As you build your PHP expertise, you will likely grow into other frameworks and applications outside of WordPress. Laravel and Symfony are two awesome frameworks. Many times, you can pull in the modules and packages from their libraries even into a WordPress application. Yes, that’s how well these frameworks are built. Let’s take a quick look at the namespacing in each of these frameworks to show you how it’s used in the wild. You can also take a look at code construction and architecture too, i.e. to further your software building skills.
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
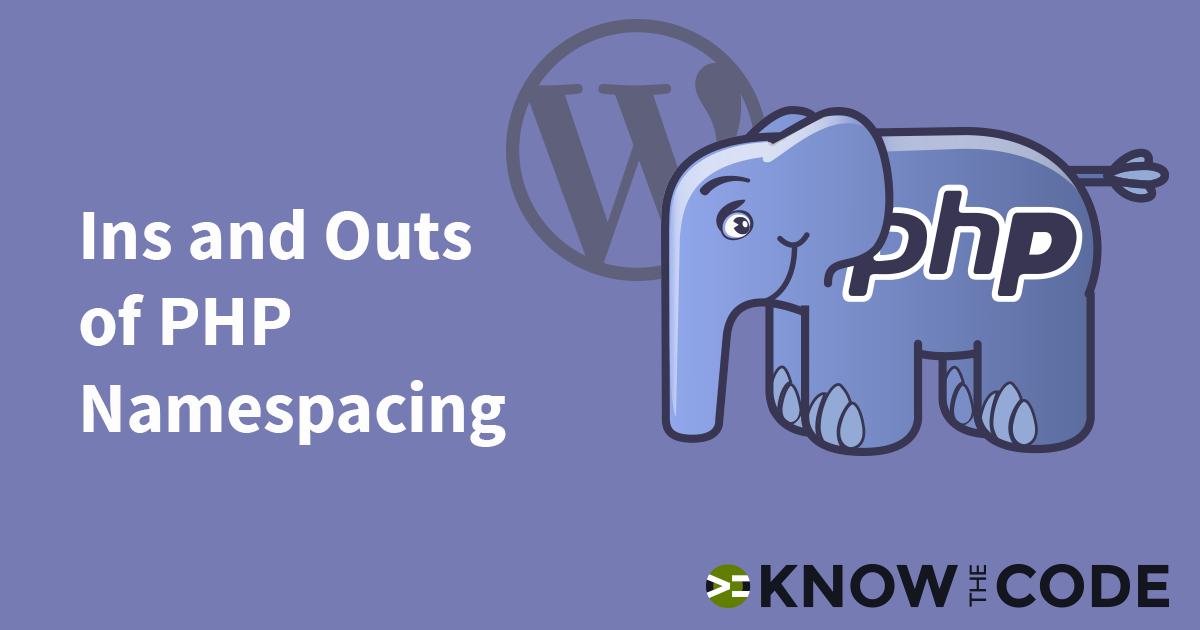
Fully Qualified Naming
To help you master the concept of “fully qualified naming,” let’s take a look at what each function’s name is. You will use the magic constant __FUNCTION__. It returns the fully qualified name of the function, which includes the namespace if one exists.
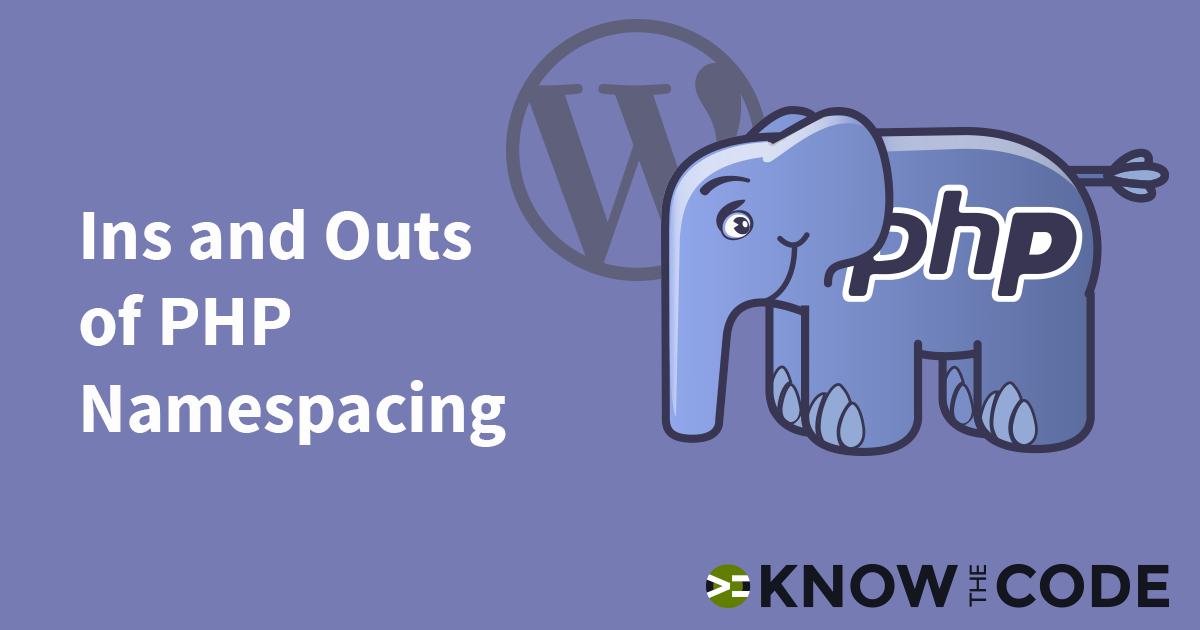
Using Functions from Another Namespace
You can use functions from different namespaces. But you have to be aware of how to bring in that function. Remember, the function has a fully qualified name which includes its namespace. When using that function in another namespace, you have choices: You can specify its fully qualified name such as \KnowTheCode\InsOutsPHPNamespacing\Sandbox\get_post_id() If the namespace is the root, then you can use a relative name such as Sandbox\get_post_id() You can alias the namespace and then use that alias in place of the fully qualified namespace If you are using PHP 5.6 and up, then you can import the function. You […]
The Basics
The best to learn about namespace and to wrap your mind around how it’s different than prefix is to use it and see it in action. In this episode, you will add a function called get_the_ID() to your plugin. Wait a minute. That function exists in WordPress Core. Aha, you’ll see what happens when you use a namespace around the plugin’s function. You’ll see how to call WordPress Core’s function versus the one in your plugin.
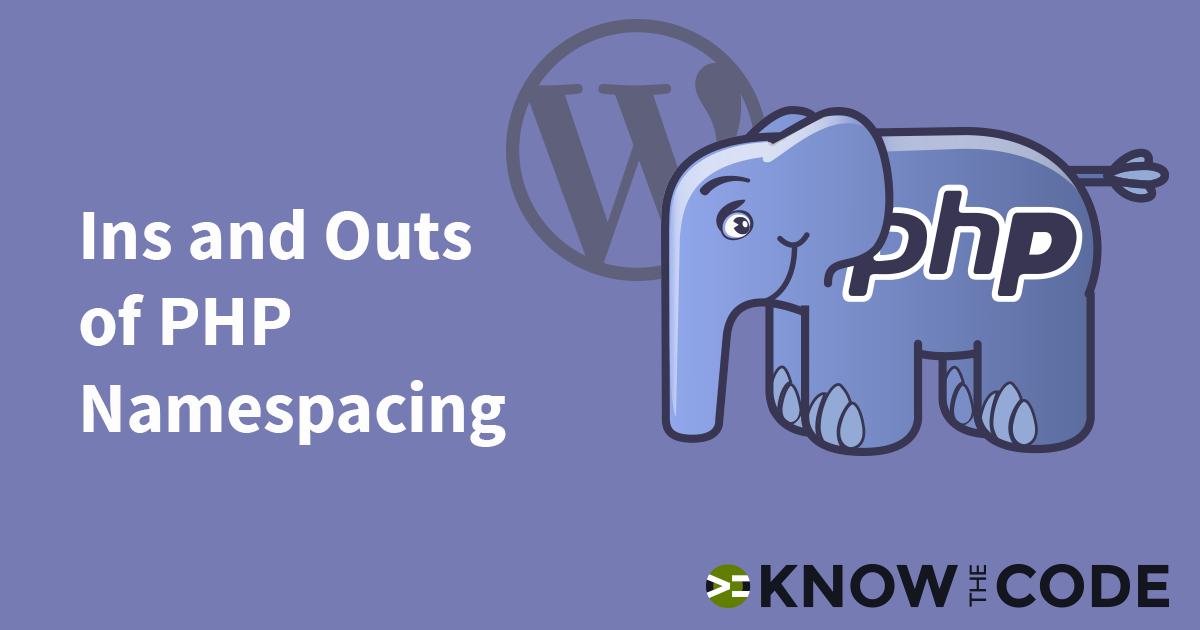
What and Why of Namespacing
What is PHP namespace? Why should you care about using it versus prefixing? What does it give you? What are the advantages? Let’s talk about it. The first thing to know is: the web server must be running at least PHP 5.3.0. This is an old version of PHP. It stopped being supported as of August 2014. It’s old. Shoot, 5.4 and 5.5 are old too and no longer supported. Check out the supported versions here on the PHP manual website. PHP namespace encapsulates your code. What does that mean? It means you are putting a container around your code. […]
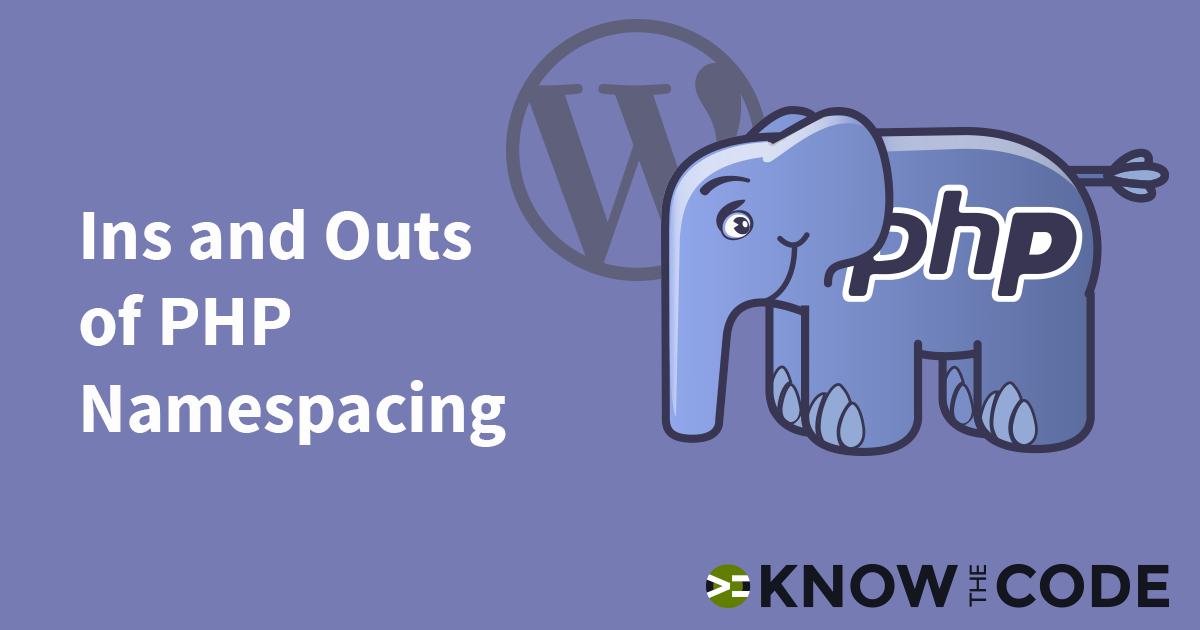
Lab Introduction
In this episode, you’ll learn what you will be doing in this lab. We’ll also make sure that your sandbox is ready to go. In order to do this lab, you will need a local sandbox site spun up and ready to go. You will need to install the lab’s starter plugin from GitHub, as you will be u
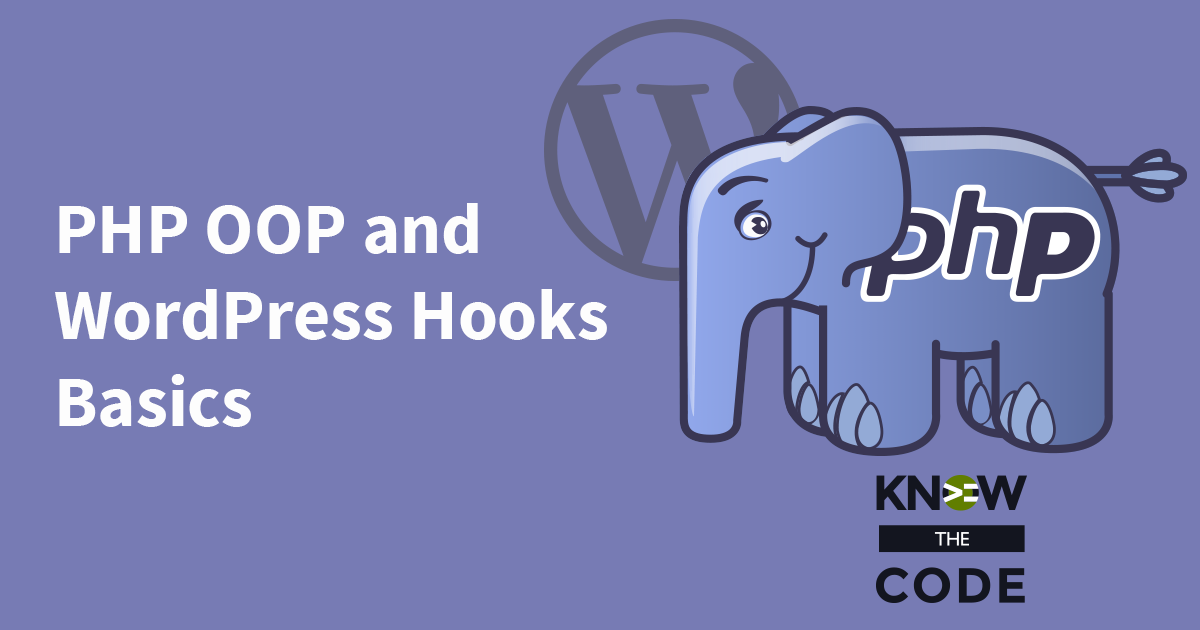
Meet the PHP Callback
Functions and methods can be dynamically called in PHP as a callback. In this episode, let’s talk about callables and callbacks, to get you prepared for how WordPress events actually work.
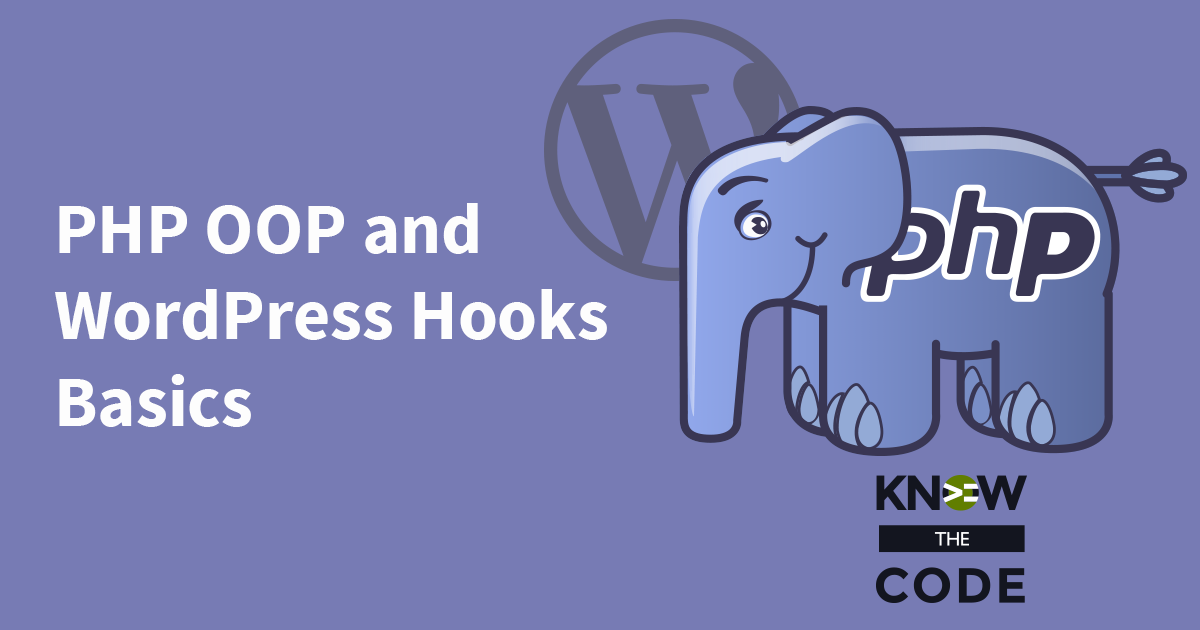
PHP OOP and WordPress Hooks Basics
Registering and unregistering WordPress hook callbacks is different with PHP objects. In this hands-on lab, you’ll learn the syntax, why it’s different, how to register and unregister, as well as strategies to work with other plugins.
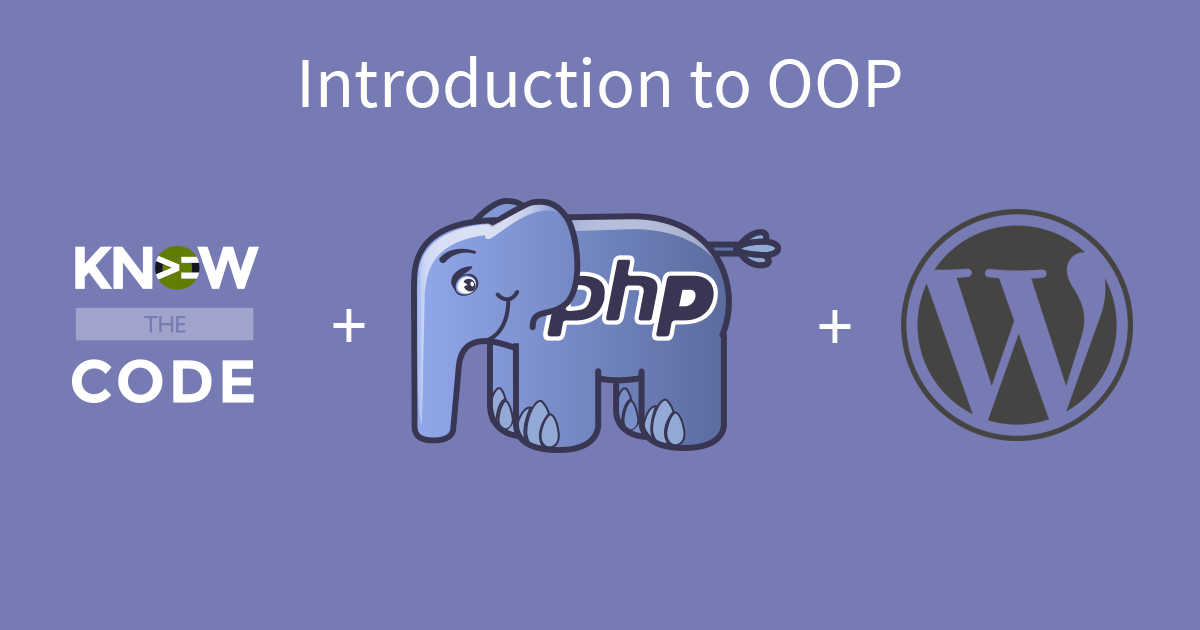
Wrap. Where to go next?
Let’s review what you learned in this lab. Then I’ll give you some resources to continue learning about OOP. Congratulations for completing this lab! Other PHP Resources A collection of other awesome educational resources. Want to really level up in PHP? Make sure you also join PHP Nomad. Leading PHP developers share their insights with you each month. Their libraries are filled with PHP awesomeness. If you are a member, they have a deal waiting for you….3 months for FREE! Object-Oriented Bootcamp in PHP – Laracasts Jeffrey Way at Laracasts has an awesome Object-Oriented Bootcamp. If you get a chance, […]
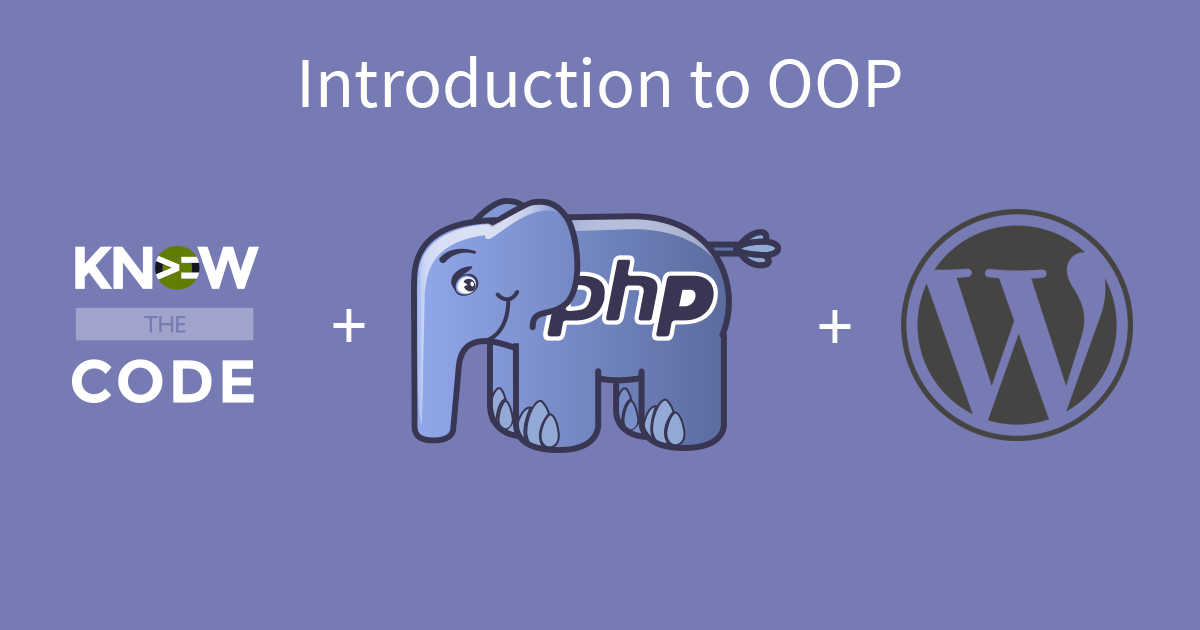
Practical Examples
Here are some practical examples of WordPress plugins that are well architected and built to clean coding standards: WordPress Custom Menu Separator by Tom McFarlin Single Post Meta Manager by Tom McFarlin Settings Page – Better Implementation by Alain Schlesser Tag Swapper by Tonya Fulcrum by Tonya Laravel’s Illuminate codebase is a beautifully crafted example of OOP and clean, quality coding. It’s more advanced, but well worth a tour through the code to see how wonderful OOP can be. Symfony is another well-crafted and architected codebase. Like Laravel, it’s both OOP and clean coding.