Let’s do some refactoring to make our JavaScript easier and remove the redundancy. The script doesn’t care that one is a marketing teaser and the other is a Q&A. Right? The script handles both of the same way. Why? Both will slide down to reveal the hidden content upon clicking the visible part. The animation and changing of the icon will be the same. Therefore, let’s do some refactoring. You’ll change the class attributes to serve both the styling and JavaScript. And let’s talk about the thought process as we do it.
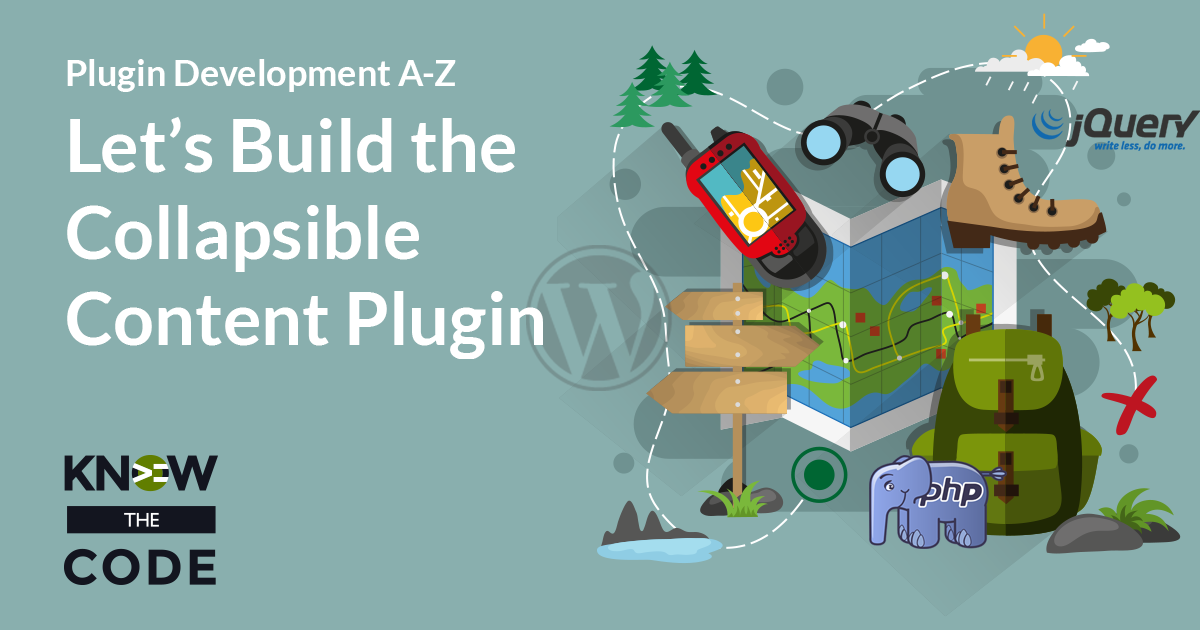