What if you want to remove the icon and replace it with a “…”? How would you do that? Think about it. Think about the code from the last episode. You have the default text coming into your callback. That means you can concatenate that string and append the dots after the incoming text.
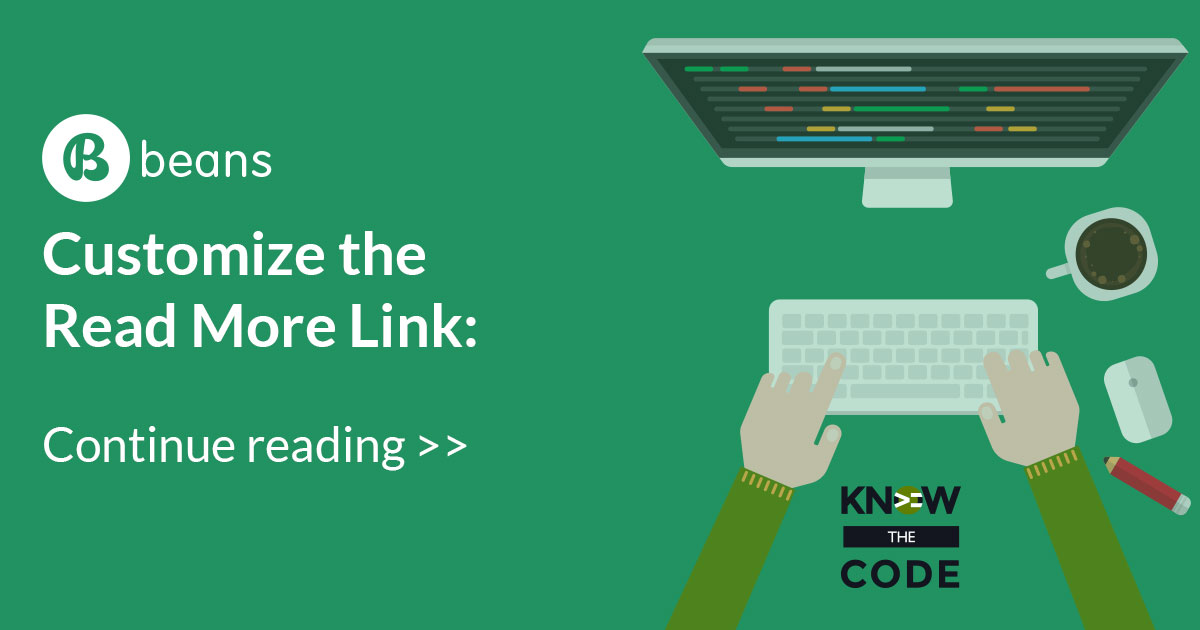