In WordPress, we register callbacks to events by using add_action or add_filter. In the last episode, you learned that you must specify the fully qualified name to invoke a function (i.e. run it) outside of the namespace. This applies to add_action or add_filter. In this episode, you will learn why as well as a shorthand version using the magic constant __NAMESPACE__.
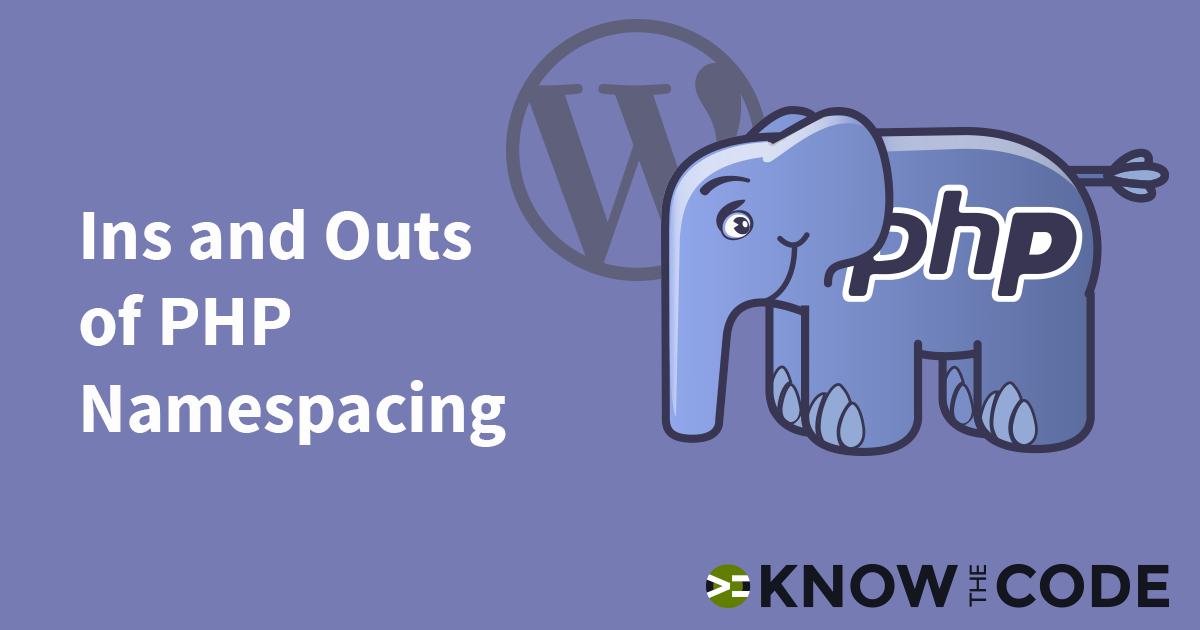