Out-of-the-box, WordPress gives you built-in taxonomies which are used to group like or similar content together. You use categories and tags to add contextual information about the content and give readers additional mechanisms to explore and discover. In this hands-on lab, you will interact with and change the built-in taxonomies. This is an introduction to taxonomies, which will prepare you for building your own custom taxonomies.
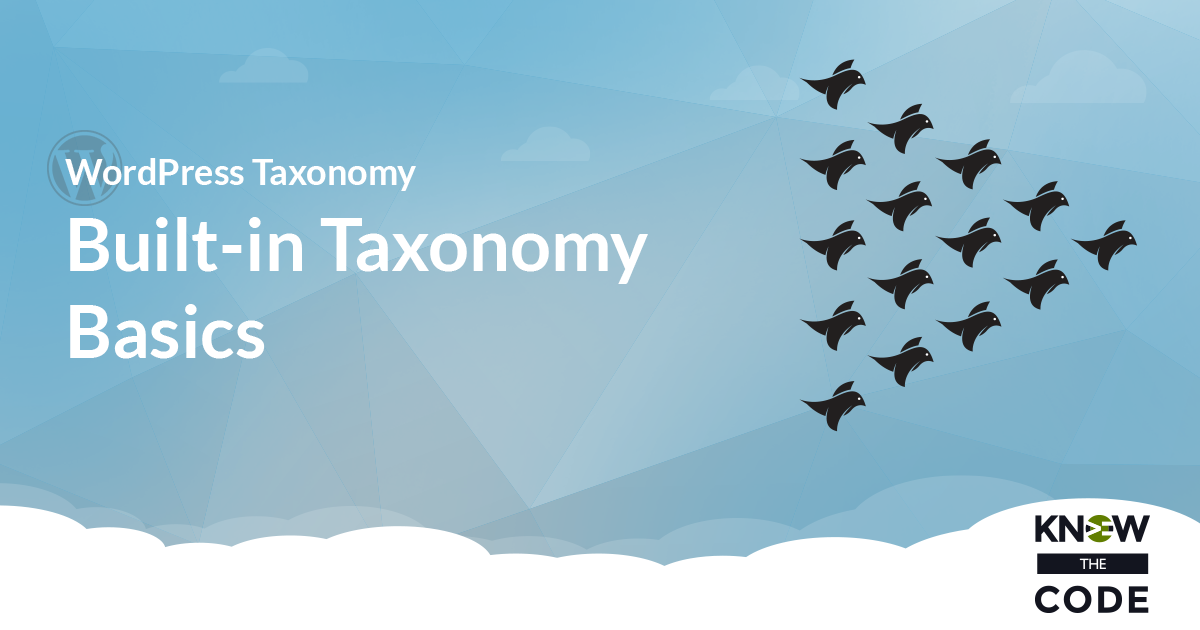