Let’s build the centralized data, state, and configuration parameters store in an Object Oriented (OOP) architecture. What You Will Learn X Prerequisites See the list of prerequisites and suggestions on the series landing page.
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
Centralized Data, State, & Config Store – Built in OOP
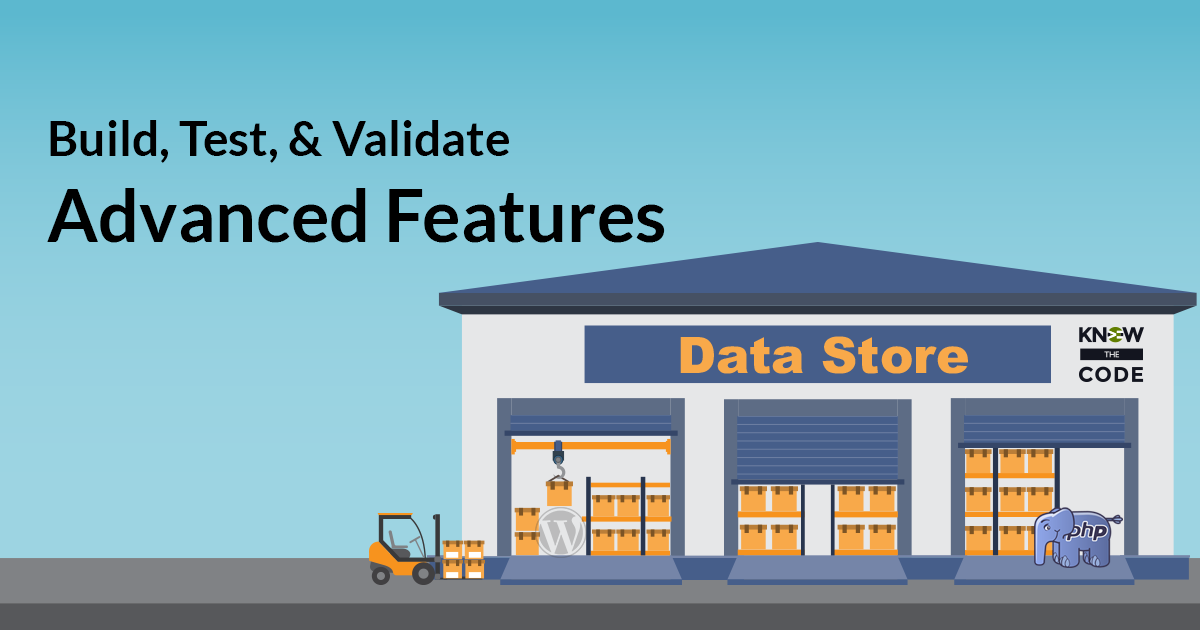
Data Store – Build, Test, & Validate the Advanced Features
This is part 4 of the series. In this hands-on coding lab, you’ll build, test, and validate more advanced features for the data store, thereby extending its functionality and utility.
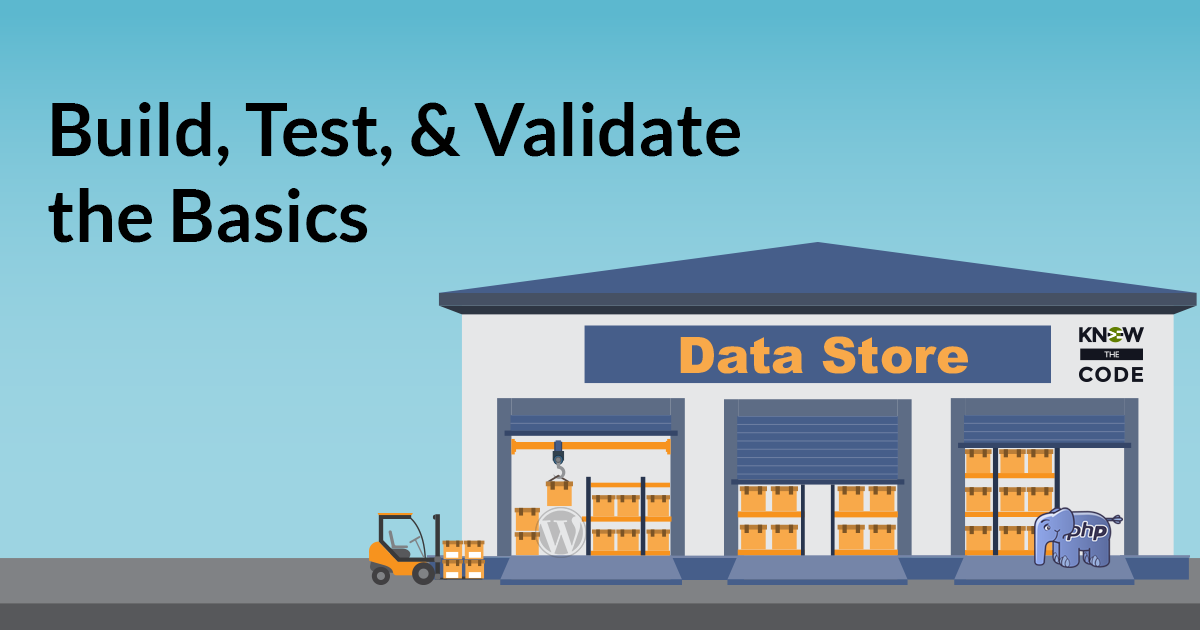
Data Store – Build, Test, & Validate the Basics
Let’s build, test, and validate the basics of the data store. In this hands-on coding lab, you’ll build the basic functionality and attributes of the data store in 3 different implementations: procedural, static class, and OOP. You’ll build a full test suite. And you’ll validate that the code complies to the WordPress Coding Standard.
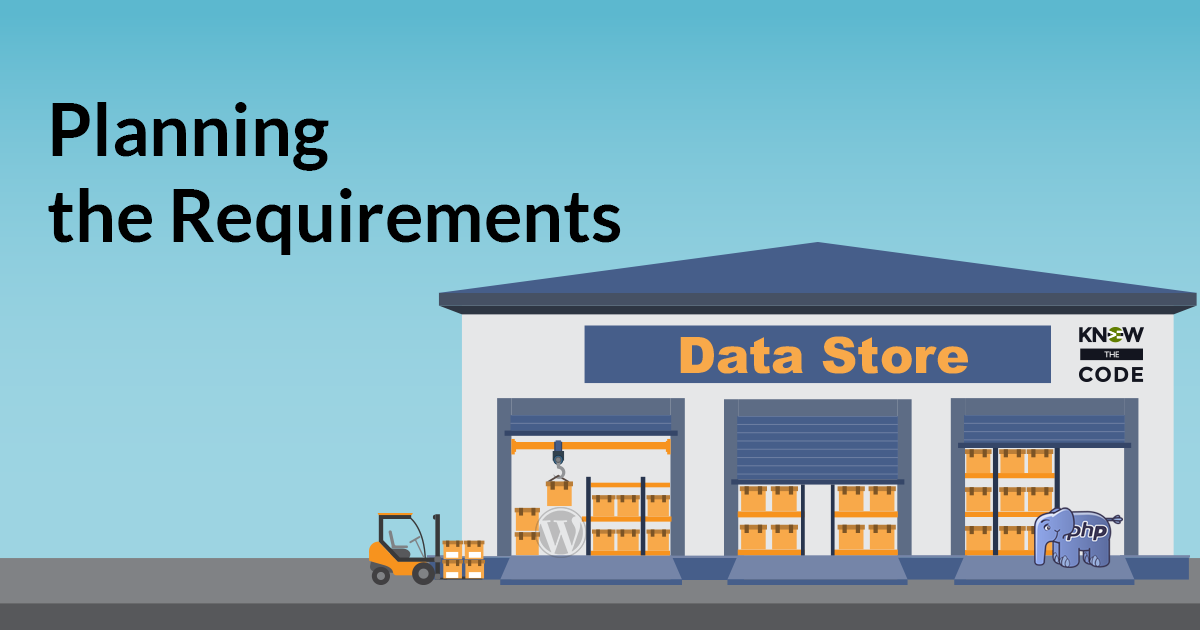
Data Store – Planning the Requirements
Before we can build the data store, we need to understand what it is we need to build. In this lab, you’ll think through each of the requirements, working to define what makes a store a store, how to interact with it, what functionality is needed, and conceptually how to achieve it, and then what approaches you’ll employ in the series.
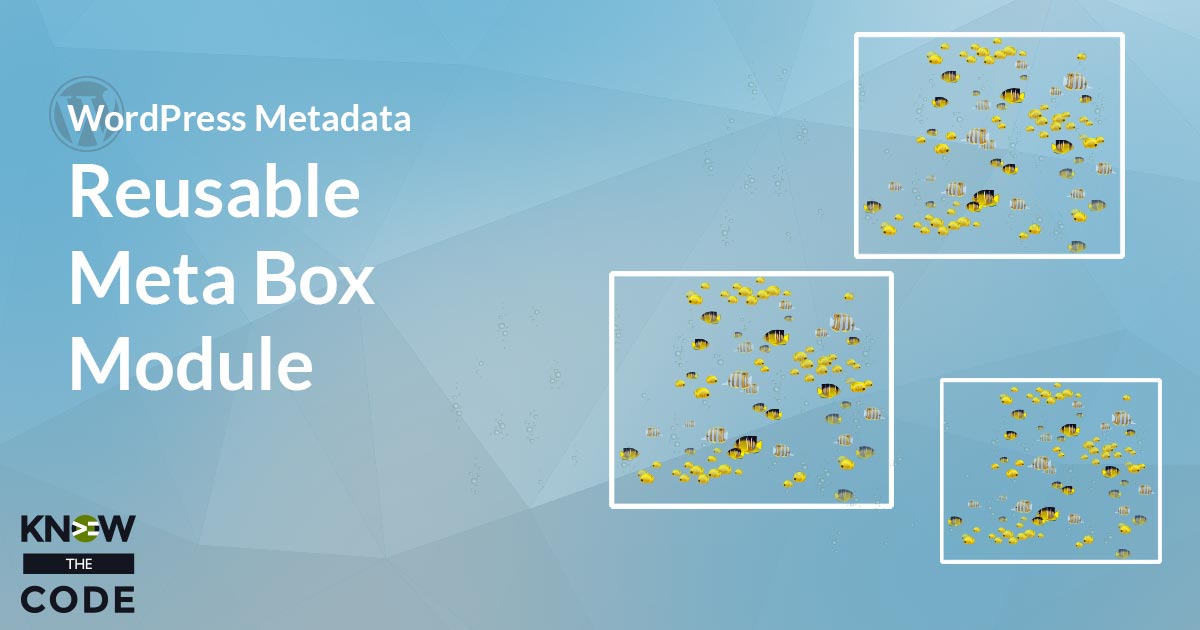
Get Only the Meta Box Keys From ConfigStore
We have a problem with our architecture. Do you know what it is? We have no way to differentiate and fetch only the keys for a specific component or module. In your work, you’ll likely have multiple modules using the ConfigStore. For example, you may have shortcodes, widgets, meta boxes, and custom post types all loading configurations into the store. How can you get only the configurations for the meta boxes? Right now, you can’t. In this episode, we’ll walk through a refactoring process to provide the means of fetching keys for just that component or module level. You’ll add […]
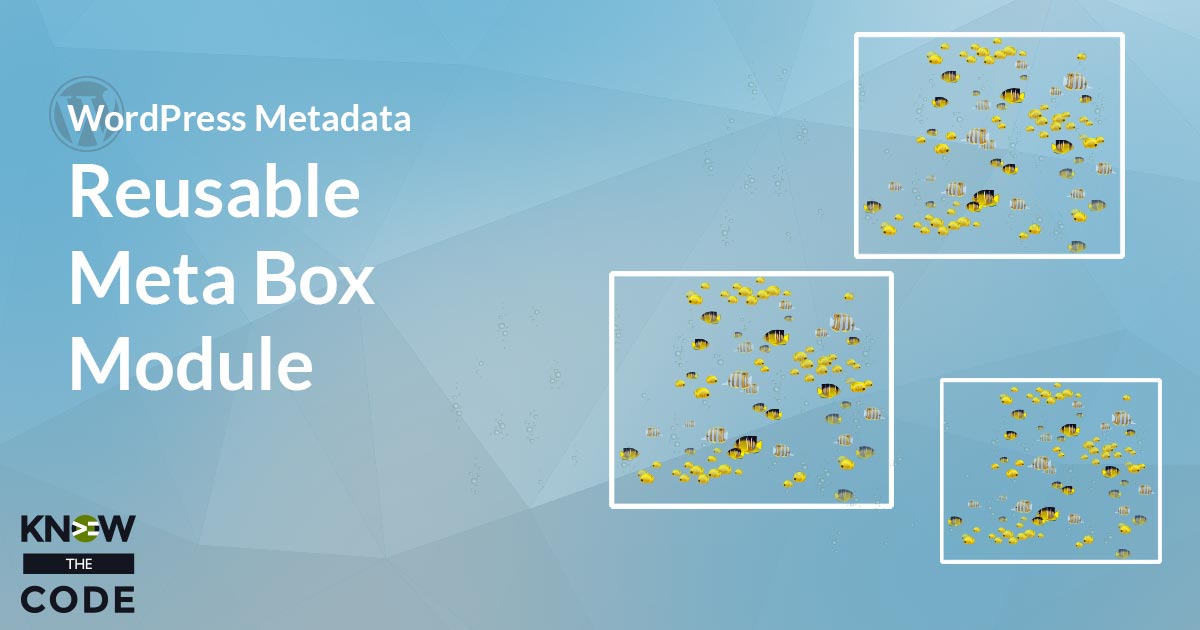
Architect the ConfigStore – Part 2
Continuing from Part 1, let’s layout our configuration store’s module, api, and internals. We are going to design this module in procedural, although you could design it in class wrapper (static functions within a class structure) or OOP. We’ll use a static variable to be our container. Resources Static Variables in PHP Static Variables – PHP Internals PHP Manual – Static Variable
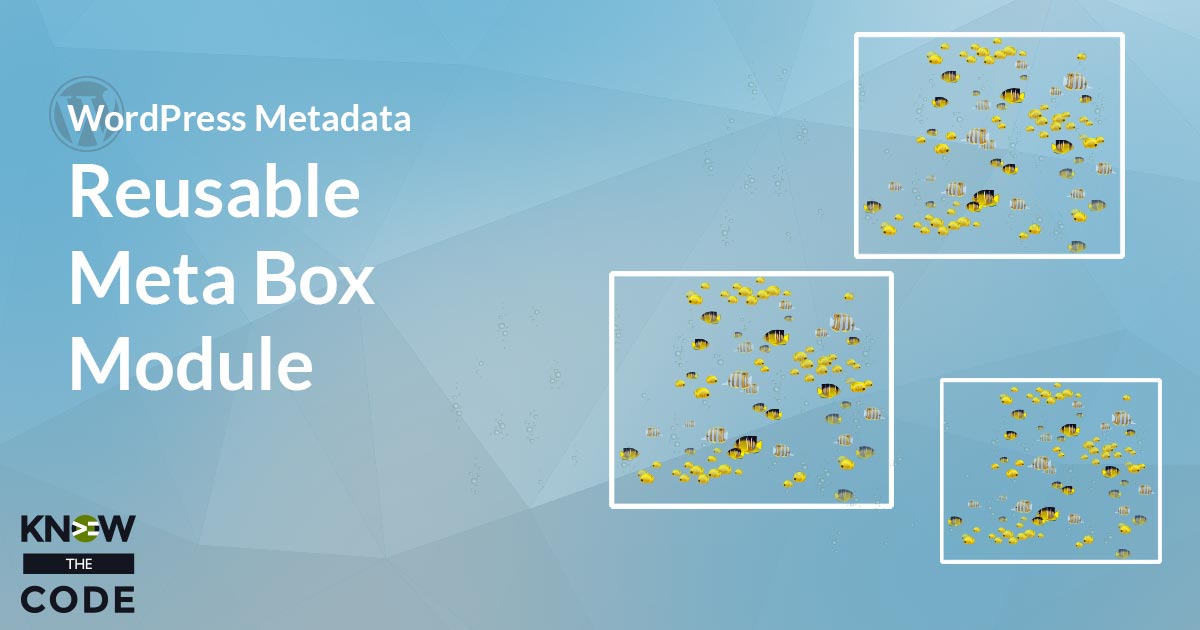
Separating Implementation from Business Logic
As you are developing your Reusable Mindset, I want you to think about implementation and business logic. More specifically, think about how to separate a specific implementation from the business logic. This design pattern makes your code reusable. For example, in our project, we have 2 custom meta boxes. Yet, both are using the same code. Imagine pulling the implementation out of the code and abstracting it into a configuration file. Now imagine that our business logic is rebuilt to accept a configuration file and then it processes it. Why? In doing so, our business logic is built once. It […]
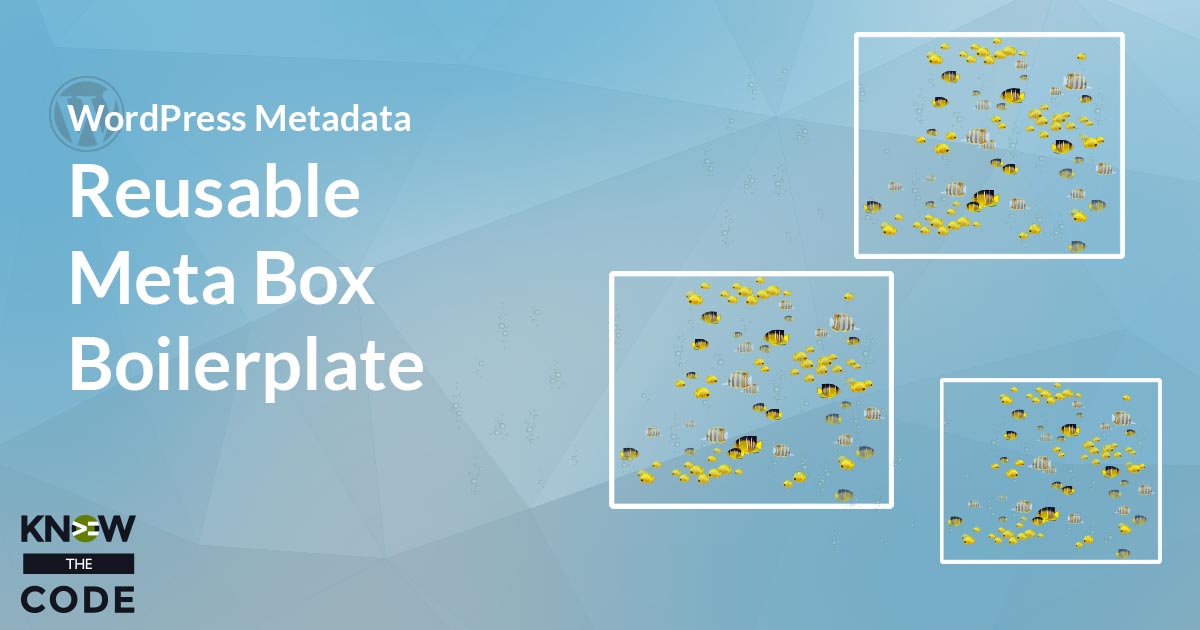
Improving the Render
Let’s improve the render function, as we have too much going on in that function. We’ll abstract away the task of fetching the metadata from the database for our custom fields’ values. Why? Our render function’s job is to render the view. It needs the values, but does not need to know how to do it. Rather, just gimme the values for the custom fields. We’ll also add a WordPress filter event to extend our meta data, allowing an external process to filter the values, do some processing, or just run before we render out to the browser.
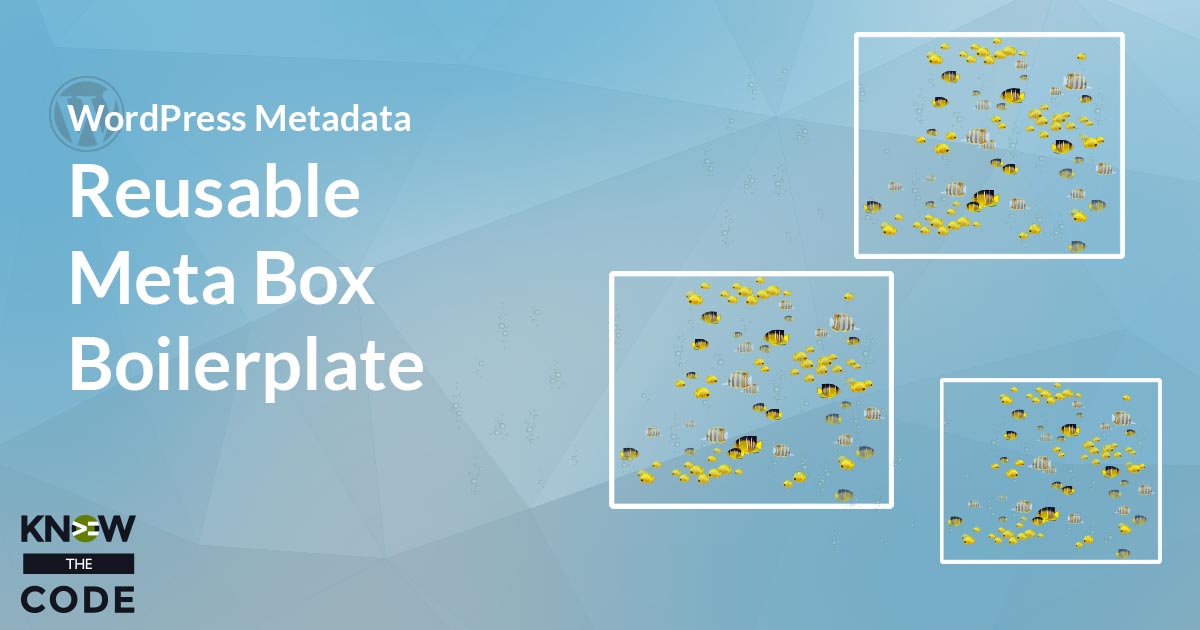
Rethink the Save Functionality
Our current save function is not reusable. As we’ve done with the register and render functions, we need to remove the implementation and allow the saving process to accept a configuration and then process it. Let’s rethink our save function, split up the different parts, and prepare for how to refactor it.