The primitive data type is the basic building block of data. They hold one single value. They represent a string, number, or boolean. Your key takeaways are: The basic building blocks of data are the primitives Primitives hold a single value representation for string, number, and boolean Each language has these building blocks Study Notes All data types are built off of the primitive types, so we’ll start with these. Different Data Representations What patterns do you see in the following table? Why are patterns important? Patterns tell you a story. Patterns give you clues. Start watching for patterns. How […]
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
Lab Introduction
Data is a big part of what you do, no matter which language you’re working with. In this lesson, we’ll talk about the different types of data type classifications. Data Type Classifications Primitive Composite (e.g. array and collection) Special (e.g. enumerated) Abstract (e.g. list, objects) Why are Data Type Classifications Important? What are some of the reasons you need to know about these data types? You need to understand how the data is being computed. Knowing how each of these data types is represented helps you avoid unexpected results. What happens when you compare a floating point to an integer […]
Pseudocode
Pseudocode helps you to transition from visual mapping, as you did in the last episode, to a textual format. It’s written in a generic non-state specific format. It’s the building block of code before you write your actual code.
Computational Visualization
In this episode, you will learn about different techniques for visualizing computation. You’ll learn about how to conceptual view your code, do visual mockups and maps, flowcharting, and thought and planning tools. Your key takeaways are: Conceptual view of code Visual mockups and mapping help you see the big elements Flowcharting helps you to map out the individual steps and conditions Thought and planning tools Study Notes How do you visualize the steps to do something? Using visualization, we’re able to map out the process for registering. Now convert this into something useful. Think logically. The flowchart helps you with […]
Computational Thought
Your next step is to learn about computational (or programmatic) thought. Your key takeaways are: Computers are stupid Software tells the computer explicitly how to solve a problem Computational thought is the act of expressing how to solve a problem Building software is a thinking profession Study Notes What is computation? Per Merriam-Webster the act or process of computing or calculating something a system of reckoning What is computing? Per Merriam-Webster to find out (something) by using mathematical processes Why mathematical processes? Remember: computers are comprised of circuitry and software. Methodical Logical Step-by-step expression Proof Drive to the solution What […]
Expressing Solution Steps
In this episode, you will walk through how to express the solution in steps. Remember that the computer requires the exact steps and conditions in order to execute the code. You’ll do various exercises to identify the exact steps. Expressions must be explicit, step-by-step, repeatable, and absolute. Oh, and you get to learn about algorithms too. Your key takeaways are: Software tells the calculator (computer) how to solve the problem The solution is expressed in a language The expression attributes are: Explicit Step-by-step Repeatable Absolute An algorithm is a recipe. It is a set of explicit, step-by-step, repeatable, and absolute […]
Lab Introduction
Let’s introduce this lab to you. You are going to learn about how to express and visualize problems. Fact Computers are very fast, but they are stupid. Why? Because you have to explicitly tell them what to do. Step-by-step. Never forget this. How does a computer do complex things? Software! Computers do not think and they do not reason. That’s your job. Computers are 1s and 0s – circuitry. You have to learn how to get the computer to do what you need. Software explicitly tells the computer how to solve a problem. The computer runs the program exactly the […]
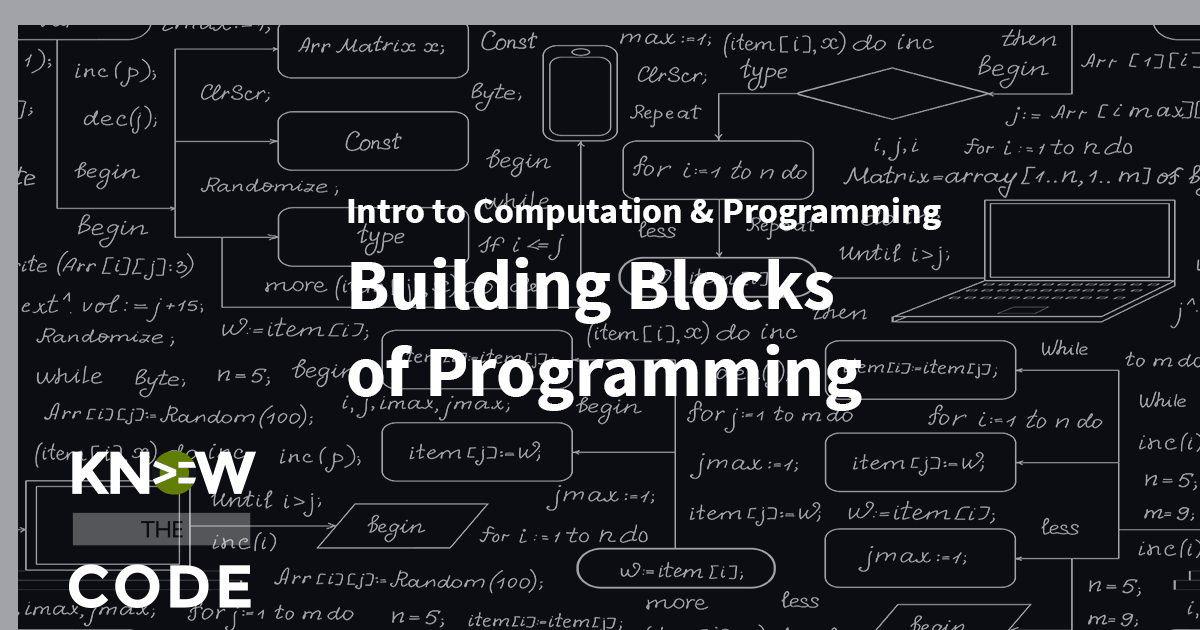
Scoping – Who Can Communicate With Whom
Let’s talk about scoping, i.e. who can communicate with whom in your program. It defines the rules to define what code can talk with other code. This is a technical discussion which includes how memory is used and allocated.
Abstraction
You need to embrace abstraction in your programming techniques. Abstraction is one of those big scary words. It removes and hides complexity, allows you to build code in a modular, purposeful fashion, and code to only what is needed. Your key takeaways are: Abstraction promotes quality code Skinny, purposeful code Promotes reuse and modularity Hides complexity Improves maintainability Study Notes What is abstraction? Purposeful methodology – everything in your code should have a specific purpose and function. Everything in that code should support only that task. Being purposeful is an earmark of quality coding. Remove or hide complexity – you only […]
Order of Execution
Software has an order of execution. This is the program sequence, meaning the order in which your lines of code will be executed. Your key takeaways are: Software executes synchronously unless asynchronous features are used Execution is in order until it encounters a control statement Order of Precedence follows rules from math Study Notes There is an order or sequence to program execution. Lines of Code Execution Types of code execution: Synchronous – current line of code is executed before moving on to the next line Asynchronous – parallel processing Languages execute code in sequential order Branching and iterators can […]