Let’s put iteration into practice. In this episode, you will learn about the different iterator constructs in each language. Then you’ll see them in action. Your key takeaways are: for, each, foreach instructions provide iterations through arrays, collections, or objects while, do/while, until instructions provide looping until the conditional expression is false Study Notes Types of Iterators There are several types of iterators: for loop foreach do/while while each Iterators for each language: Language for foreach, each do/while, while, until PHP Yes Yes Yes JavaScript Yes Yes Yes Ruby Yes Yes Yes Python Yes Yes C Yes Yes C# Yes […]
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
Basics of Iteration
Let’s talk about the basics of iteration. In this episode, you will learn about iteration as it is a key process in programming. Your key takeaways are: Iteration is repeating a sequence It’s a loop Separate the iteration functionality from the container Study Notes Here’s the primary question: How do you iterate through a container? How do you “say or do a thing again or again and again” (Merriam-Webster.) What is Iteration? Per Merriam-Webster: the action or a process of iterating or repeating: as a procedure in which repetition of a sequence of operations yields results successively closer to a […]
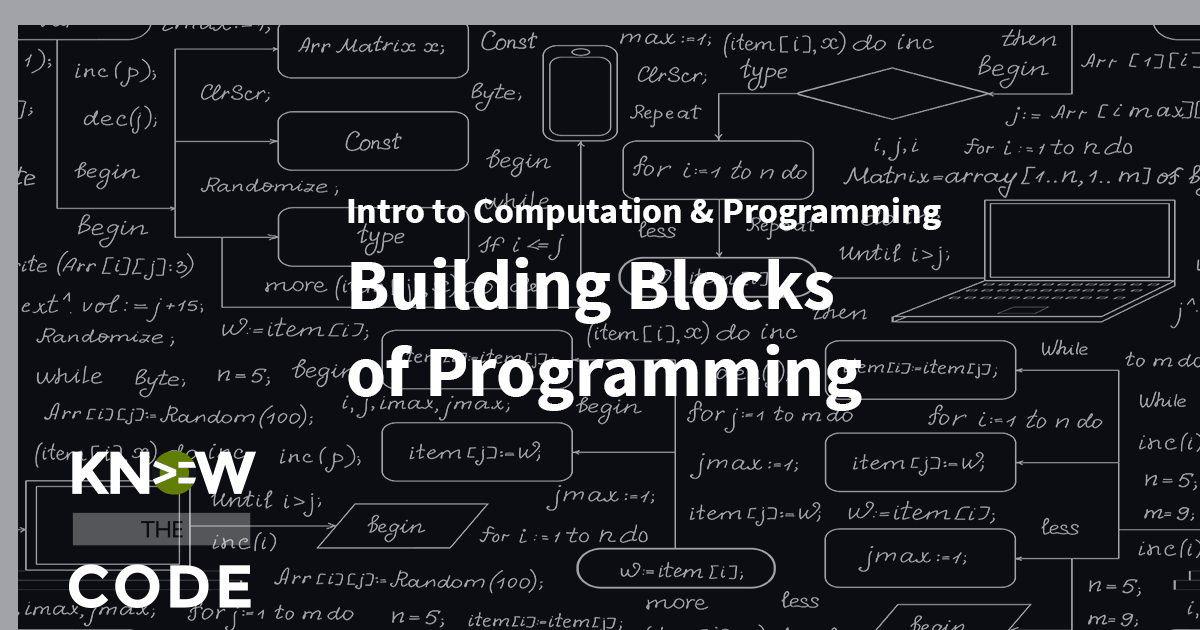
Decision Branching – switch/case
There are times when you need to make multiple decisions. In these cases, the level of branches grows. Programming languages give you an alternative with the switch/case construct. Your key takeaways are: Switch/case more concise representation of an if/else if/else conditional control statement Don’t have to repeat the conditional comparison in each branch Easier to read Gives you control to determine what code to run (execute) Study Notes Below is some pseudocode to show a multistate decision tree: Convert the multi-state decision tree into a less verbose format with a switch/case statement: Anatomy of the Switch Statement Switch Statements in […]
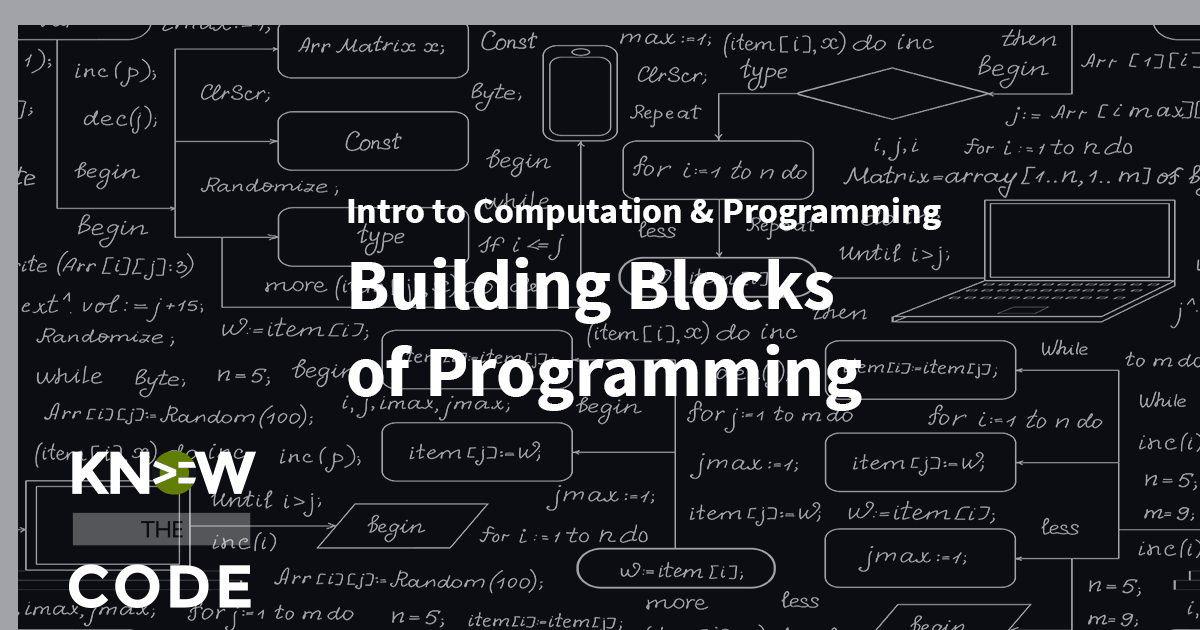
Decision Branching – if/then/else
Let’s talk about decision branching and more specifically about the “if/then/else” constructs. Decisions control the flow of your program. The branch only runs when the conditional control statements is true.
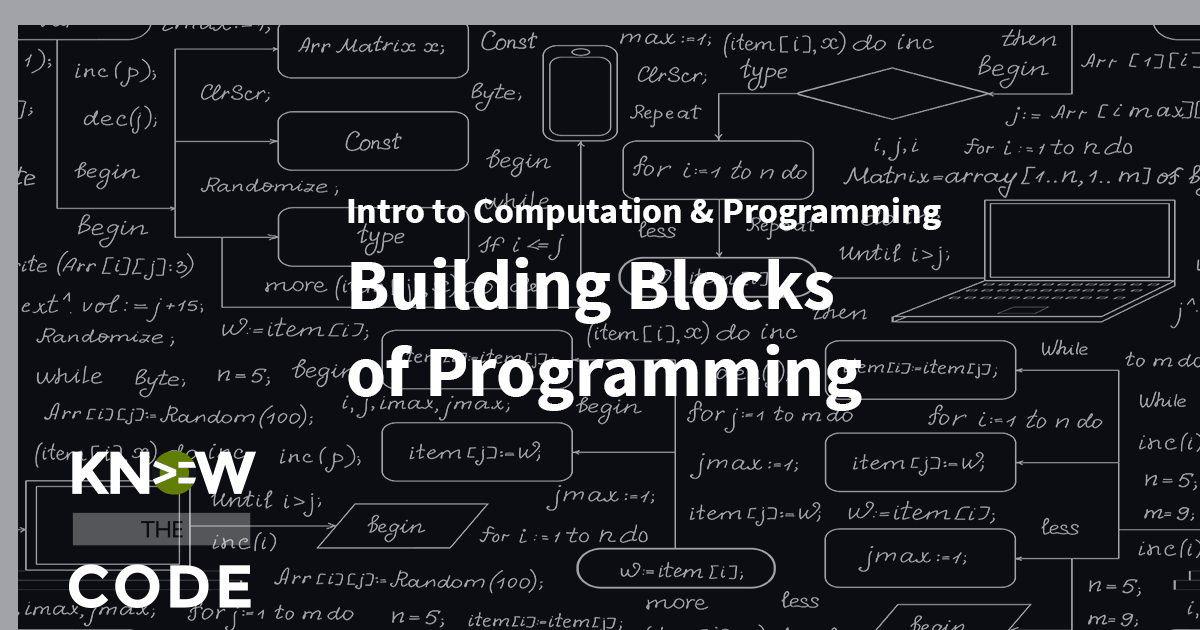
Fundamentals of Syntax
Let’s talk about the fundamentals of syntax. Language has structure and set of rules. We call this syntax. You’ll learn what it is and why language needs it.
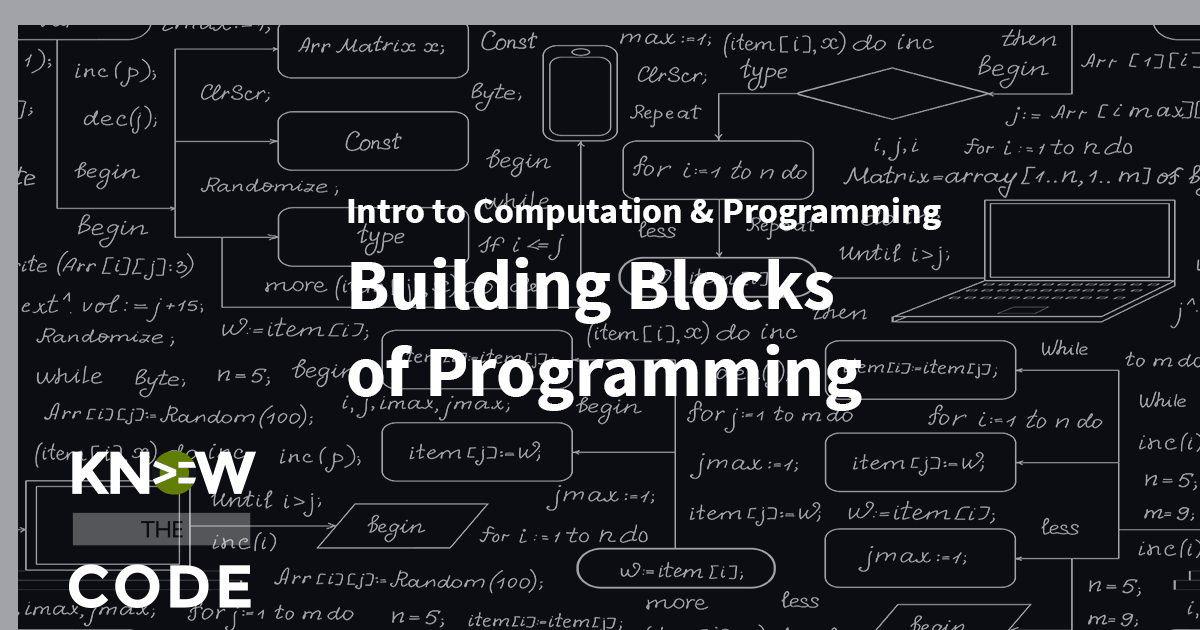
User-Friendly Expression of Information
In this episode, you will learn about user-friendly expression of information. What does that mean? Information is data. This episode talks about how data is represented in software, including variables, memory, and more.
Lab Introduction
Let’s introduce you to this lab. Are you ready to start learning about programming? Your key takeaways are: Giving you the recipes Building blocks of all programming languages You will be on your way to becoming a chef Recap Computer circuitry represents all numbers in patterns of 1s and 0s known as binary stores a bit (single 1 or 0) in its circuitry adds binary numbers How to visualize solutions How to formulate those visuals into a mock code format known as pseudocode
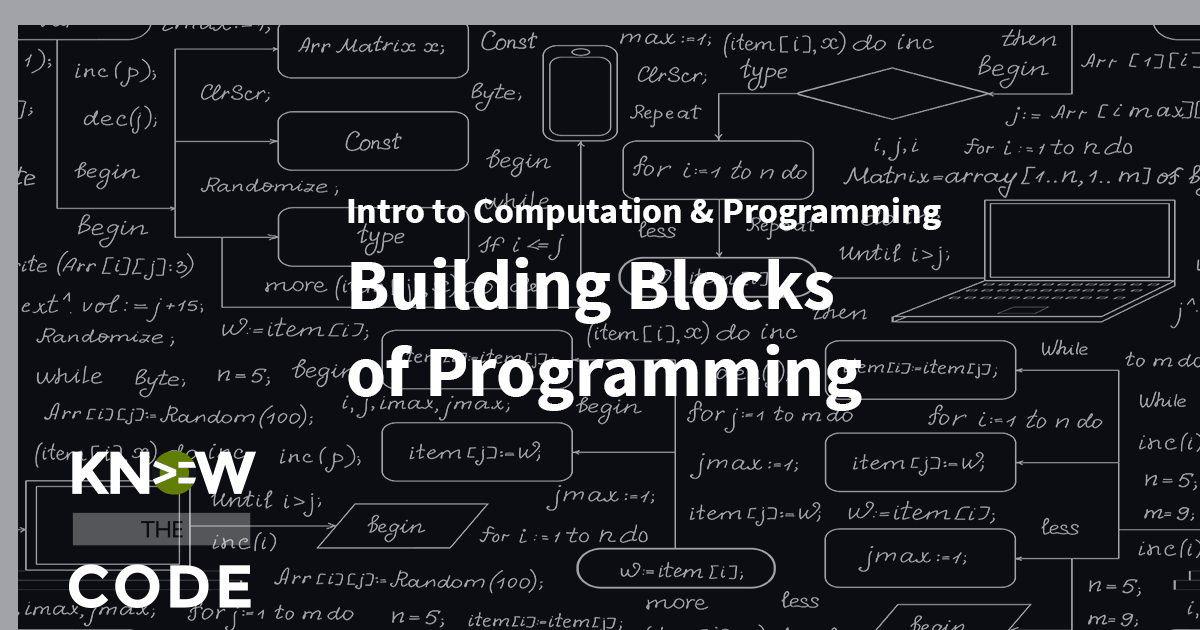
Building Blocks of Programming
All languages use the same building blocks. All of them. Period. In this lab, you will learn these building blocks. You learn about syntax, branding, iteration, order of execution, decisions, abstraction, and scoping.
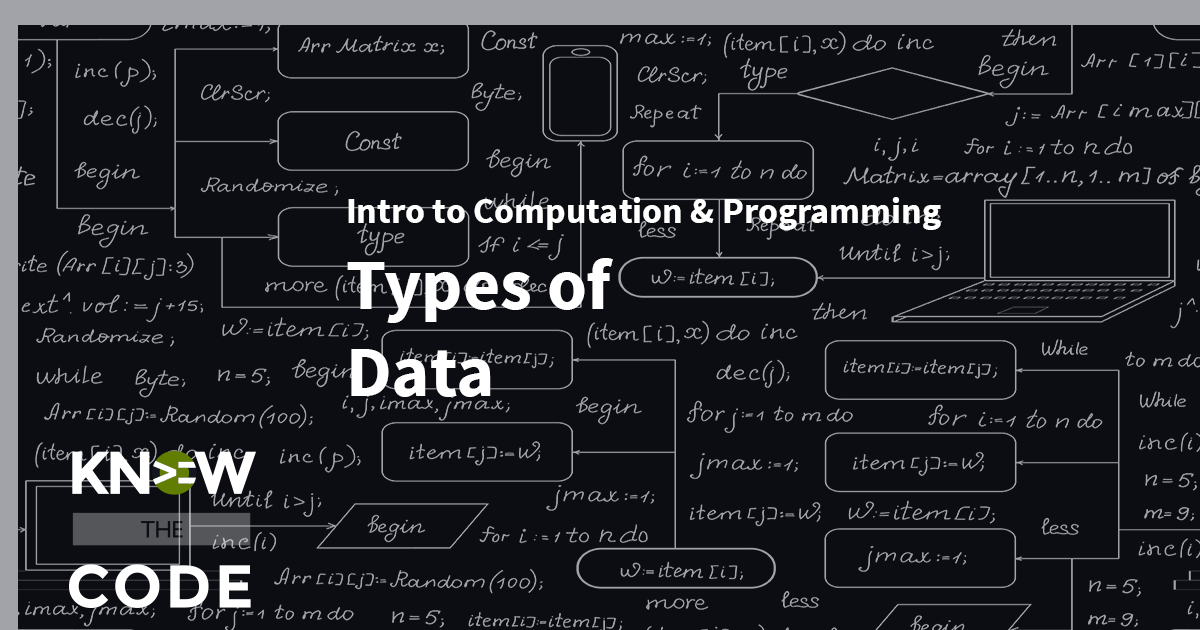
Types of Data
Data is a big part of what you do, no matter the programming language. In this lesson, we’ll talk about the different types of data type classifications including: primitives, composition, special, and abstract data types.
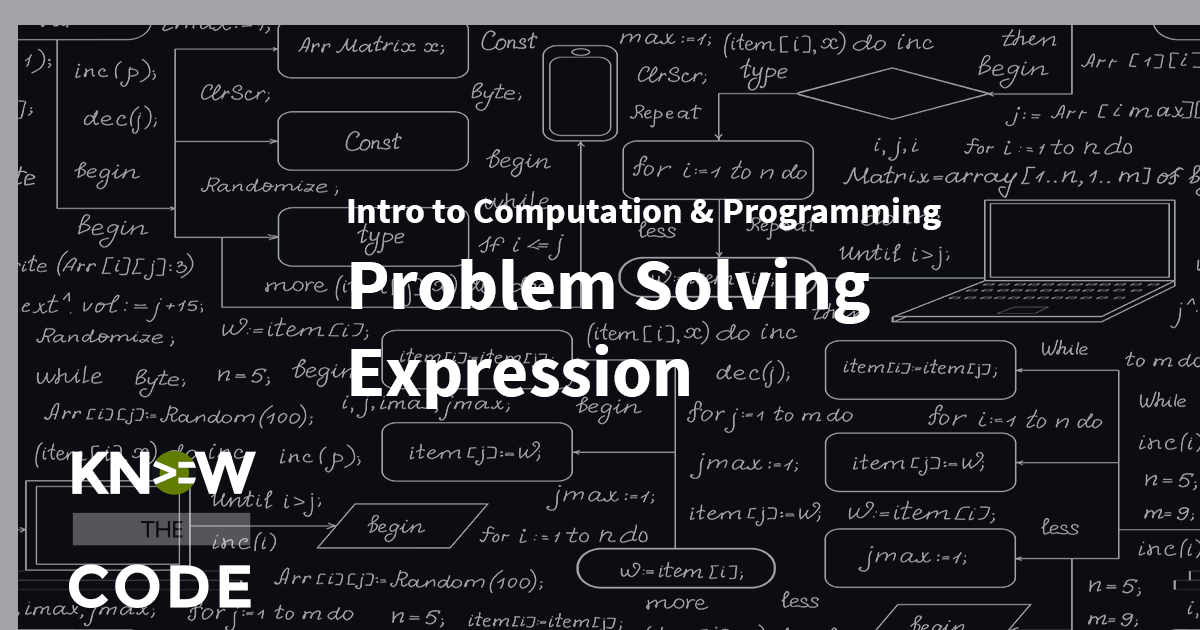
Problem Solving Expression
As a software professional, your job is to solve problems through web technologies. You are a problem solver. In this lab, you will learn about the problem expression solution steps, computational thought, computational visualization techniques, and pseudocode. This lab is about how you express and capture the problem and its solution.