Let’s see how namespacing affects variables.
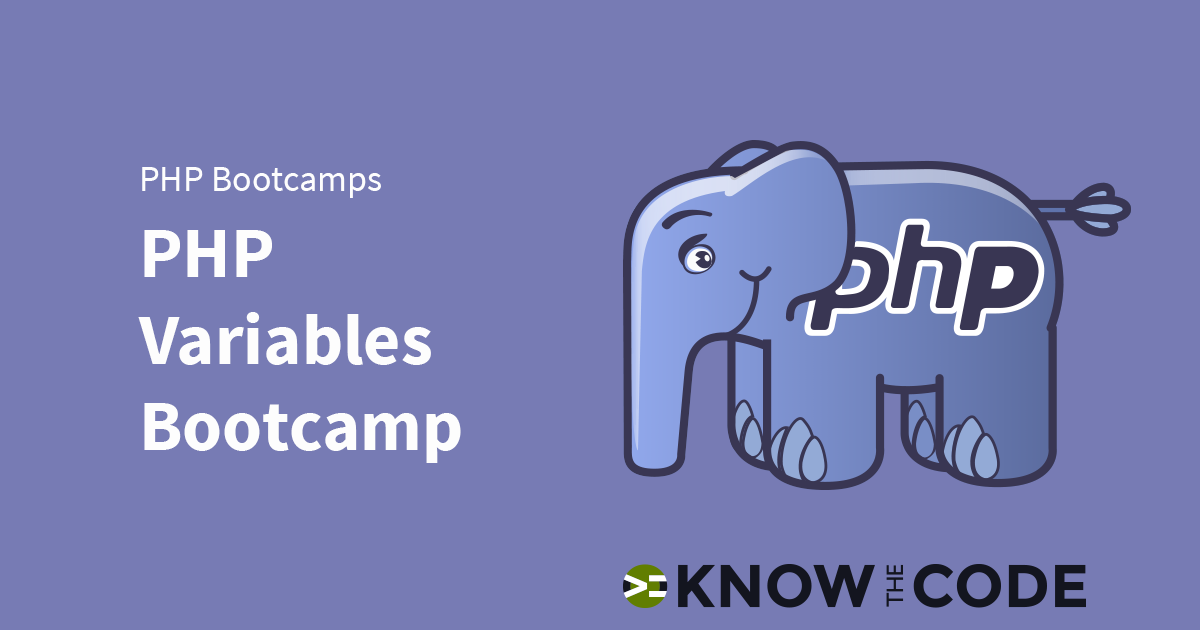
Developing & Empowering WordPress Developers
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
0 Videos Runtime
Let’s see how namespacing affects variables.
0 Videos Runtime
Now you’ve seen passing by reference in action. Let’s dive into how PHP manages it. You’re going into the PHP interpreter and its C language code.
0 Videos Runtime
Let’s dive into passing data by reference. By reference allows you to work with the same data between different variables. This means you can change the data and it is changed in both variables. You will build some code to test this concept and see how it works. Then you and I will talk about when to use it.
0 Videos Runtime
Let’s see how static variables retain their data within PHP internals.
0 Videos Runtime
Static variables retain their data even after the function gets done running. Then the next time you call it, it remembers the data it had from before because it is retained and persistent for the life of the web page request. Statics offer you an option instead of using global variables. Let’s take a look at how you can pass memory around in procedural coding without using globals. In this video, you will write code to show how the static and non-static variable work. You’ll build a for loop and then call two separate functions.
0 Videos Runtime
Let’s look at how PHP manages global variables and provides the scope. You’ll look again at memory.
0 Videos Runtime
The intent of global variables is to allow all of the code, all plugins and themes, to be able to both read and write to the variable. You want anything within the website’s PHP code to have full, unprotected access to the data that your variable represents. That means that any code within the website can change the data. For example, $wp_query is a global variable. Therefore, any piece of code in WordPress Core, plugins, and theme can both read and change the data.
0 Videos Runtime
To better understand variables and scope, it helps to look at the PHP internals and how variables are managed in memory. No, this is not a C course. Rather, you will see the concept of how PHP tracks and manages the variables and the data they represent.
0 Videos Runtime
The variable(s) declared within the parentheses of the function itself is called a parameter. You can have both optional and required parameters. Let’s talk about function parameters, why is it called a parameter, its scope, its intent, and how arguments are passed to each parameter.
0 Videos Runtime
Let’s talk about variable scope. Scope defines how and where you can access and use variables. It relates to memory. A variable within a function belongs to that function. Scoping is like a gatekeeper. It protects our code from other functions and code, to ensure we have control. It protects our variable naming to avoid naming conflicts.
Know the Code flies on WP Engine. Check out the managed hosting solutions from WP Engine.
WordPress® and its related trademarks are registered trademarks of the WordPress Foundation. The Genesis framework and its related trademarks are registered trademarks of StudioPress.
This website is not affiliated with or sponsored by Automattic, Inc., the WordPress Foundation, or the WordPress® Open Source Project.