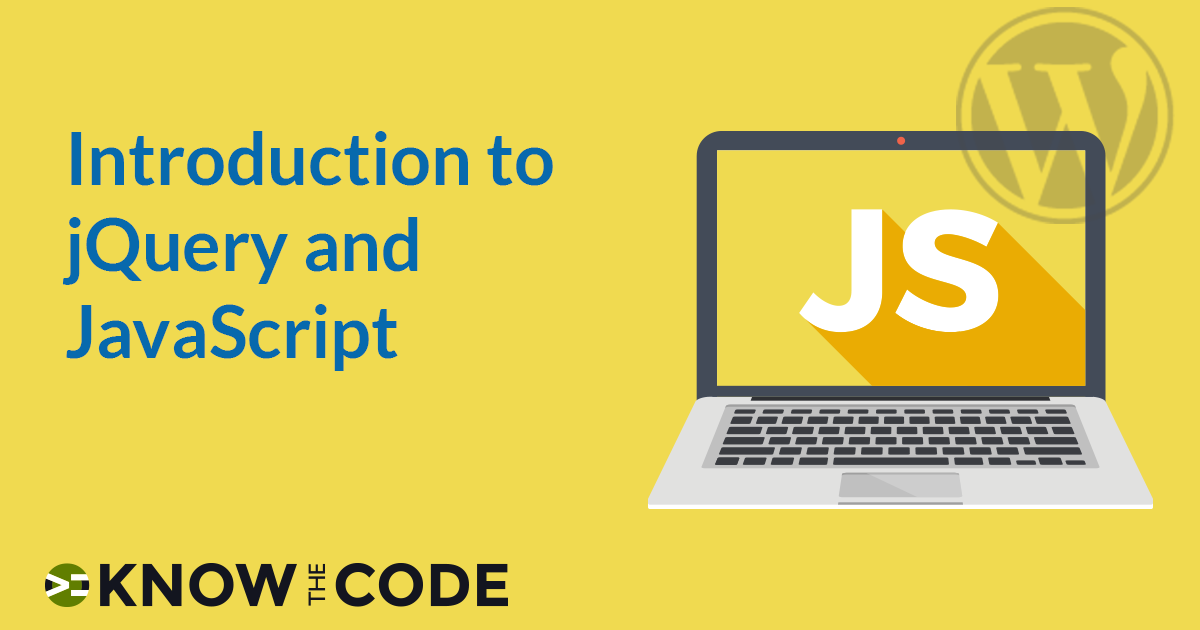
Document Object Model (DOM)
What is the DOM? In JavaScript and its libraries and frameworks, including jQuery, it works with the DOM. Okay, what is it? This episode presents it to you. Further Reading: What is the DOM – on MDN W3C – Introduction to the DOM