Let’s build the centralized data, state, and configuration parameters store in an Object Oriented (OOP) architecture. What You Will Learn X Prerequisites See the list of prerequisites and suggestions on the series landing page.
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
Centralized Data, State, & Config Store – Built in OOP
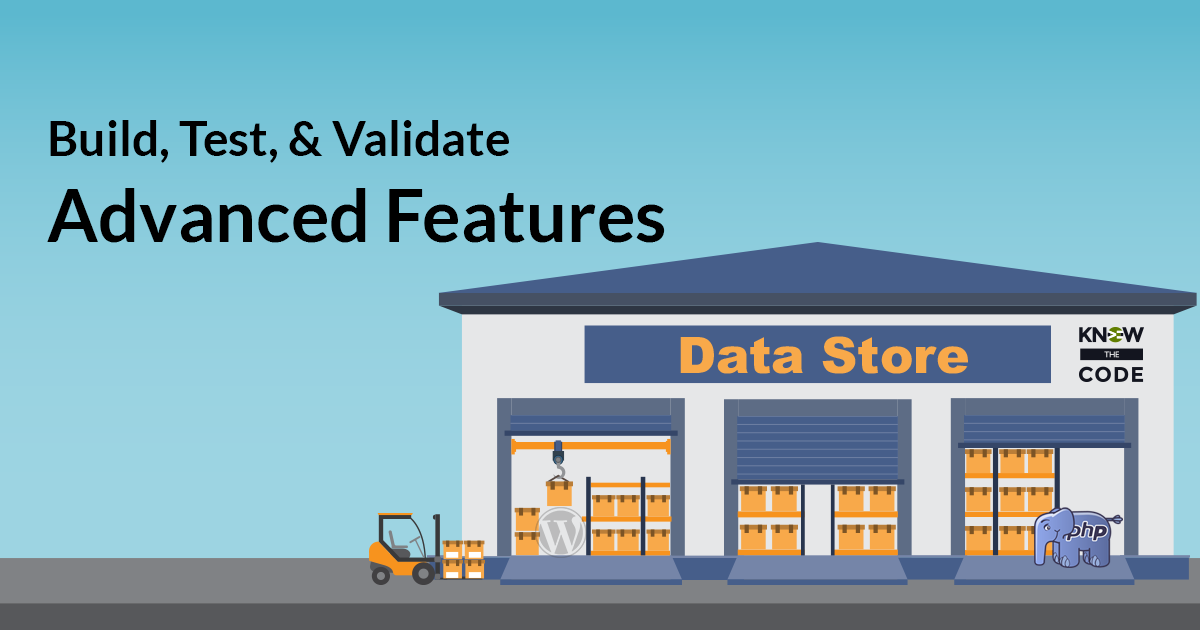
Data Store – Build, Test, & Validate the Advanced Features
This is part 4 of the series. In this hands-on coding lab, you’ll build, test, and validate more advanced features for the data store, thereby extending its functionality and utility.
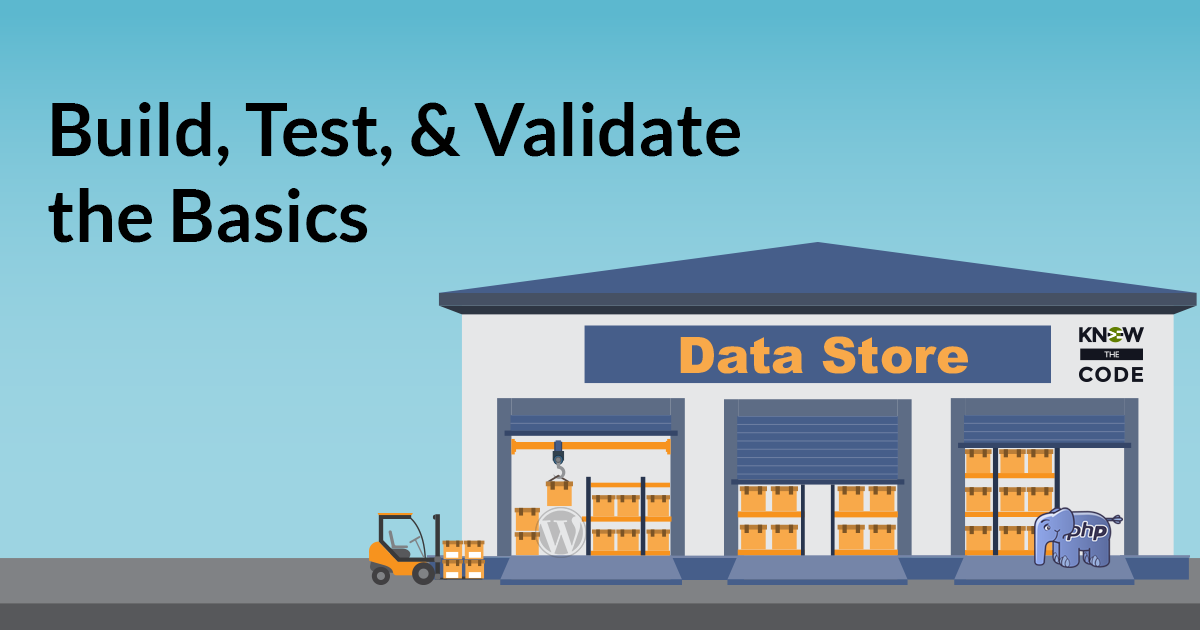
Data Store – Build, Test, & Validate the Basics
Let’s build, test, and validate the basics of the data store. In this hands-on coding lab, you’ll build the basic functionality and attributes of the data store in 3 different implementations: procedural, static class, and OOP. You’ll build a full test suite. And you’ll validate that the code complies to the WordPress Coding Standard.
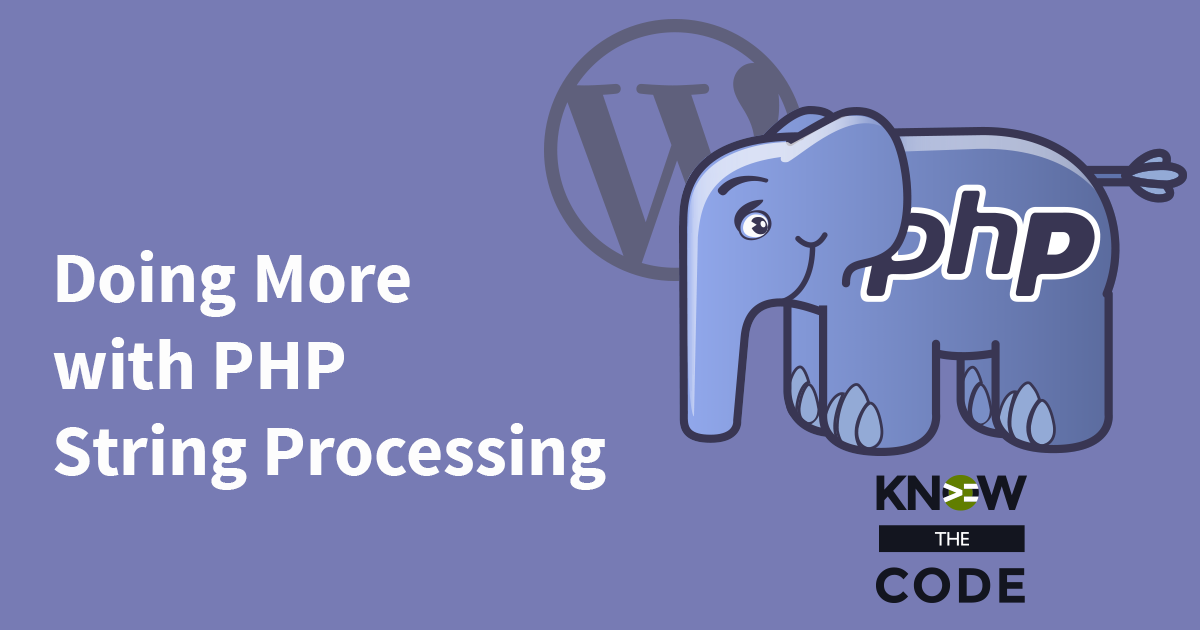
Explaining the “String Starts With” Strategy
It occurred to me that there were strategies I did in the last episode which need further explanation such as: How mb_substr works 05:21 Why did I use mb_substr instead of mb_strpos 08:40 Refactoring to make the code more clear 09:21 How does function_exists() work and why/when do you use it 11:01 Should you use namespacing or function_exists() for helper utilities In this episode, let’s dive into each of these strategies to help you figure out the best approach for you.
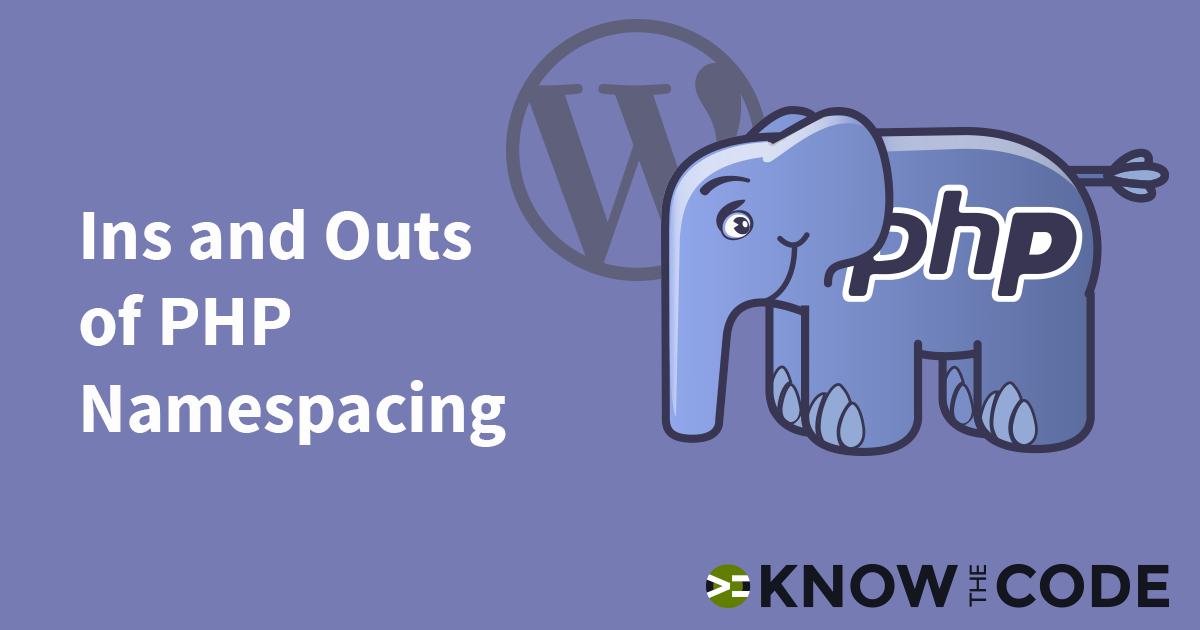
Callbacks and Namespacing
In WordPress, we register callbacks to events by using add_action or add_filter. In the last episode, you learned that you must specify the fully qualified name to invoke a function (i.e. run it) outside of the namespace. This applies to add_action or add_filter. In this episode, you will learn why as well as a shorthand version using the magic constant __NAMESPACE__.
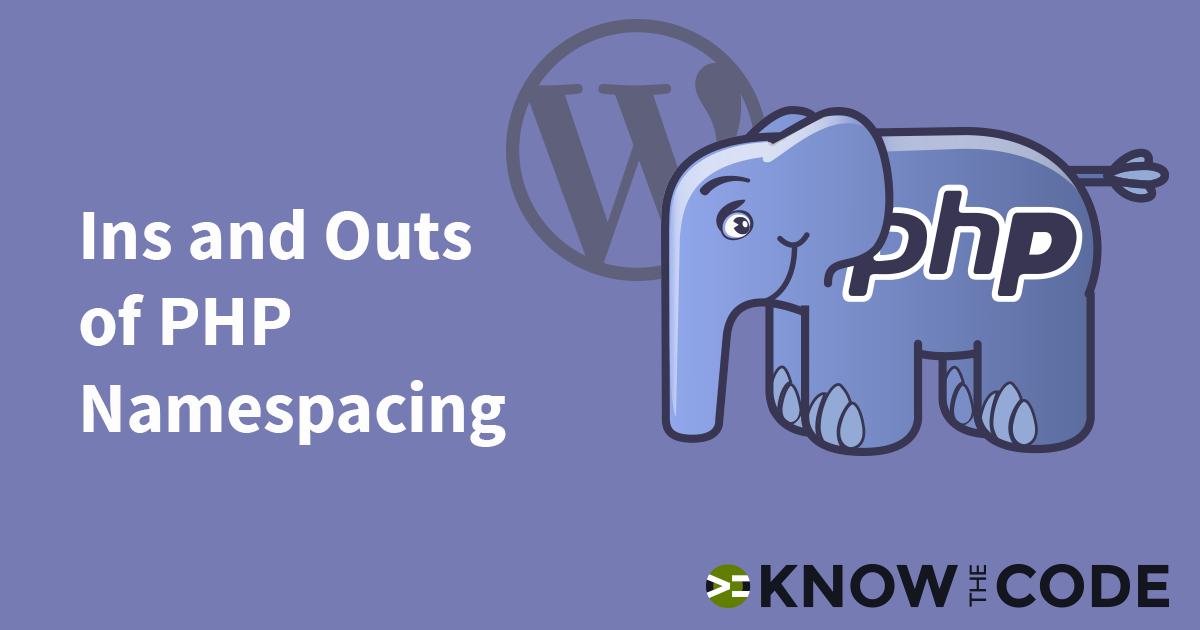
Wrap it Up
Whew, you did it! Congratulations for completing this lab! Let’s review what you learned as well as show you the code here on Know the Code. Remember, namespacing is only available in PHP version 5.3.0 and up. That shouldn’t be a problem for you. Why? Because 5.3 stopped being supported back in 2014. It’s old. Anything older than it can be problematic. Therefore, build your code to be at least 5.3 and up. You can check the PHP supported versions by clicking here.
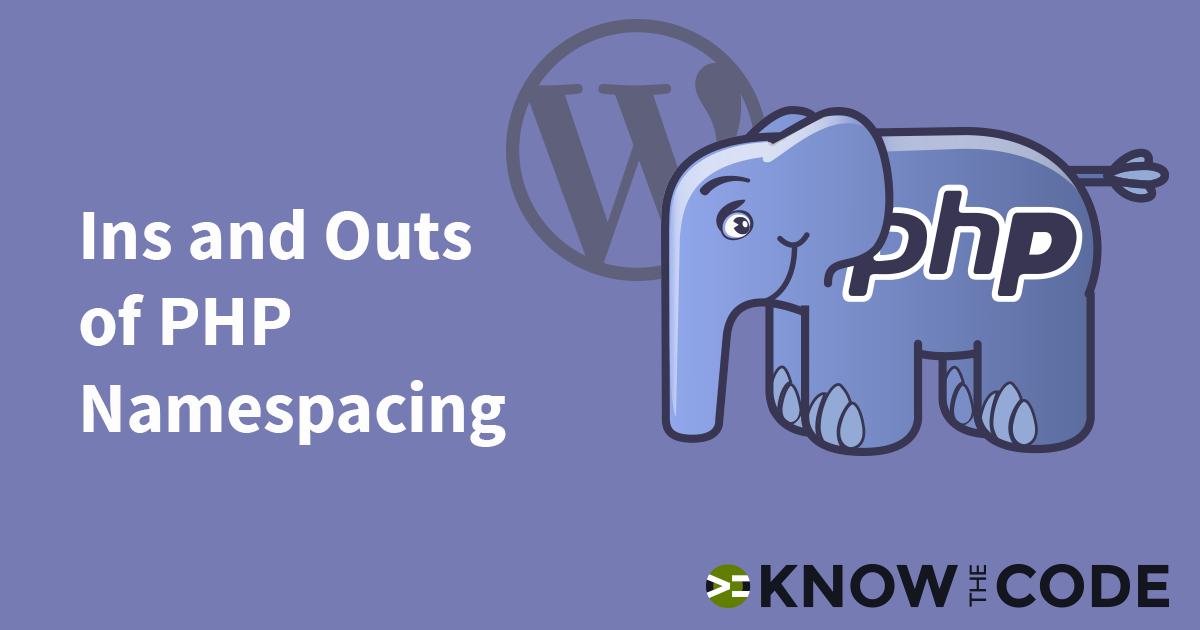
Architecture – Building in Packages and Modules
In this episode, let’s stop and talk about how you architect your code. Code should be built in modules. These modules are not assembled into your plugin or theme. It’s not snippets of code. Rather, they are complete, fully-tested, ready-to-go component or feature modules. All you have to do is change the configuration and none of the codebase. That makes it a reusable module. For example, let’s say you are building a theme. In your code libraries, you have modules for: WooCommerce bbPress LearnDash MemberPress BuddyPress Customizer and more The theme you are building needs WooCommerce, bbPress, and Customizer. You […]
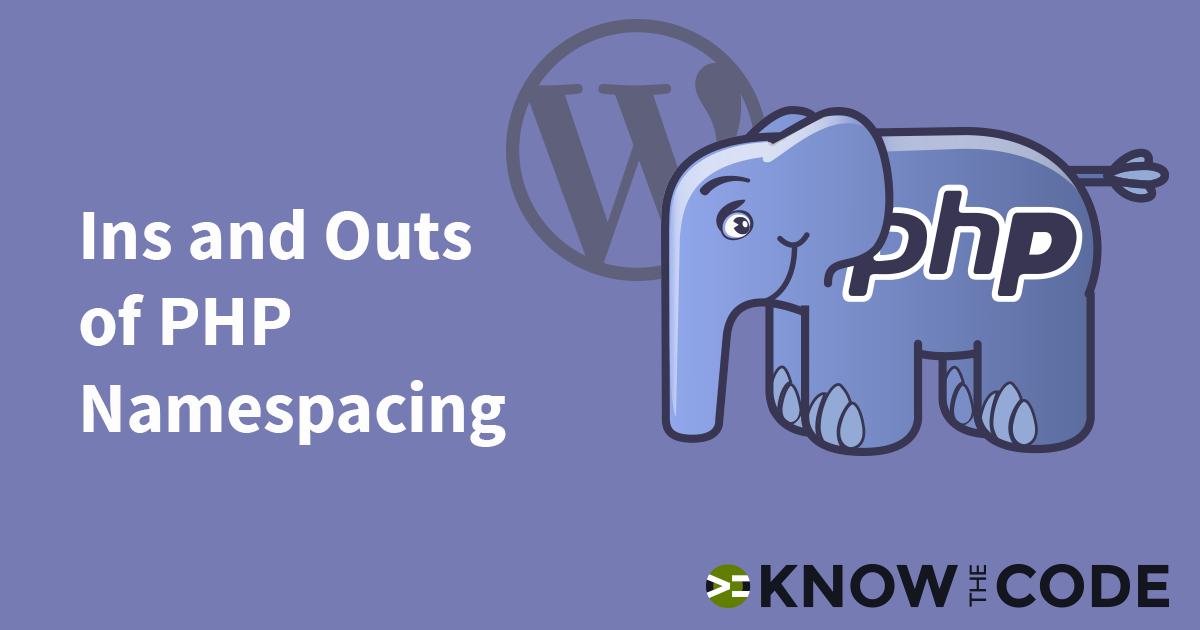
Real World Example: Convert from Prefixing
Next, let’s take a real world plugin and convert it from prefixing to namespacing. Let’s convert Tom McFarlin’s Easier Excerpts plugin. You can install this plugin within the WordPress back end by going to Plugins > Add New and then type in Easier Excerpts. In this episode, you will walk through the steps to remove the prefixing, add in the namespacing, and provide better function naming that starts with a verb.
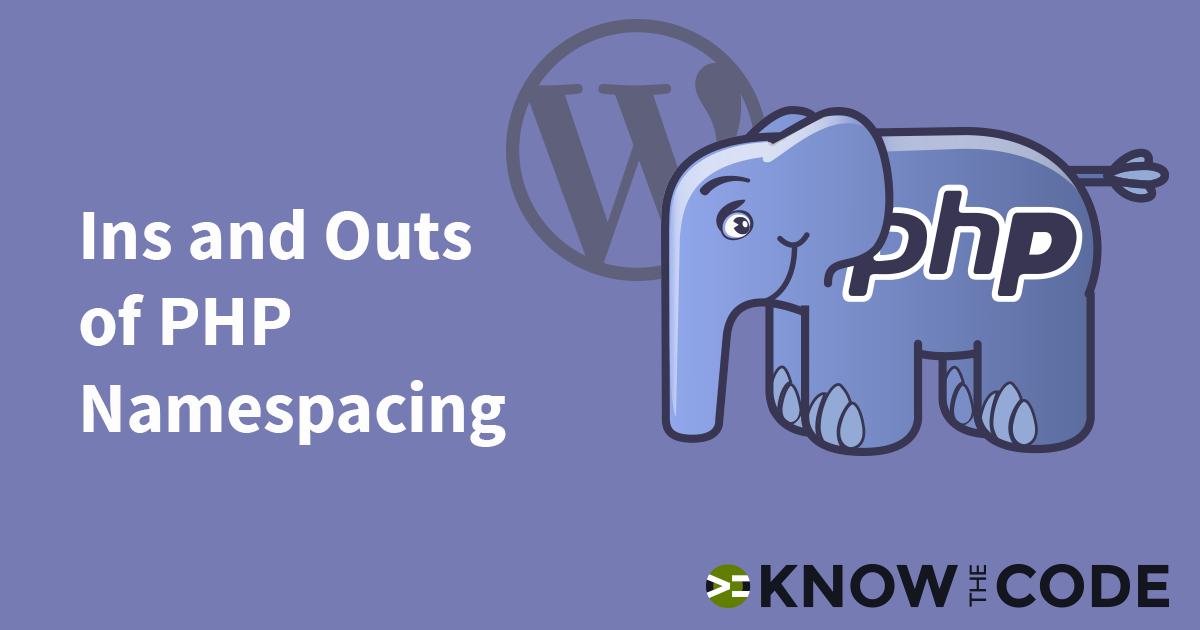
Name Resolution Rules
Next, let’s talk about PHP name resolution rules. That means: how PHP figures out what code to run for the given name. If you look at the PHP Manual, they give you a techie write-up that likely will confuse you. Essentially, here’s what you need to know: How you declare the function is the starting point. If you include the fully qualified name, you are telling PHP exactly which function you want; whereas, if you leave off the namespace, then it walks through its process ordering to figure out which function to run. You can name using: Unqualified name: no […]