Let’s build the centralized data, state, and configuration parameters store in an Object Oriented (OOP) architecture. What You Will Learn X Prerequisites See the list of prerequisites and suggestions on the series landing page.
Labs
Labs are hands-on coding projects that you build along with Tonya as she explains the code, concepts, and thought processes behind it. You can use the labs to further your code knowledge or to use right in your projects. Each lab ties into the Docx to ensure you have the information you need.
Each lab is designed to further your understanding and mastery of code. You learn more about how to think about its construction, quality, maintainability, programmatic and logical thought, and problem-solving. While you may be building a specific thing, Tonya presents the why of it to make it adaptable far beyond that specific implementation, thereby giving you the means to make it your own, in any context.
Lab Introduction
Centralized Data, State, & Config Store – Built in OOP
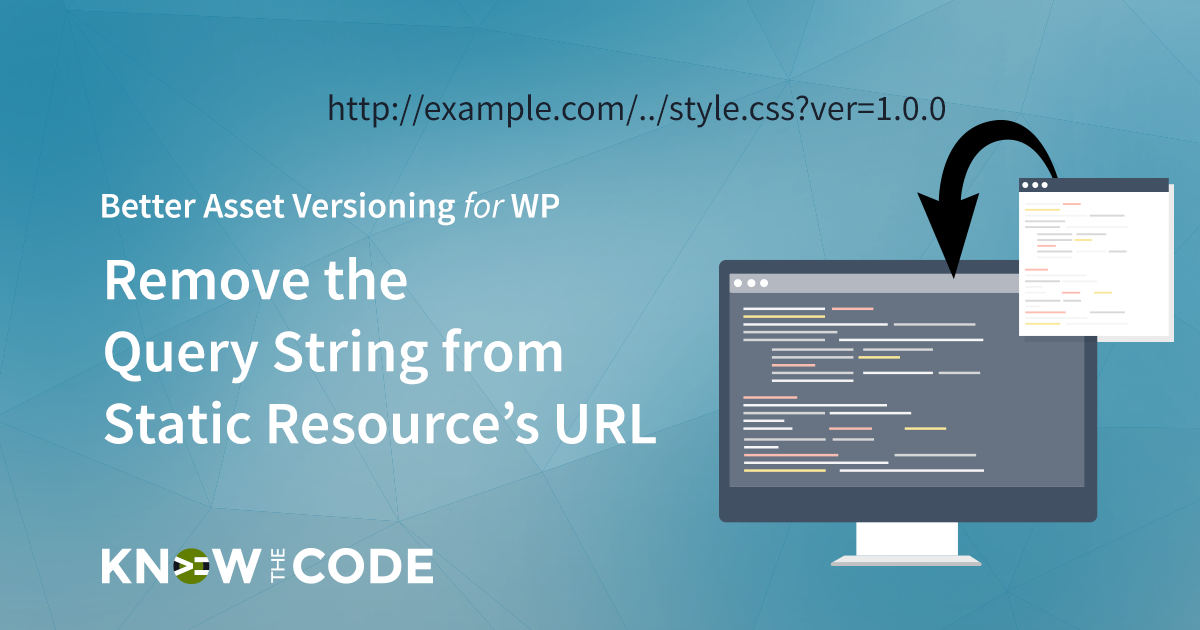
Hook into WordPress
We need to hook into a filter event within WordPress to change the stylesheets’ and scripts’ URL. How do we find this hook? Let’s talk about a strategy first. And then let’s look in WordPress Core to see where it’s fired and what arguments we will get when our code runs.
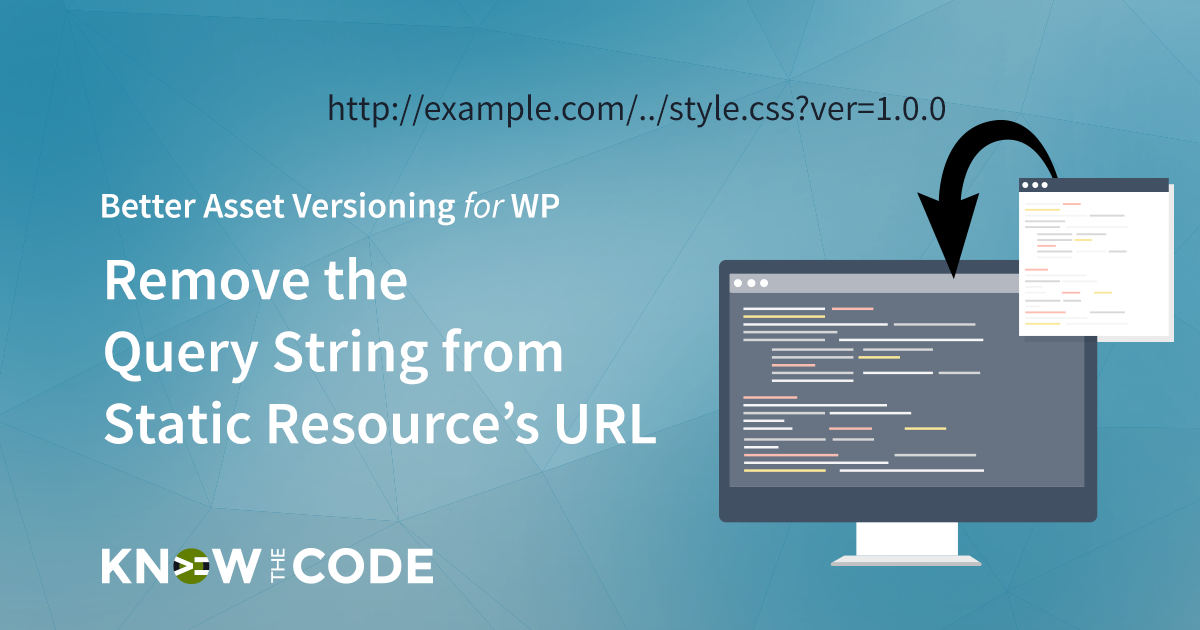
Start the URL Converter Class
Let’s start building our URL Converter class, i.e. the class that will handle checking and then converter each assets’ URL. You and I will layout the basic structure of the class including some of the starter methods and initializers. We’ll create a runtime configuration file that will get passed to the object, allowing us to change the implementation. Then we’ll add the code to the plugin’s bootstrap file to launch, load the configuration, and then create (instantiate) the URL Converter.
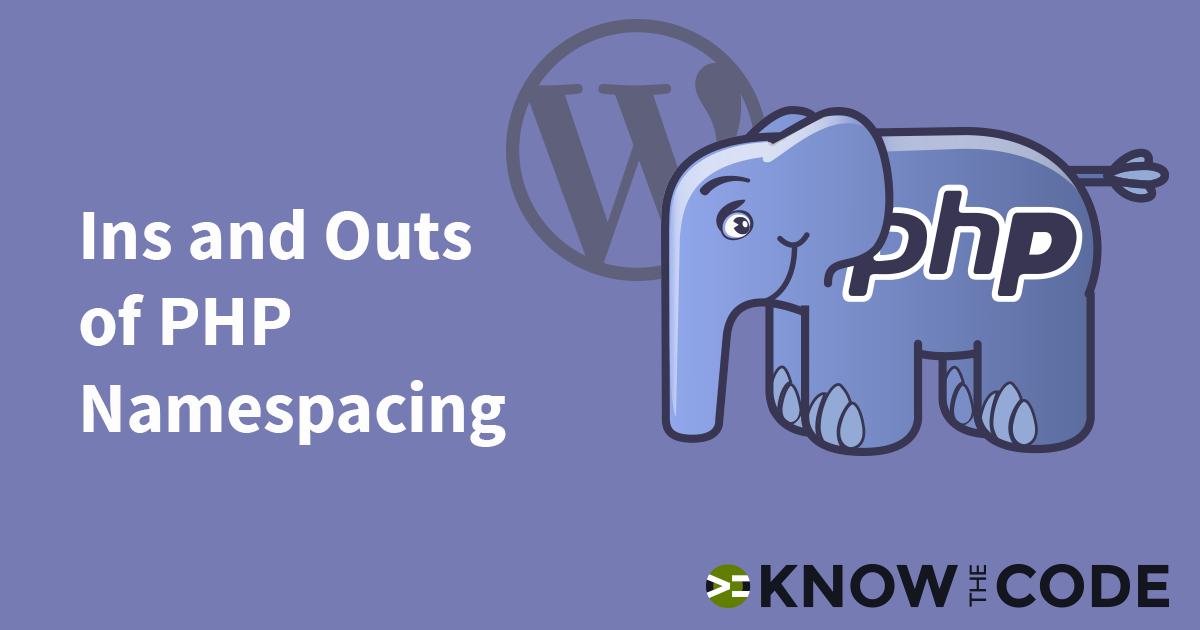
Practical Example: Convert from Prefixing
Let’s put your name skills to use. In this episode, you will convert the supplied plugin from prefixing to PHP namespacing. The best way to learn this is to start where you are, i.e. in prefixing, and walk through step-by-step the process of making a plugin namespaced. You’ll change callbacks, use the magic constant __NAMESPACE__. You’ll remove the namespacing. Step-by-step. Let’s do this together.
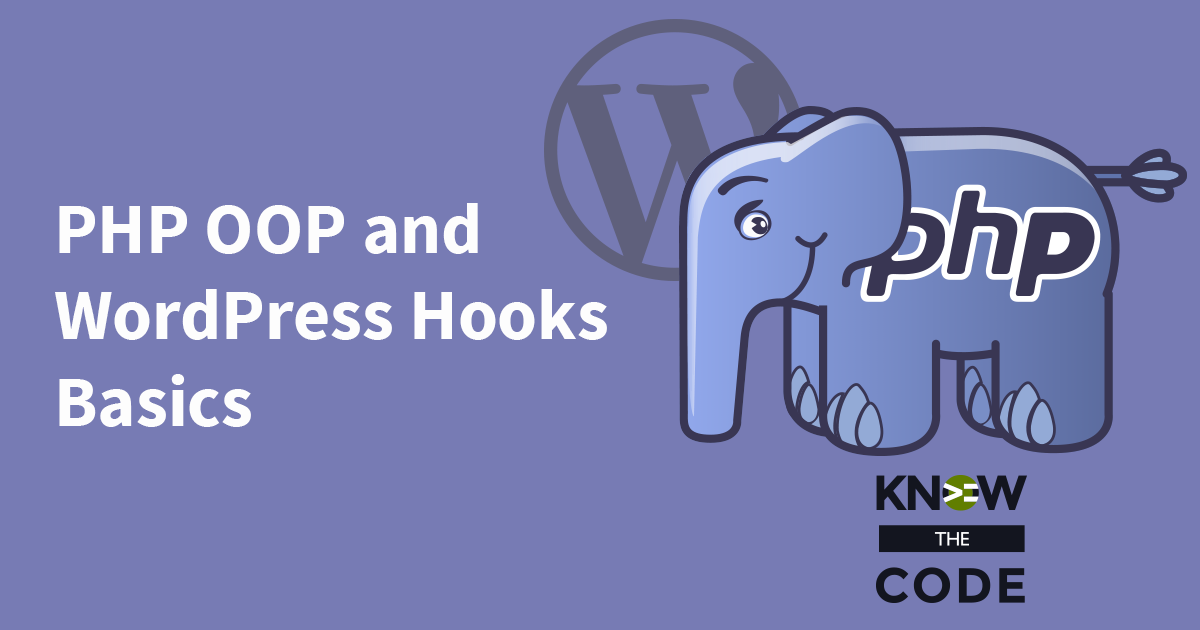
“Object Not Available” Strategies
There are times when you can’t get a hold of the object in order to remove a method callback from that object. The author wrote it such that s/he did not expose the object for you. What do you do? How can you get that object in order to do your work and unregister its callback? Here are strategies in order: Talk to the author and see s/he will add a filter or action to pass the object to you. If it’s open source, submit a pull request to add the filter/action code. Evaluate if you have to use this […]
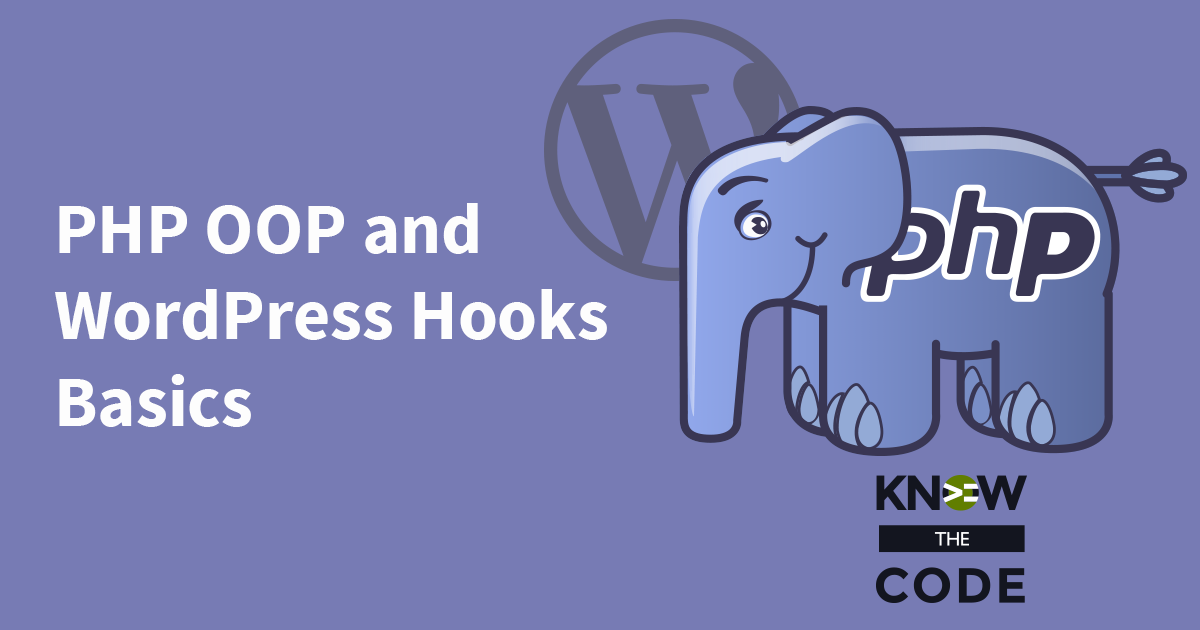
Wrap it Up
Let’s review what you learned in this lab about registering and unregistering callbacks for both static and object methods. You looked into WordPress Core and saw that it is using call_user_func_array to call each of the registered callbacks for a given event hook. You learned strategies for working with 3rd party code including WooCommerce. If the WordPress Event registrations confused you, go take this lab. Congratulations for completing this lab!
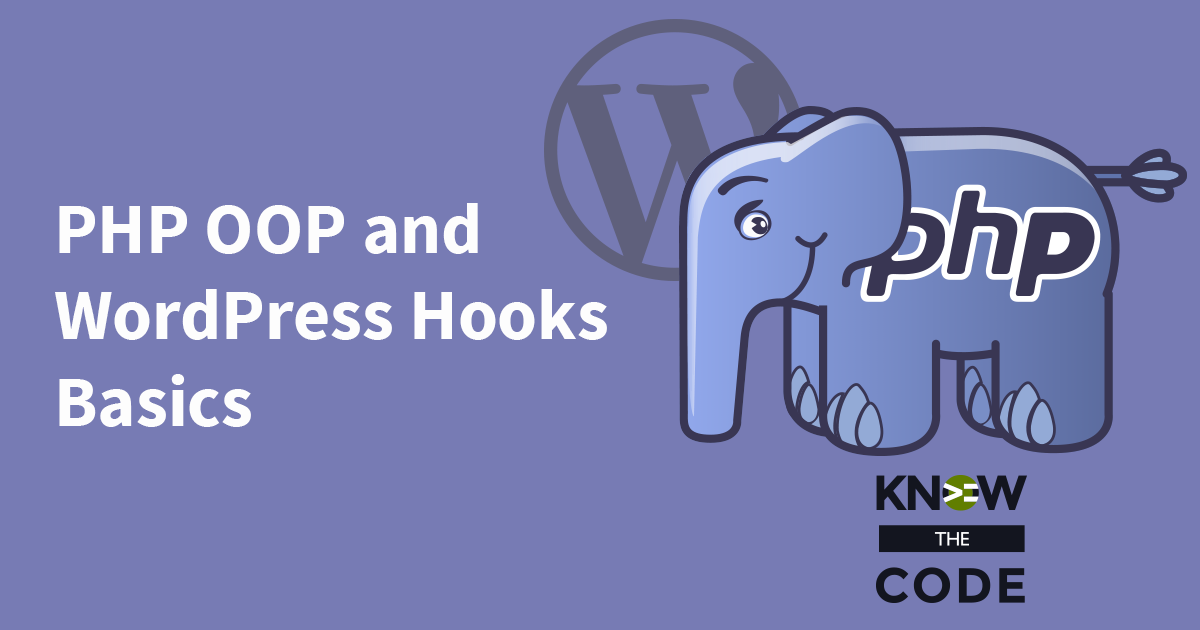
Design Strategy
When you are registering and unregistering callbacks within an object, you typically do this task as part of the object’s initialization process. Instead of putting all of the init tasks into the constructor, break it out. Put the callback registration into a separate method called init_events().
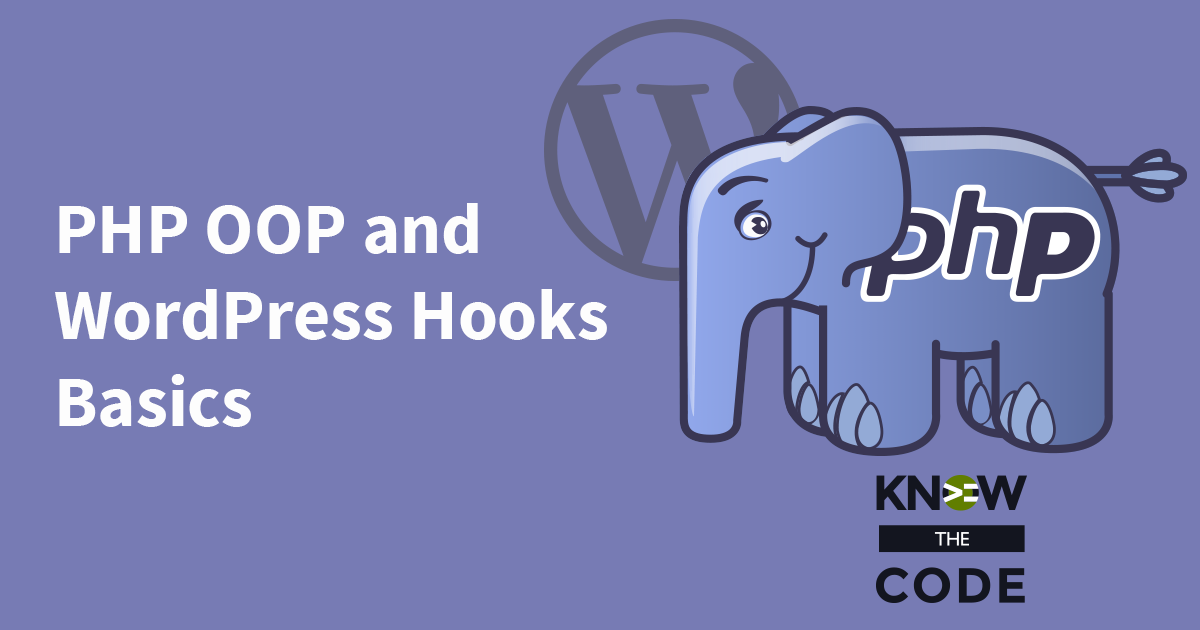
3rd Party Static Callbacks
There will be projects where you need to remove a static method’s callback from a 3rd party plugin. How do you find and remove it? You should never edit their code. Why? Because when they do updates, your changes will be overwritten. Instead, you need to find where the callback is registered, i.e. with a add_action or add_filter. Then, you need the class name. Lastly, you have to figure out the timing of when the callback is registered. Why? Because you can’t unregister it until it’s been registered. Doing it too early is a timing mistake and will cause wonky […]