There are times when you need to control the PHP output buffer. Shortcodes are a good example of when you want to capture the buffer and return it to WordPress instead of sending it out to the browser. In this hands-on lab, you will dive into the functionality available to you for the PHP output control.
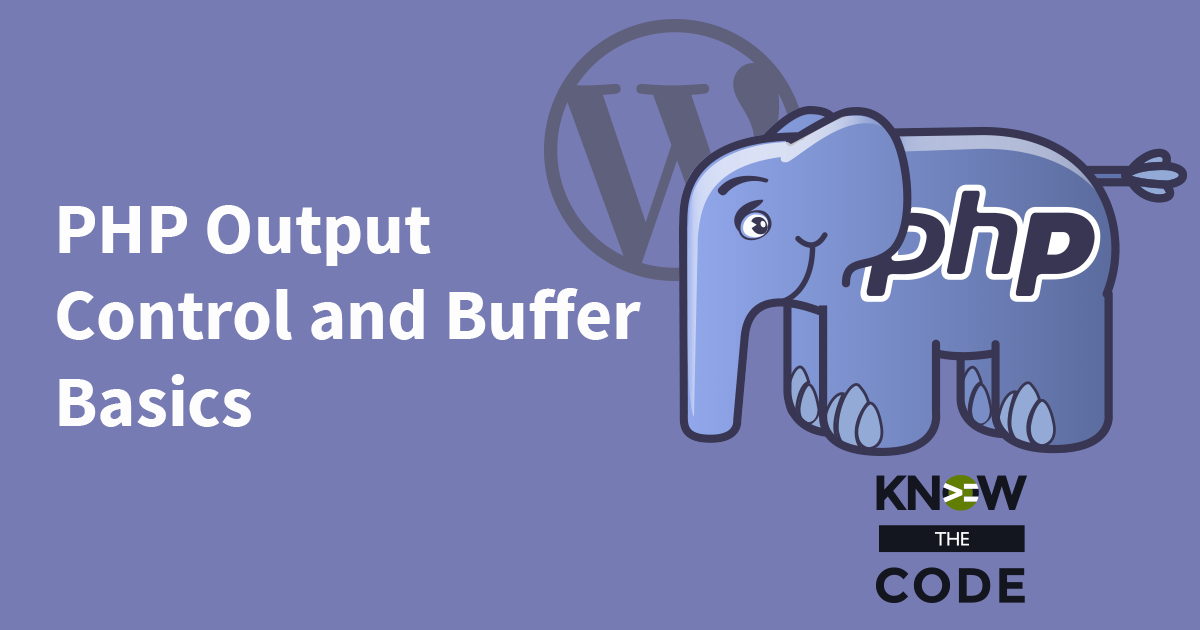