Introducing you to the Introduction to Computation and Programming course. Code is code. The basic building blocks of code are the same across all languages. Let me show you “Hello World” in many different languages.
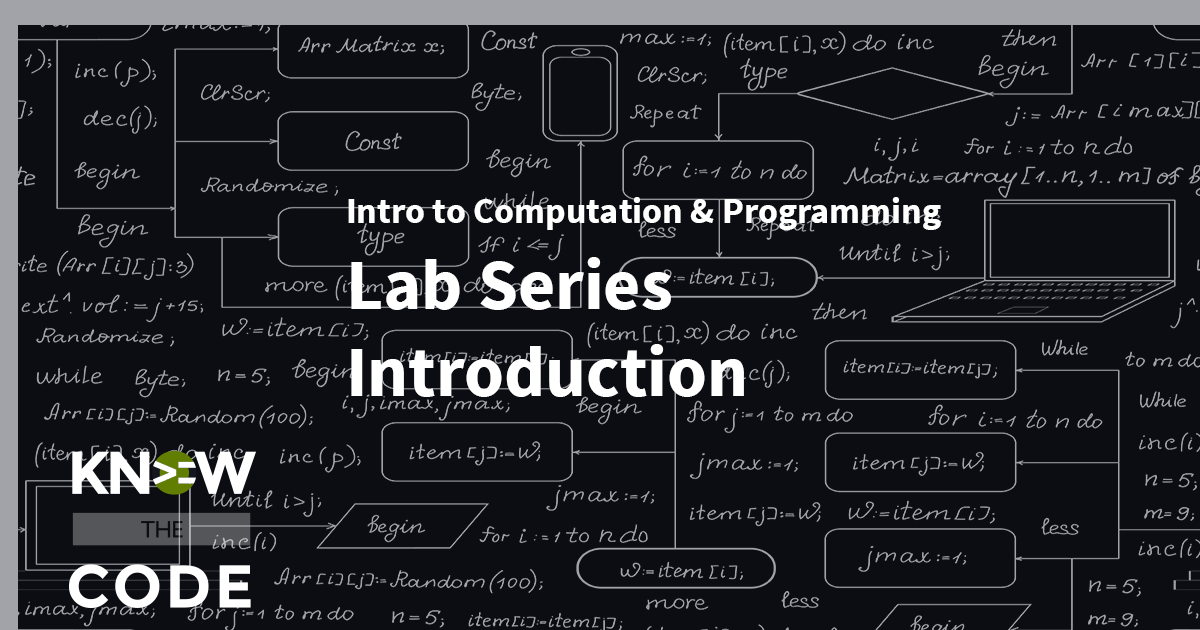
Developing & Empowering WordPress Developers
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
1 Videos 6:45 Runtime
Introducing you to the Introduction to Computation and Programming course. Code is code. The basic building blocks of code are the same across all languages. Let me show you “Hello World” in many different languages.
0 Videos Runtime
How does the computer do addition? It uses the Adder circuits which are the Half Adder and Full Adder. Your key takeaways from this episode are: Half Adder adds 2 bits holds a carry bit but, it can’t add the carry bit from the previous column Full Adder adds 2 bits plus the carry bit for a total of 3 bits capable of adding the previous column Study Notes We’ve come a long way in this lesson. Here’s a brief recap: We’ve taken a look at the different types of gate circuits. We then combined those circuits together to form […]
0 Videos Runtime
Let’s talk about how memory works. If you’re geeky, then this episode is for you. You’ll look at the S-R Latch as it handles the basics of the memory circuit. Your key takeaways in this episode are: The S-R Latch is a flip-flop circuit Uses 2 NOR gates The S-R Latch is one bit of memory Set is “true” -> stores 1 Reset is “true” -> stores 0 Study Notes We’ve been talking bits, bytes, 1s, 0s…but how does a computer actually retain memory? It retains memory one bit at a time, using an S-R Latch. Imagine we have the […]
0 Videos Runtime
The NOR Gate inverts the output of the OR Gate. Let’s see how it’s done in this episode. Your key takeaways are: NOR has an inverter after the OR Gate Opposite output state as the OR Gate If both input states are false, then the NOR output computes to true Else it’s false Study Notes What makes the output of an OR Gate true? Since it runs in parallel, if one or the other state is true, then the output is true. So, for a NOR Gate, if both states are false, then do something. Let’s compare the two: OR […]
0 Videos Runtime
The NAND Gate is a combination of the NOT and AND gates. First, it processes the AND portion. Then it inverts it. Your key takeaways from this episode are: NAND has an inverter after the AND Gate Opposite output state as the AND Gate If both input states are true, then the NAND output computes to false Else it’s true Study Notes An NAND Gate is a combination of the following gates: one AND Gate one NOT Gate The NAND Gate is simply an AND Gate with an inverter on the output. Therefore, whatever the output from the AND Gate […]
0 Videos Runtime
The XOR in software can be confusing. Let’s look at it in circuitry to understand how it works. Your key takeaways from this episode are: Study Notes An XOR Gate is a combination of the following gates: two NOT Gates two AND Gates one OR Gate Each input is wired to one leg of each AND with one of those legs going through a NOT Gate first before the AND. The NOT leg provides an inverse state of the input’s state. Each AND Gate output wires to one of the inputs into the OR Gate. Therefore, either AND Gate can […]
0 Videos Runtime
In software, the OR operator in a conditional expression is true when either decision is true. In circuitry, it works the same. When either input is on, then the OR gate turns on. Your key takeaways are: OR works the same in circuitry as it does in code OR (shown as || in code) works in “parallel” If both input states are “false,” then the circuit computes to “false” Else, the circuit computes to “true” Study Notes An OR Gate consists of inputs wired in parallel, meaning that the output will be “on” when one or both of the inputs […]
0 Videos Runtime
Just like in software, the AND gate turns on the output when both inputs are in an on state. Your key takeaways are: AND works the same in circuitry as it does in code AND Gate (or && in code) work in “series” Both input states must be “true” in order for the circuit to compute as “true” Study Notes AND Gates are inputs wired in series, meaning that both inputs must be in an “on” state for the output to be “on.” Logically you can use pseudocode to visualize the gate’s process: if ( this AND that ) then […]
0 Videos Runtime
The NOT gate in both circuitry and software represent an inverse or opposite state. It’s an inverter, meaning it flips the state and returns the opposite of it. Your key takeaways for this episode are: NOT works the same in circuitry as it does in code The NOT gate (or the ! in your code) gives you the opposite-or inverse-state Study Notes NOT = Inverse (Opposite state) What is the opposite state of the following?: On (off) Ok (not ok) True (false) 1 (0) Go (stop) Inverse Relay Logic In inverse relay logic, when the relay is off, the natural […]
0 Videos Runtime
Pseudocode is a technique for expressing code in a more natural language. It allows you to think through your code before writing it. Then the truth tables provide you with the switch (gate) states. You will use these states in your code too. Your takeaways from this episode are: Pseudocode is a verbose human-readable expression of logic Truth table provides the expected state of the output for the given argument(s) Both tools build logical and analytical thought processes Both tools help you to visualize for problem solving Study Notes Pseudocode What is pseudocode? Written way to express logic Verbose Human-readable […]
Know the Code flies on WP Engine. Check out the managed hosting solutions from WP Engine.
WordPress® and its related trademarks are registered trademarks of the WordPress Foundation. The Genesis framework and its related trademarks are registered trademarks of StudioPress.
This website is not affiliated with or sponsored by Automattic, Inc., the WordPress Foundation, or the WordPress® Open Source Project.