Let’s do a quick review of data types in JavaScript. We’ll talk about primitive types (string, number, boolean, undefined, and null) as well as objects and arrays. You will learn: JavaScript is a loosely typed (dynamic) language, meaning the data type is assigned by its value Changing data types Checking what the data type is with typeof Arrays and Objects For a more in-depth lesson on data types, make sure you sign up for JavaScript for WordPress and/or MDN JavaScript data types and data structures.
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
Abstract Data Type
The abstract data type, or ADT, is a container whose properties (characteristics) and functionality are separate from the implementation. Let’s take about it. Study Notes What is abstract? Per Merriam-Webster: relating to or involving general ideas or qualities rather than specific people, objects, or actions We’re separating the general from the specific. What is an Abstract Data Type (ADT) Container Properties (data and functionality) are independent from an implementation View the type from the application’s (or user’s) perspective Model to “abstract” away complexity (abstraction) Lists Designed specifically for the needs of a list Properties and functionality to track the list […]
Special Data Type
Special data types are specified by the programming language. One example is the enumeration. Let’s explore this data type. Your key takeaways are: Special data types are specified by the programming language Enumeration is a common data type It is an example of using the right tool for the job Study Notes It’s a data type specific to a particular language. One fairly common data type is an enumeration. Examples of Enumeration enum Month (JAN, FEB, MAR, APR, MAY, JUN, JUL, AUG, SEP, OCT, NOV, DEC); enum Season (SPRING, SUMMER, FALL, WINTER); enum Users (SUBSCRIBER, CONTRIBUTOR, AUTHOR, EDITOR, ADMIN, SUPERADMIN); […]
Composite Data Type
Composite data types are a combination of primitives and other data types. They include arrays, lists, and collections. Your key takeaways are: Composite data types include arrays, lists, and collections Combination of primitives and other data types Arrays ordered arrangement of data each element keyed – implied or declared value held in the element can be any data type Study Notes What is a Composite Data Type? Combination of the primitives and other composite types It’s a composition of different types Examples of Composite Data Types Array Collection What is an Array? An array is an ordered arrangement of data. […]
Primitive Data Type
The primitive data type is the basic building block of data. They hold one single value. They represent a string, number, or boolean. Your key takeaways are: The basic building blocks of data are the primitives Primitives hold a single value representation for string, number, and boolean Each language has these building blocks Study Notes All data types are built off of the primitive types, so we’ll start with these. Different Data Representations What patterns do you see in the following table? Why are patterns important? Patterns tell you a story. Patterns give you clues. Start watching for patterns. How […]
Lab Introduction
Data is a big part of what you do, no matter which language you’re working with. In this lesson, we’ll talk about the different types of data type classifications. Data Type Classifications Primitive Composite (e.g. array and collection) Special (e.g. enumerated) Abstract (e.g. list, objects) Why are Data Type Classifications Important? What are some of the reasons you need to know about these data types? You need to understand how the data is being computed. Knowing how each of these data types is represented helps you avoid unexpected results. What happens when you compare a floating point to an integer […]
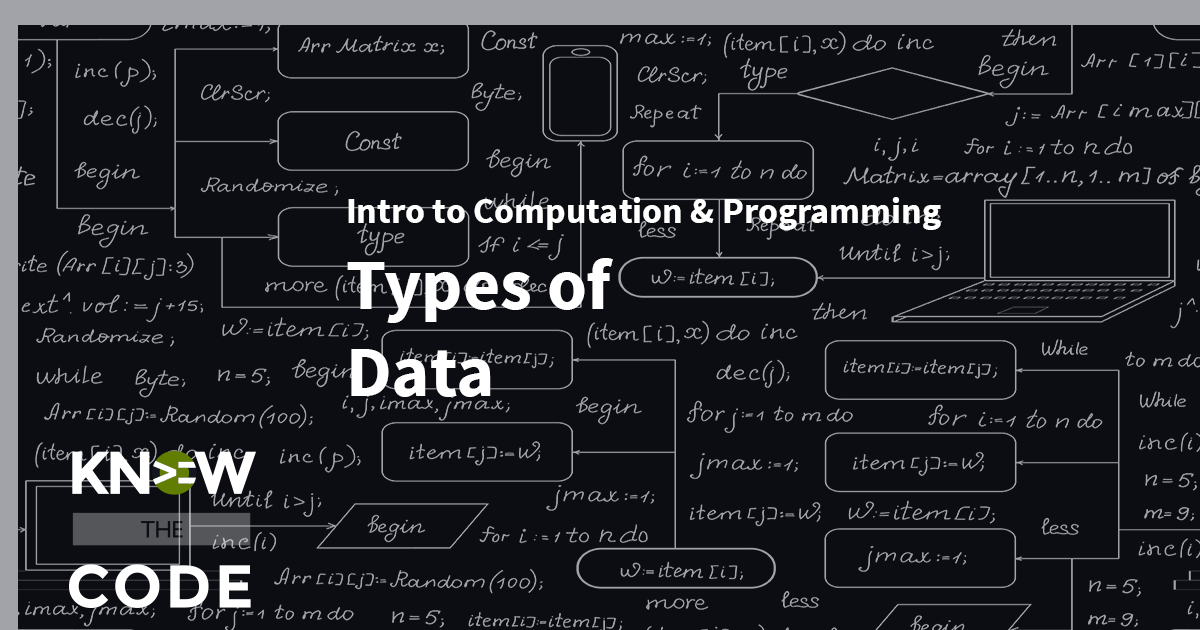
Types of Data
Data is a big part of what you do, no matter the programming language. In this lesson, we’ll talk about the different types of data type classifications including: primitives, composition, special, and abstract data types.
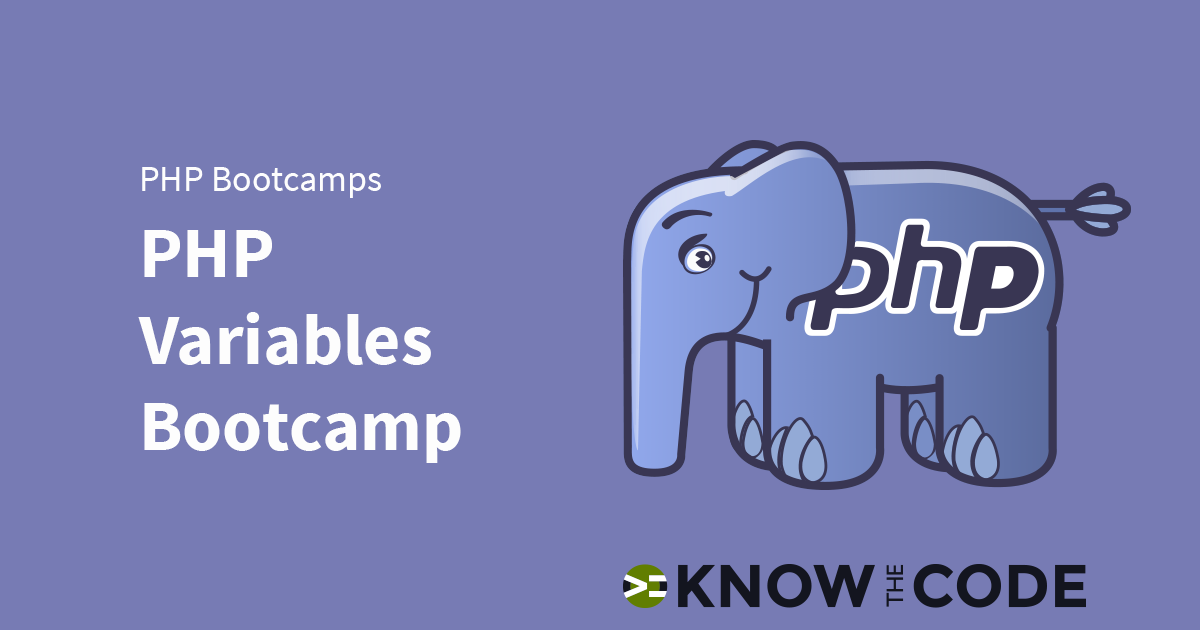
Type Juggling
PHP does Type Juggling and determines the data type of the variable based upon its context. You can learn more about PHP Type Juggling in the Docx.