Character set encoding matters as many language characters are not part of the standard ASCII character set. Instructions such as strlen will not behave the way you think when using it with extended characters such as €, ©, ®, à, ë, and many others. And languages such as Mandarin and Russian require a different approach when working with strings. In this episode, let’s talk about why the encoding matters and how the character set is really represented in the computer. Hint: Everything in the computer breaks down into binary, i.e. ones and zeros. Simple string instructions like strlen use bytes. […]
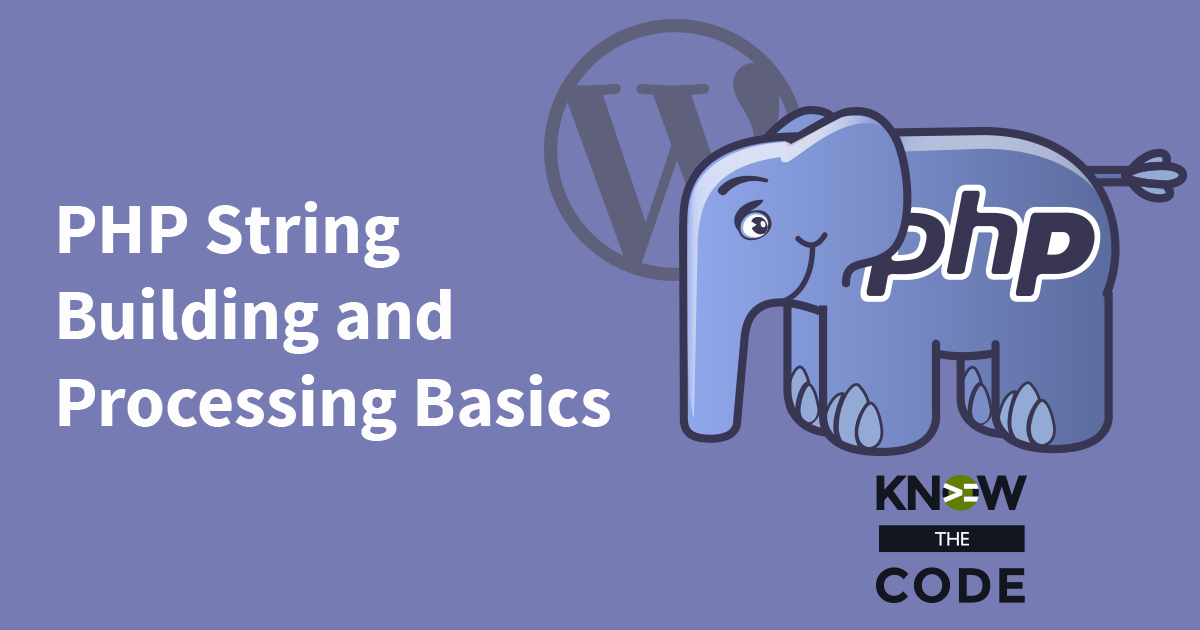