Let’s put your name skills to use. In this episode, you will convert the supplied plugin from prefixing to PHP namespacing. The best way to learn this is to start where you are, i.e. in prefixing, and walk through step-by-step the process of making a plugin namespaced. You’ll change callbacks, use the magic constant __NAMESPACE__. You’ll remove the namespacing. Step-by-step. Let’s do this together.
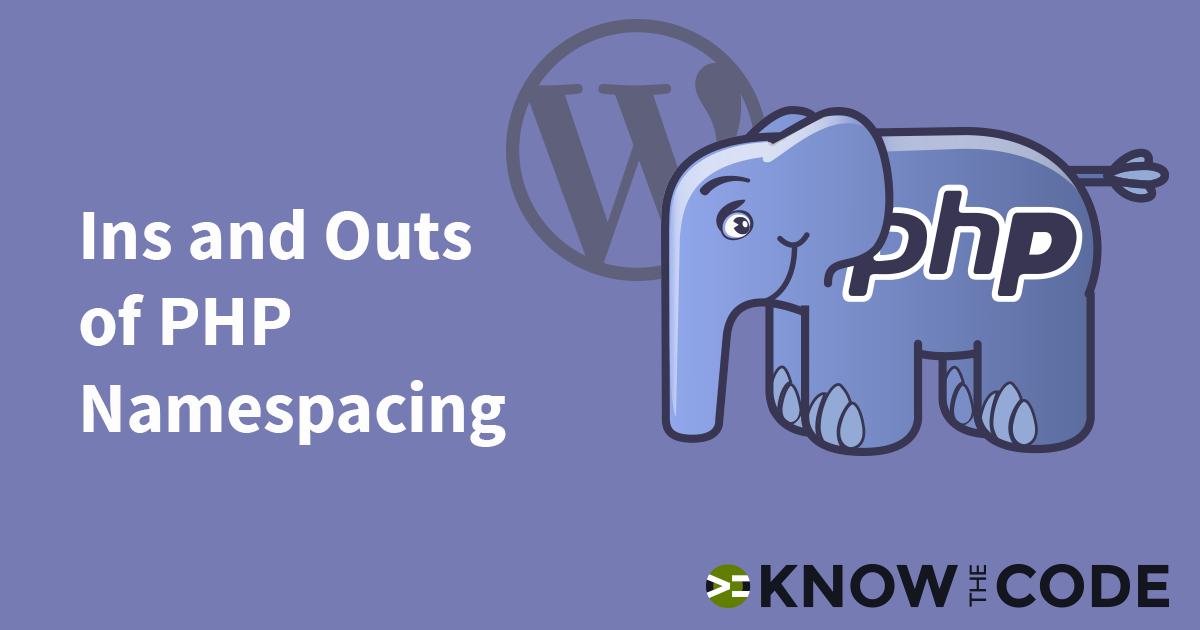