The intent of global variables is to allow all of the code, all plugins and themes, to be able to both read and write to the variable. You want anything within the website’s PHP code to have full, unprotected access to the data that your variable represents. That means that any code within the website can change the data. For example, $wp_query is a global variable. Therefore, any piece of code in WordPress Core, plugins, and theme can both read and change the data.
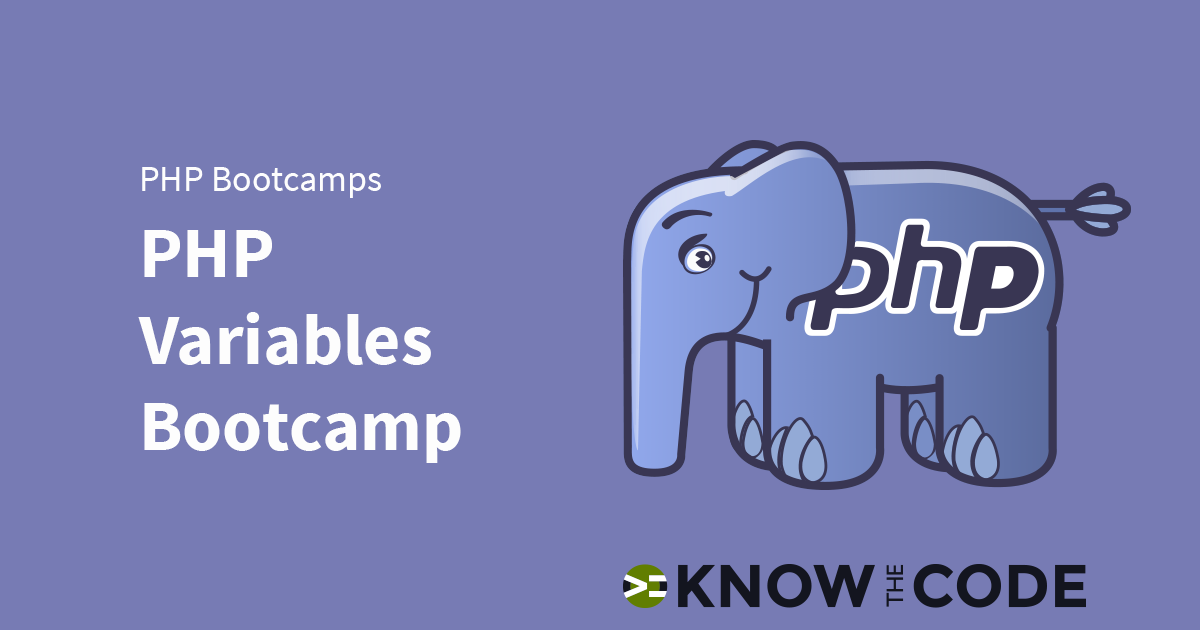