Let’s review what you learned in this lab. Congratulations for completing this lab!
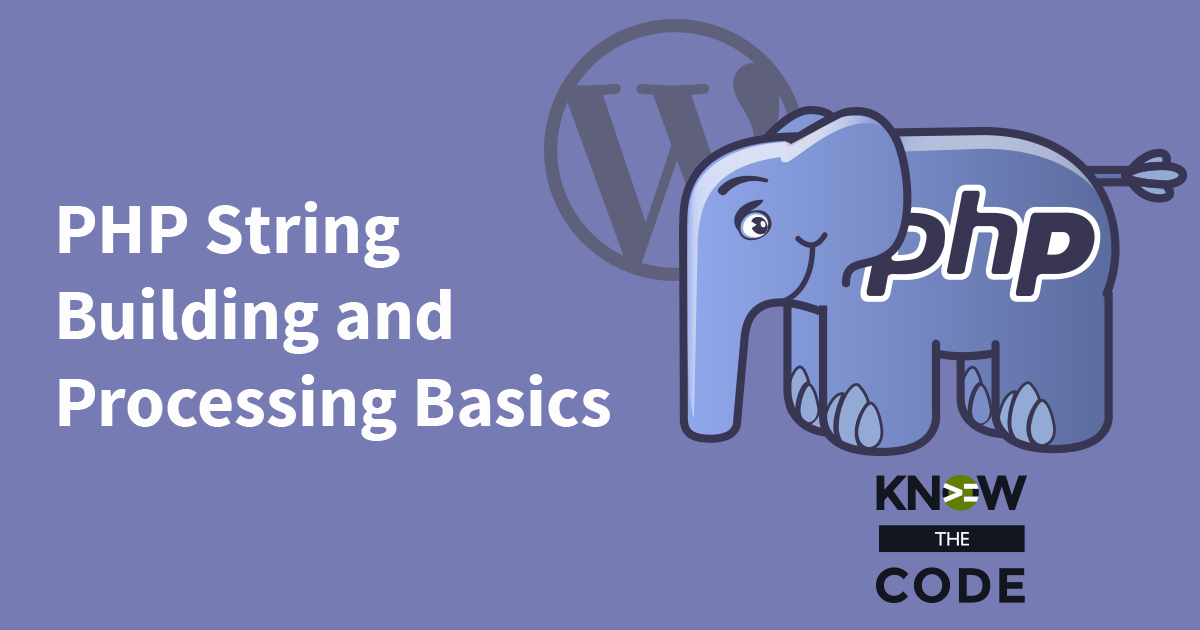
Developing & Empowering WordPress Developers
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
0 Videos Runtime
Let’s review what you learned in this lab. Congratulations for completing this lab!
0 Videos Runtime
0 Videos Runtime
Truncating or trimming the length of a string down to a set limit is a task that we do often. Right? You have a blog page and you want to show a snippet of the content, but only 200 or 300 words and not the whole 2000 word articles. WordPress has a function built in that you can use called wp_trim_words(). I have one that I’ve used which is inspired by Laravel and Symfony. It’s part of my Fulcrum plugin that I use on all of my projects. Genesis has one built into too called genesis_truncate_phrase(). There are multiple ways […]
0 Videos Runtime
There are times when you want to truncate a string by a certain number of characters. You are limiting the string to say 100 or 200 characters. How do you do that? In this episode, you and I will build the utility function that will handle it for you. Then you just call it whenever you need that feature in your theme or plugin. Resources In this utility function, you used the following instructions: mb_strwidth mb_strimwidth rtrim
0 Videos Runtime
Your next challenge is to figure out how to check for a character or substring in a given haystack (source string). Just like with the “starts with” functionality, there are a couple of different implementations. In this two-part series, you’ll explore: Implementation #1: Using a string position implementation mb_strpos by leveraging the offset argument to start exactly where we want. Implementation #2: Use the same strategy as we did with “starts with”. We’ll walk through both implementations, talk about how they work and process, and then explore which is the better solution and why.
0 Videos Runtime
In this episode, we’ll take on the challenge of how to test a string to see if it starts with “http://”. You’ll discover there are multiple ways to do this check. Then we’ll create a utility function called starts_with and put that into our new Site Utilities plugin. Regex is not needed here. In this episode, I show you two different approaches to checking if a string begins with a substring: Check its position using strpos Check the beginning of the string to a specific length using mb_substr and mb_strlen
0 Videos Runtime
0 Videos Runtime
0 Videos Runtime
There are times when you need to know the length of a string. For example, if you are checking for a string and you want to know if it’s at the end of another string, then you need to know the length of what you are looking for first. That’s a good example. Let’s get you started learning about string lengths, how it’s processed (character vs. byte count), and character encoding. In this episode, you’ll work with the PHP instruction strlen. This instruction counts the number of bytes in the string, not the number of characters. For now, don’t worry […]
0 Videos Runtime
Frequently, you need to strip off unwanted characters or entities from the start and end of a string. For example, form field submissions, such as custom fields, typically require you to clean them up before processing. When you pull data from the database, many times, you need to clean it up before you process and then render it out to the browser. In this episode, you’ll experiment with and learn about trim. You’ll pass character masks to it to see how you can control what is stripped off.
Know the Code flies on WP Engine. Check out the managed hosting solutions from WP Engine.
WordPress® and its related trademarks are registered trademarks of the WordPress Foundation. The Genesis framework and its related trademarks are registered trademarks of StudioPress.
This website is not affiliated with or sponsored by Automattic, Inc., the WordPress Foundation, or the WordPress® Open Source Project.