You need to load files. Right? In PHP you use one of these constructs to load a file into memory (which then makes it run): include( ‘path/to/file’ ) include_once( ‘path/to/file’ ) require( ‘path/to/file’ ) require_once( ‘path/to/file’ ) Let’s look at how these work as well as talk about when you want to use one over the other. You’ll try to load a file that doesn’t exist and see that include gives you a warning while require causes the site to stop with a fatal error.
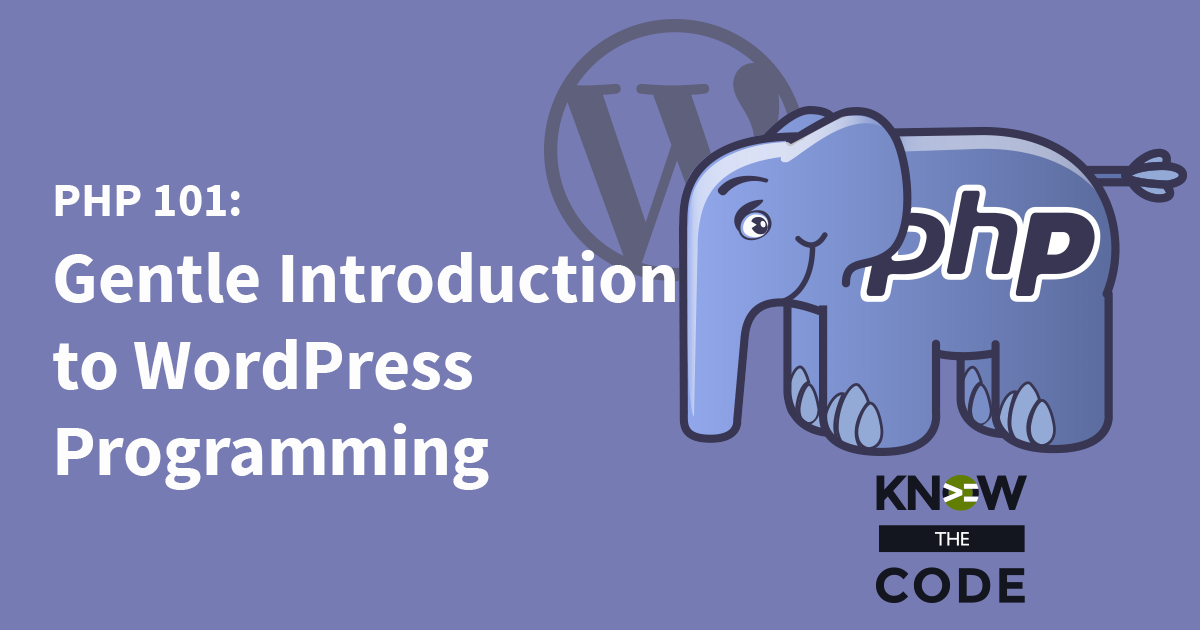