Let’s talk about what is the intent of variables, syntax, and naming standards. What is a variable? A variable is a symbol that represents data. It represents some value. It’s variable because you can change its value by reassigning it another value. For example, if you had a variable $post_id = 10;, you can change the value of the variable by doing $post_id = 20;. It’s the same variable, but you varied the value by assigning it a new value. Why is it a “symbol?” A symbol lets us define an alphanumeric symbol that we can read. It “represents” something […]
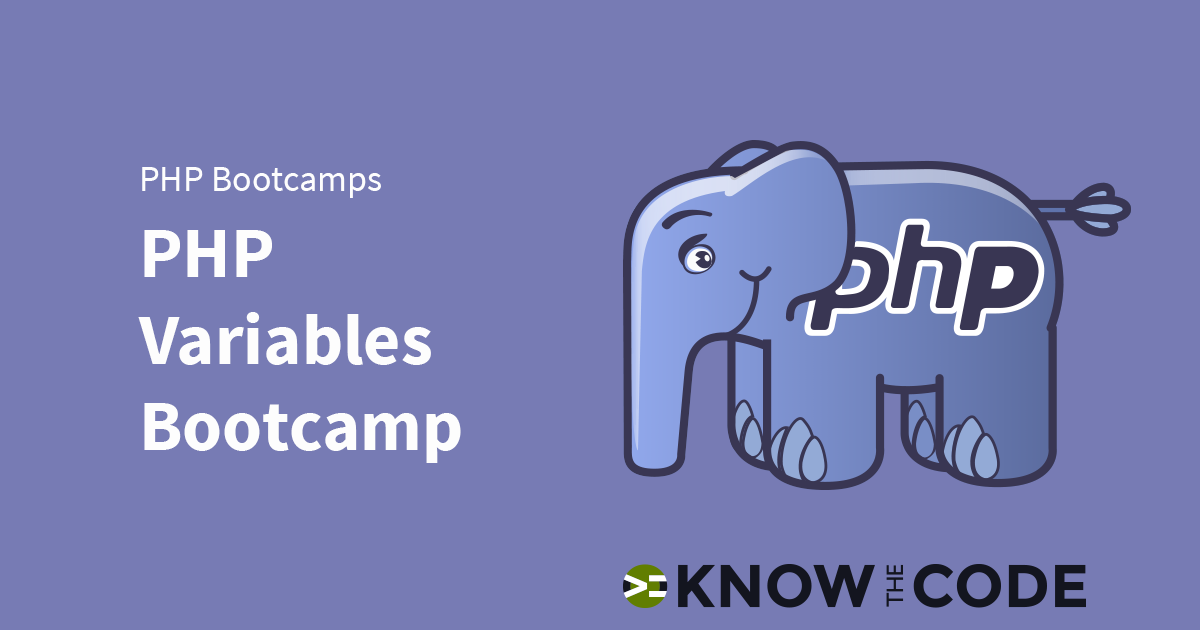