For the feature that fetches the FAQs by a specified topic, we need to build a custom query. WordPress provides the ability for us to configure what we want using WP_Query. Let’s walk through the configuration parameters and figure out what we need to build a query that is based upon a certain post type, taxonomy, and term slug. We’ll use the reference guide from Bill Erickson to explore the needed configuration parameters. We’ll also take a look in WordPress Core at the WP_Query class construct and talk a little bit about PHP OOP.
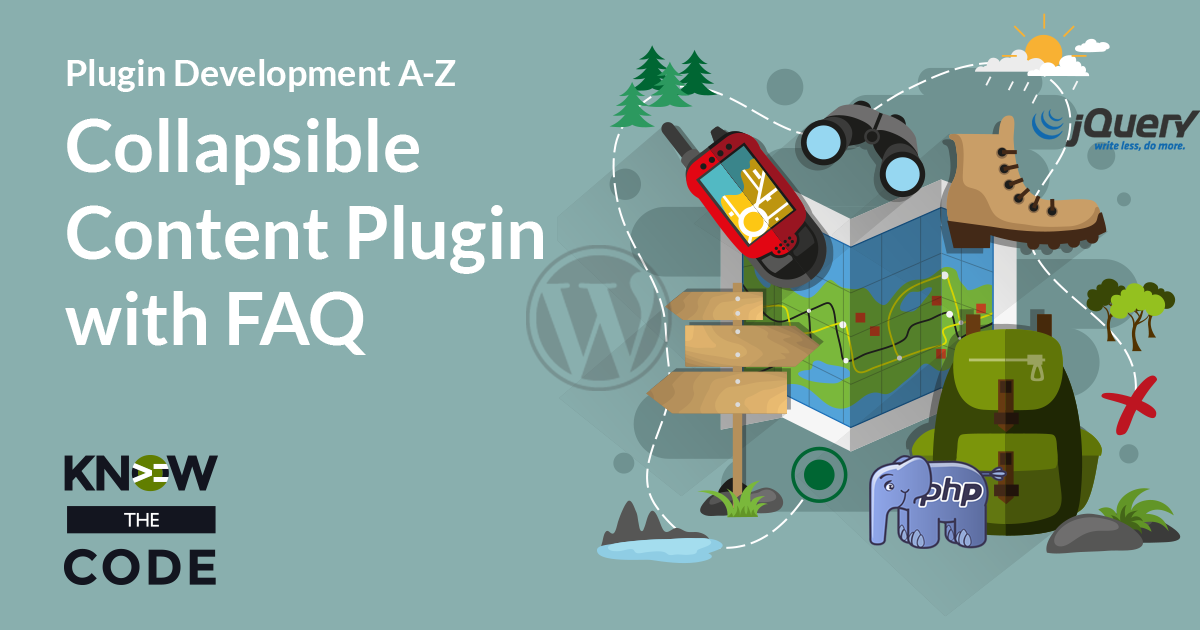