In this episode, we’ll take on the challenge of how to test a string to see if it starts with “http://”. You’ll discover there are multiple ways to do this check. Then we’ll create a utility function called starts_with and put that into our new Site Utilities plugin. Regex is not needed here. In this episode, I show you two different approaches to checking if a string begins with a substring: Check its position using strpos Check the beginning of the string to a specific length using mb_substr and mb_strlen
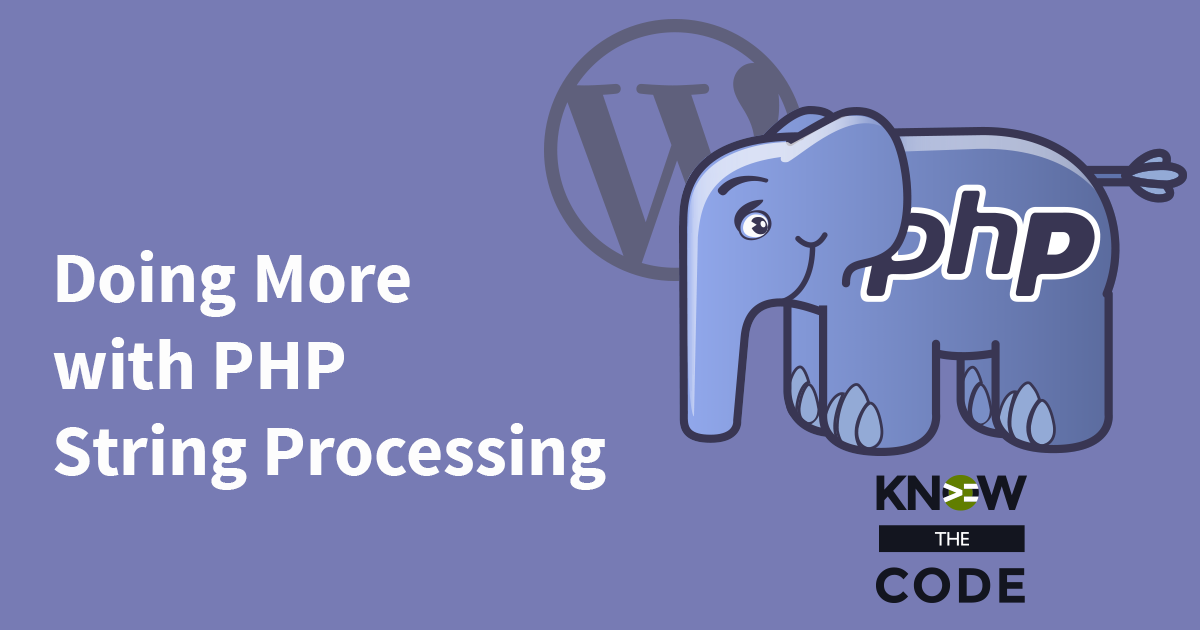