Let’s build a simple check for substring utility function and add it to our utility plugin.
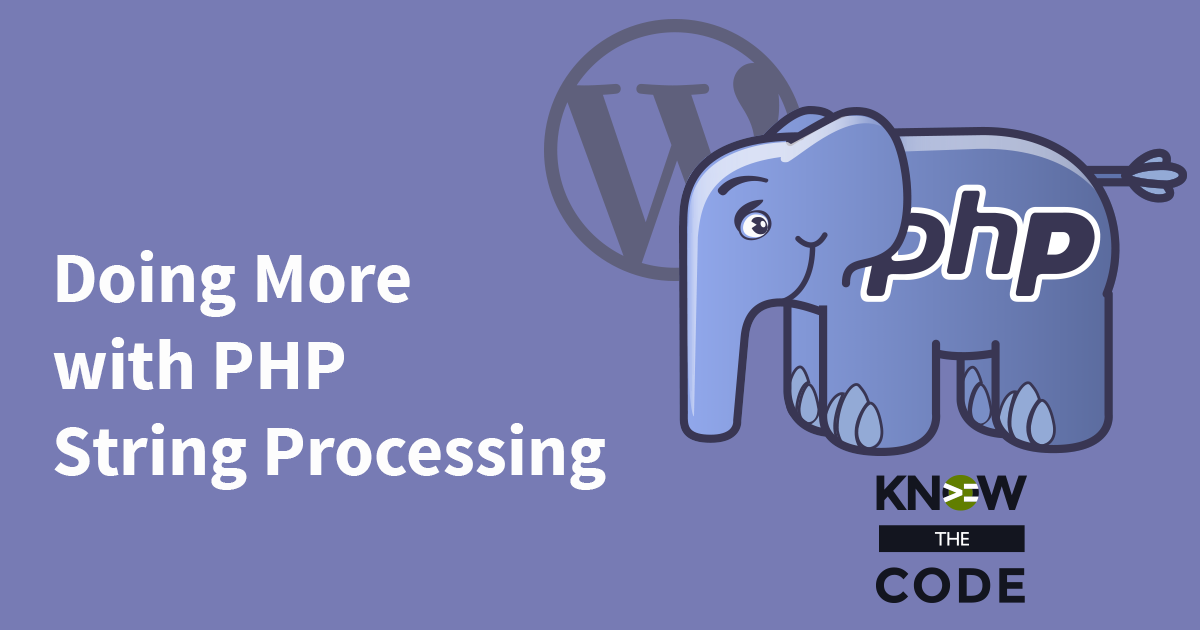
Developing & Empowering WordPress Developers
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
0 Videos Runtime
Let’s build a simple check for substring utility function and add it to our utility plugin.
0 Videos Runtime
Let’s continue working on our str_ends_with() utility function in this episode. We’ll explore the second implementation which uses the same strategy as we used in “starts with”. There is a functional difference between searching a string for its position and returning a substring from it. In this episode, you’ll implement a solution that uses mb_substr. Then we’ll break it down and discuss how each processes and why this implementation is a better solution.
0 Videos Runtime
It occurred to me that there were strategies I did in the last episode which need further explanation such as: How mb_substr works 05:21 Why did I use mb_substr instead of mb_strpos 08:40 Refactoring to make the code more clear 09:21 How does function_exists() work and why/when do you use it 11:01 Should you use namespacing or function_exists() for helper utilities In this episode, let’s dive into each of these strategies to help you figure out the best approach for you.
0 Videos Runtime
We need to check that UTF-8 encoded characters work with str_replace. In this episode, let’s continue working with the German and Russian words to verify if it works for us.
0 Videos Runtime
In the previous episode, you learned about the character sets, encoding, and that PHP has string instructions that count bytes and others that count characters. We are stepping back now to see if this also applies to strpos for checking if a substring exists in a string. Let’s add a word in both Russian and German to the content. Then let’s see if strpos gives us the right starting position for each word. Additional Resources mb_strpos strpos
0 Videos Runtime
Character set encoding matters as many language characters are not part of the standard ASCII character set. Instructions such as strlen will not behave the way you think when using it with extended characters such as €, ©, ®, à, ë, and many others. And languages such as Mandarin and Russian require a different approach when working with strings. In this episode, let’s talk about why the encoding matters and how the character set is really represented in the computer. Hint: Everything in the computer breaks down into binary, i.e. ones and zeros. Simple string instructions like strlen use bytes. […]
0 Videos Runtime
Let’s introduce you to what you’ll be doing in this hands-on lab. You will be building a plugin that houses different utility functions. In this lab, you’ll be working on string processing and checking functions. You’ll build reusable functions that you can use on every project…to save you time.
13 Videos 01:58:54 Runtime
There are many times when we need to more complex processing with strings. PHP provides us with the instructions we need to verify and manipulate strings. In this hands-on lab, you will continue learning about processing strings in PHP.
0 Videos Runtime
What if you need to repeat one of the arguments within the formatted string? You could repeat the argument in the list again like this: But then that’s a WET pattern, as you are repeating code. Instead, PHP lets you specify which argument you want for each of the placeholders. Resources sprintf – in Docx sprintf – PHP Manual printf – PHP Manual Type Casting
0 Videos Runtime
In the last episode, I had you wrap the embedded variable within curly braces. Why? Readability. Yes, you can omit the curly braces with a simple variable embed. However, to me, it’s less readable. Therefore, I advocate always using the curly braces like this: “My name is {$first_name}.” How do you embed an element from an associative array or object? These embeds are known as complex variables. For these, you must wrap the variable within curly braces. In this episode, you will walk through embedding an associative array element within a string.
Know the Code flies on WP Engine. Check out the managed hosting solutions from WP Engine.
WordPress® and its related trademarks are registered trademarks of the WordPress Foundation. The Genesis framework and its related trademarks are registered trademarks of StudioPress.
This website is not affiliated with or sponsored by Automattic, Inc., the WordPress Foundation, or the WordPress® Open Source Project.